Facebook Like Drag Background Cover Changer using Jquery
Submitted by rinvizle on Wednesday, August 31, 2016 - 16:02.
In this tutorial we will show you how to create a Facebook Like Drag Background Cover Changer using Jquery by uploading, positioning and adjusting the image. And if you are looking for a application that can upload images this one is very interesting and suits for your system, and it has a combination of many features. See the example code below.
UserUpdates.php - This simple PHP class contains three functions called userDetails, userBackgroundUpdate and userBackgourndPositionUpdate.
ImageUploadAjax.php - This is for the uploading image file into uploads folder and inserting background image name into users table. And every image that the user uploads has a restricted size of 1mb.
Hope that you learn from this tutorial and don't forget to Like & Share. Enjoy Coding!
Sample Code
Index.php - This file is for displaying the background image based on the login user session id.- <!DOCTYPE html>
- <html>
- <head>
- <title>Facebook Like Drag Background Cover Changer using Jquery</title>
- <link href='timeline.css' rel='stylesheet' type='text/css'/>
- <script src="js/jquery.min.js"></script>
- <script src="js/jquery-ui.min.js"></script>
- <script src="js/jquery.wallform.js"></script>
- <script>
- $(document).ready(function()
- {
- $('body').on('change','#bgphotoimg', function()
- {
- $("#bgimageform").ajaxForm({target: '#timelineBackground',
- beforeSubmit:function(){},
- success:function(){
- $("#timelineShade").hide();
- $("#bgimageform").hide();
- },
- error:function(){
- } }).submit();
- });
- $("body").on('mouseover','.headerimage',function ()
- {
- var y1 = $('#timelineBackground').height();
- var y2 = $('.headerimage').height();
- $(this).draggable({
- scroll: false,
- axis: "y",
- drag: function(event, ui) {
- if(ui.position.top >= 0)
- {
- ui.position.top = 0;
- }
- else if(ui.position.top <= y1 - y2)
- {
- ui.position.top = y1 - y2;
- }
- },
- stop: function(event, ui)
- {
- }
- });
- });
- $("body").on('click','.bgSave',function ()
- {
- var id = $(this).attr("id");
- var p = $("#timelineBGload").attr("style");
- var Y =p.split("top:");
- var Z=Y[1].split(";");
- var dataString ='position='+Z[0];
- $.ajax({
- type: "POST",
- url: "image_saveBG_ajax.php",
- data: dataString,
- cache: false,
- beforeSend: function(){ },
- success: function(html)
- {
- if(html)
- {
- $(".bgImage").fadeOut('slow');
- $(".bgSave").fadeOut('slow');
- $("#timelineShade").fadeIn("slow");
- $("#timelineBGload").removeClass("headerimage");
- $("#timelineBGload").css({'margin-top':html});
- return false;
- }
- }
- });
- return false;
- });
- });
- </script>
- </head>
- <body>
- <ul>
- <li style="margin-left: 165px;"><a href="#home">Facebook</a></li>
- <li style="margin-left: 743px;"><a href="#profile"><img src="#">Sourcodester</a></li>
- <li><a href="#home">Home</a></li>
- <li><a href="#logout">Logout</a></li>
- </ul>
- <div id="container">
- <div id="timelineContainer">
- <div id="timelineBackground">
- <img src="<?php echo $path.$profile_background; ?>" class="bgImage" style="margin-top: <?php echo $profile_background_position; ?>;">
- </div>
- <div style="background:url(images/timeline_shade.png);" id="timelineShade">
- <form id="bgimageform" method="post" enctype="multipart/form-data" action="image_upload_ajax.php">
- <div class="updateInfo timelineInfo">
- <input type="button" class="updateInfo" value="Update Info" alt="Update Info">
- </div>
- <div class="uploadFile timelineUploadBG">
- <input type="file" name="photoimg" id="bgphotoimg" class=" custom-file-input" original-title="Change Cover Picture">
- </div>
- </form>
- </div>
- <div id="timelineProfilePic"><img src="images/profilepic1.jpg" style="width: 170px;height: 170px;"></div>
- <div id="timelineTitle"><?php echo $name; ?></div>
- <div id="timelineNav">
- <div class="buttonside">
- <input type="button" class="button" value="Timeline">
- <input type="button" class="button button2" value="About">
- <input type="button" class="button button3" value="Friends">
- <input type="button" class="button button4" value="Photos">
- <input type="button" class="button button5" value="More">
- </div>
- </div>
- </div>
- </div>
- </body>
- </html>
- <?php
- class userUpdates
- {
- private $db;
- public function __construct($db)
- {
- $this->db = $db;
- }
- public function userDetails($user_id)
- {
- $query=mysqli_query($this->db,"SELECT username,email,name,profile_background,profile_background_position FROM users WHERE user_id='$user_id' ")or die(mysqli_error($this->db));
- return $data;
- }
- public function userBackgroundUpdate($user_id,$actual_image_name)
- {
- $query=mysqli_query($this->db,"UPDATE users SET profile_background='$actual_image_name' WHERE user_id='$user_id'")or die(mysqli_error($this->db));
- return $query;
- }
- public function userBackgroundPositionUpdate($user_id,$position)
- {
- $query=mysqli_query($this->db,"UPDATE users SET profile_background_position='$position' WHERE user_id='$user_id'")or die(mysqli_error($this->db));
- return $query;
- }
- }
- ?>
- <?php
- include 'db.php';
- $session_uid='1';
- include 'userUpdates.php';
- $userUpdates = new userUpdates($db);
- include_once 'getExtension.php';
- {
- $name = $_FILES['photoimg']['name'];
- $size = $_FILES['photoimg']['size'];
- {
- $ext = getExtension($name);
- {
- if($size<(1024*1024))
- {
- $tmp = $_FILES['photoimg']['tmp_name'];
- $bgSave='<div id="uX'.$session_uid.'" class="bgSave wallbutton blackButton">Save Cover</div>';
- {
- $data=$userUpdates->userBackgroundUpdate($session_uid,$actual_image_name);
- if($data)
- echo $bgSave.'<img src="'.$path.$actual_image_name.'" id="timelineBGload" class="headerimage ui-corner-all" style="top:0px"/>';
- }
- else
- {
- echo "Fail upload folder with read access.";
- }
- }
- else
- echo "Image file size max 1 MB";
- }
- else
- echo "Invalid file format.";
- }
- else
- echo "Please select image..!";
- exit;
- }
- ?>
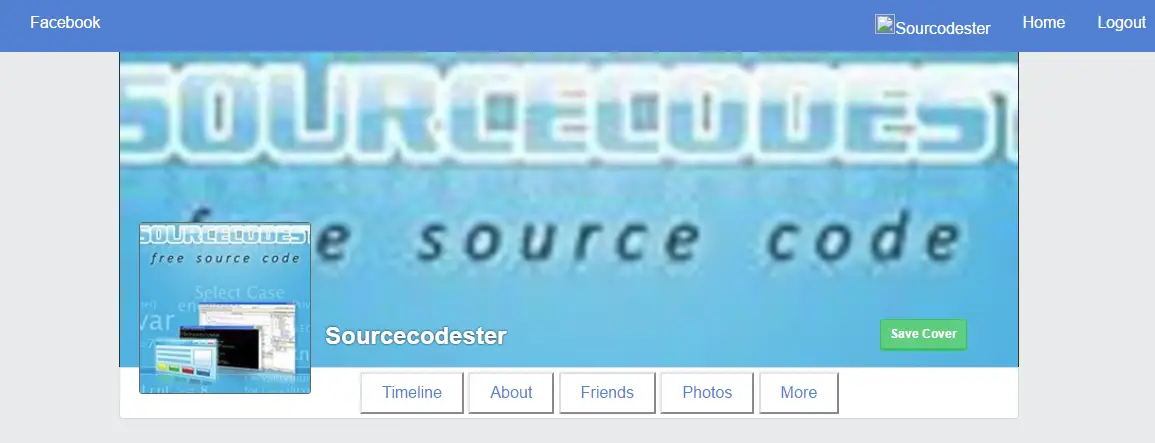