How to Create a Line Border in Java
Submitted by donbermoy on Monday, December 8, 2014 - 18:12.
This tutorial will teach you how to create a line border in java. A line border has only one line with a single color and has its own thickness for its border.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of lineBorders.java.
2. Import the following packages:
3. We will initialize variables in our Main, variable frame as JFrame and button1 labeled "1 pixel",button2 labeled "10 pixel", and button3 labeled "Round 5 pixel" as JButton.
For the thicker border, we will add another parameter to the color which is the thickness of the line in a pixel value. We will put the 10 pixel line border for button2.
For a round line border, aside from putting color of the line and its thickness, we will have Boolean value for the rounded line. Which means if we set it to True then it will be rounded. Otherwise, it is not. We will set this border in button3.
Add all the buttons in the frame using the add method.
5. Have your Layout in Grid. Lastly, set the size, visibility, and the close operation of the frame. Have this code below:
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import java.awt.*; //used to access the GridLayout and Color class
- import javax.swing.*; //used to access the JFrame, JButton, and BorderFactory class
- import javax.swing.border.*; //used to access the Border and LineBorder subclass of the border class in swing
4. Now, we will create a 1 pixel line border for Button1. We will use the BorderFactory class of the Border class. We will color the line as a yellow color. We will also use the setBorder method of the button to have the border.
- button1.setBorder(thinBorder);
- button2.setBorder(thickBorder);
- button3.setBorder(roundedBorder);
- frame.getContentPane().add(button1);
- frame.getContentPane().add(button2);
- frame.getContentPane().add(button3);
- frame.pack();
- frame.setSize(300, 100);
- frame.setVisible(true);
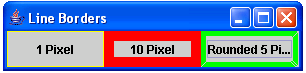
- import java.awt.*; //used to access the GridLayout and Color class
- import javax.swing.*; //used to access the JFrame, JButton, and BorderFactory class
- import javax.swing.border.*; //used to access the Border and LineBorder subclass of the border class in swing
- public class lineBorders {
- button1.setBorder(thinBorder);
- button2.setBorder(thickBorder);
- button3.setBorder(roundedBorder);
- frame.getContentPane().add(button1);
- frame.getContentPane().add(button2);
- frame.getContentPane().add(button3);
- frame.pack();
- frame.setSize(300, 100);
- frame.setVisible(true);
- }
- }