Using Google Maps in your Android program
Submitted by pavel7_7_7 on Friday, September 12, 2014 - 04:19.
Now the most part of Android devices have installed Google Maps Application by default. You can use Google Maps in your application for showing different locations.
Let's create a new Android Project. The application will consist of some buttons that will show different locations using different ways.
The first step is to create the layout of the application:
The goal of this program is not to create an application with a excellent User Interface. The main task is to test the possibilities of the using Google Maps in your program to show locations. That's way your layout should look something like this:
Now let's initialize all the buttons in the activity to be able to add click listener to them. First of all add these fields to your Activity class
After this you can initialize the buttons in the onCreate method:
The first two parameters are clear.These are the coordinates of the place what you want show. The last one is the zoom parameter - the maximal value for zoom is 23 and the minimum value is 2.
You can see that there is not always a good idea to use coordinates. Let's show the London by simply specifying the name of the city:
You can see that the format of the location string is now much more clear:
You can specify any correct street address to be shown.
Also, you can specify a search query for this format. Let's find McDonald's In New York:
Now you need some basic things how to show different locations from your application. The Google Maps can be integrated in the Android application without starting the Google Maps application. But it's another theme for another article.
- <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
- xmlns:tools="http://schemas.android.com/tools"
- android:layout_width="match_parent"
- android:layout_height="match_parent"
- tools:context="${relativePackage}.${activityClass}" >
- android:id="@+id/London"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:layout_below="@+id/Earth"
- android:layout_centerHorizontal="true"
- android:layout_marginTop="42dp"
- android:text="Show London" />
- android:id="@+id/Earth"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:layout_alignLeft="@+id/London"
- android:layout_alignParentTop="true"
- android:layout_alignRight="@+id/London"
- android:layout_marginTop="24dp"
- android:text="Show Earth" />
- android:id="@+id/mc"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:layout_centerHorizontal="true"
- android:layout_centerVertical="true"
- android:text="Show New Yourk McDonald's" />
- </RelativeLayout>
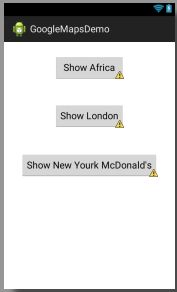
And now we can add the listeners to all of the buttons. The onClickListeners are implemented as the anonymous classes. The first example is to show the Earth continent:
Now let's take a look on the location string. It has the following format:
- geo:latitude,longitude?z=zoom
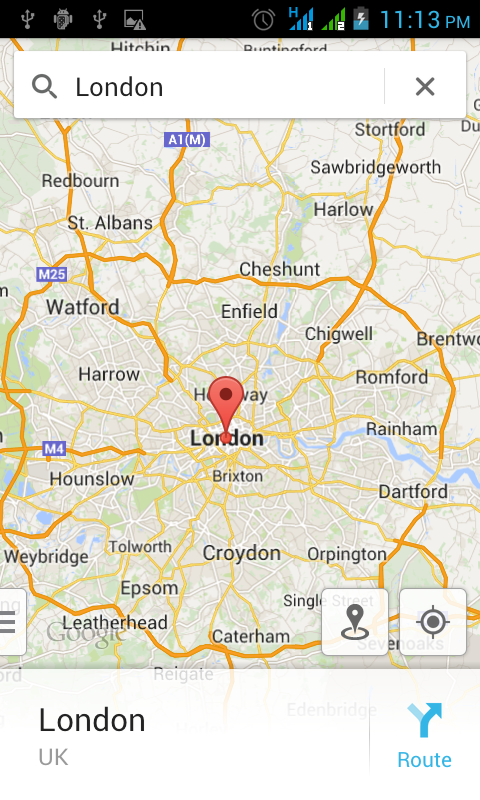
- geo:0,0?q=street+address
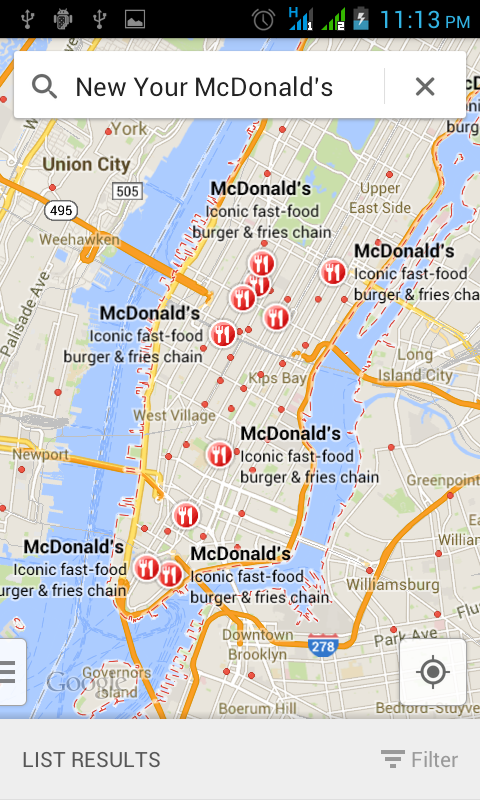