Adding Watermark to Picture using C#
Submitted by donbermoy on Friday, May 30, 2014 - 13:36.
In this tutorial, we will create a program that adds a watermark to an image/picture using C#. We all know that watermark is a faint picture or mark made in some paper during manufacture, which is visible when held against the light and typically identifies the maker, it a special design contained in electronic documents, pictures, music etc that is used to stop people from copying them.
Follow the following steps to create the program:
1. Let's start with creating a Windows Form Application in C# for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application and name your project as Add a Watermark.
2. Next, add one PictureBox named PictureBox1 and upload your desired image. Add a TextBox named TextBox1 so that the text that we have inputted from out TextBox will be displayed as our watermark in the PictureBox. Then add a Button named Button1. Design your layout like the image below:
3. Now, put this code in Button1_Click. This will trigger to put the text you have inputted in your TextBox to be displayed in your PictureBox as your Watermark.
Initialize variable font that contains a font type of Arial, font size of 36, and font style of italic.
We will use the SolidBrush class that the brush variable will be held. This SolidBrush class defines a brush of a single color and we access the argb color. Brushes are used to fill graphics shapes, such as rectangles, ellipses, pies, polygons, and paths. This class cannot be inherited.
Have this color from our RGB color of (128, 0, 0, 0).
Create a method named CreateGraphics().DrawString() of our picturebox. This will create a watermark image as it draws the string in our inputted textbox, the font we have initialized and its brush, the left location of 15, and the top location of 135.
Full source code:
Press F5 to Run the program.
Output:
Download the source code below and try it! :)
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
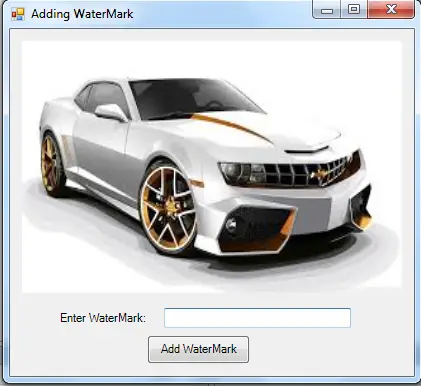
- brush.Color = Color.FromArgb(128, 0, 0, 0);
- PictureBox1.CreateGraphics().DrawString(TextBox1.Text, font, brush, 15, 135);
- using System.Diagnostics;
- using System;
- using System.Windows.Forms;
- using System.Collections;
- using System.Drawing;
- using System.Data;
- using System.Collections.Generic;
- namespace Add_a_Watemark
- {
- public partial class Form1
- {
- public Form1()
- {
- InitializeComponent();
- }
- public void Button1_Click(System.Object sender, System.EventArgs e)
- {
- brush.Color = Color.FromArgb(128, 0, 0, 0);
- PictureBox1.CreateGraphics().DrawString(TextBox1.Text, font, brush, 15, 135);
- }
- }
- }
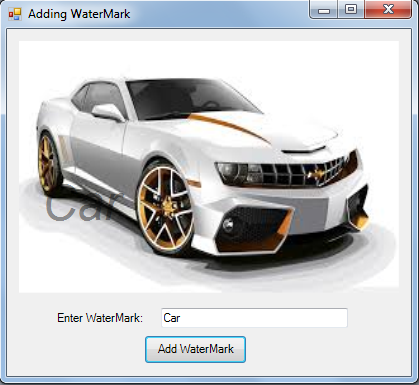