How to Login User Accounts from Microsoft Access Database using C#
Submitted by joken on Thursday, May 22, 2014 - 16:30.
This tutorial is a continuation of our last topic called “Searching a Record From Database using C#”. But this time,we will be focusing on how to create an application that will allow the user to create a login system where the user accounts stored in the database.And now we will be using the Microsoft database we created from our last tutorial and we’re going to create a new project called “userlogin”. Then design the user interface that looks like as shown below.
Next, let’s start adding functionalities to our application. First double click the form and you will be redirected to code window. Then on the “
The new added code is a .NET Framework Data Provider for OLE DB describes a collection of classes used to access an OLE DB data source in the managed space.
And under the “
At this time you can run and test the application.And as you observe, the application has a bug. The bug will occur when the user input something in the username and Password which is not found in the database. The error is look like as shown below:
To solve this bug, we’re going to modify our code. And this will now look like as shown below:
In our new modified code above, we simply first check if there’s a result found based on the user inputs. And if there’s a result, it will display the name of the user who logged in. And this will look like as shown below:
Else if there’s no result, it will show a message dialog box looks like as shown below:
Here’s all the code used in this application:
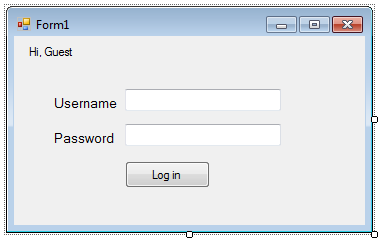
namespace userlogin
”, add this single line of code:
- using System.Data.OleDb;
public partial class Form1 : Form {
“ add the following code:
- //declare new variable named dt as New Datatable
- //this line of code used to connect to the server and locate the database (usermgt.mdb)
- static string connection = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source= " + Application.StartupPath + "/usermgt.mdb";
- <csharp>
- The code above will simply declare a new variable the following will make the connection to the server an locate our database named “usermgt.mdb”.
- Next, double click the “<strong>Login</strong>” button and add the following code:
- <csharp>
- //this query will give us the result based on user input for Username and Password
- string sql = "Select * from tbluseraccounts where userusername='"+ txtuser.Text +"' AND userpassword='"+ txtpass .Text +"'";
- da.Fill(dt);
- //then the result will get the name of the user and display it in the form using label control.
- label3.Text = dt.Rows[0][2].ToString();
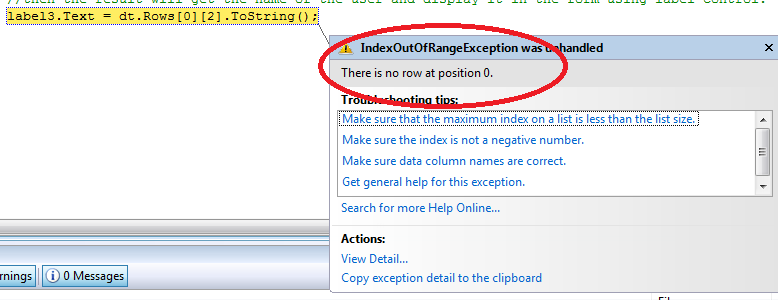
- //this query will give us the result based on user input for Username and Password
- string sql = "Select * from tbluseraccounts where userusername='"+ txtuser.Text +"' AND userpassword='"+ txtpass .Text +"'";
- da.Fill(dt);
- if (dt.Rows.Count > 0)
- {
- //then the result will get the name of the user and display it in the form using label control.
- label3.Text = dt.Rows[0][2].ToString();
- }
- else
- {
- MessageBox.Show("Username or Password Not registered!Please Contact administrator!");
- }
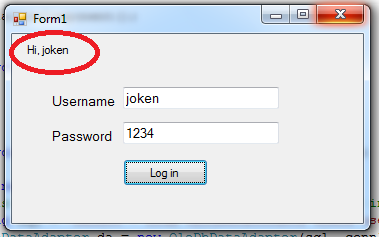
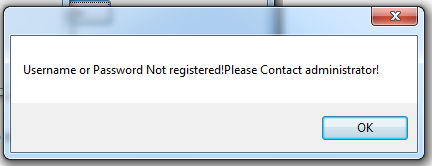
- using System;
- using System.Collections.Generic;
- using System.ComponentModel;
- using System.Data;
- using System.Drawing;
- using System.Linq;
- using System.Text;
- using System.Windows.Forms;
- using System.Data.OleDb;
- namespace userlogin
- {
- public partial class Form1 : Form
- {
- //declare new variable named dt as New Datatable
- //this line of code used to connect to the server and locate the database (usermgt.mdb)
- static string connection = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source= " + Application.StartupPath + "/usermgt.mdb";
- public Form1()
- {
- InitializeComponent();
- }
- private void Form1_Load(object sender, EventArgs e)
- {
- }
- private void btnlogin_Click(object sender, EventArgs e)
- {
- //this query will give us the result based on user input for Username and Password
- string sql = "Select * from tbluseraccounts where userusername='"+ txtuser.Text +"' AND userpassword='"+ txtpass .Text +"'";
- da.Fill(dt);
- if (dt.Rows.Count > 0)
- {
- //then the result will get the name of the user and display it in the form using label control.
- label3.Text = "Hi, " + dt.Rows[0][2].ToString();
- }
- else
- {
- MessageBox.Show("Username or Password Not registered!Please Contact administrator!");
- }
- }
- }
- }
Add new comment
- 680 views