Image Converter Using Visual Basic.Net
Submitted by joken on Friday, September 6, 2013 - 14:41.
This tutorial will teach you on how to create a simple Image Converter using Visual Basic. This program is able to change the image format like converting JPEG format to PNG format.
To build this program open Visual Basic and create a new project and name it as Image Converter. Then add two buttons, one OpenFileDialog, one SaveFileDialog, one Combobox, one Label and one Picturebox.
After setting the user interface, add the following code to “Browse Image” button.
To do this double click this button and add the code below.
And then we are now going to add the code for “Convert” Button. The code is:
Now lets try to run our program. When the program is running you can click the “Browse Image” button then select the specific image then as expected the image selected will display on the picturebox then click the “Convert Now” button and then the savefiledialog will show and write the image you desire and finally click “Save” button. After this step you can now check if the image is successfully saved to a new format.
That’s it for now folks. See you in my next tutorial thanks for reading.
Object Property Settings Button1 Name btnbrowseimg Text Browse Image Button2 Name btnConvert Text Convert Now OpenFileDialog1 Name OFDialog SaveFileDialog1 saveDialog Combobox1 Name cbformat Items PNG BMP GIF JPEG Label1 Text Image Converter Picturebox1 Name PicBox Form1 Name imgfrm Text Image Converter StartupPosition CenterScreen Show Icon FalseThe User interface looks like as shown below.
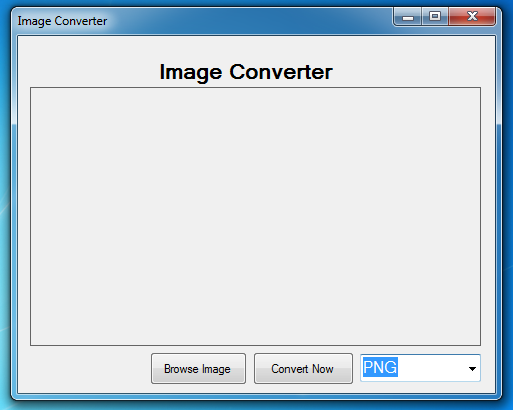
- dim val as boolean
- Private Sub btnbrowse_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnbrowse.Click
- Try
- ' when the Browse Image button is click it will open the OpenfileDialog
- 'this line of will check if the dialogresult selected is cancel then
- If OFDialog.ShowDialog <> Windows.Forms.DialogResult.Cancel Then
- 'the the selected image speciied by the user will be put into out picturebox
- picbox.Image = Image.FromFile(OFDialog.FileName)
- 'then the image sizemode is set to strectImage
- picbox.SizeMode = PictureBoxSizeMode.StretchImage
- 'then we assign val in to true because val variable above is declare as boolean
- val = True
- End If
- Catch ex As Exception
- End Try
- End Sub
- Private Sub btnconvert_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnconvert.Click
- Try
- 'it check if the variable val is set to val
- If val = True Then
- 'check if what format is selected by user in the combobox
- 'the after this the program will convert it to the desired format by the user.
- If cbformats.SelectedItem = "PNG" Then
- saveDialog.Filter = "PNG|*.png"
- If saveDialog.ShowDialog <> Windows.Forms.DialogResultDialogResult.Cancel Then picbox.Image.Save(saveDialog.FileName, System.Drawing.Imaging.ImageFormat.Png)
- ElseIf cbformats.SelectedItem = "JPEG" Then
- saveDialog.Filter = "JPEG|*.jpg"
- If saveDialog.ShowDialog <> Windows.Forms.DialogResult.Cancel Then picbox.Image.Save(saveDialog.FileName, System.Drawing.Imaging.ImageFormat.Jpeg)
- ElseIf cbformats.SelectedItem = "BMP" Then
- saveDialog.Filter = "BMP|*.bmp"
- If saveDialog.ShowDialog <> Windows.Forms.DialogResult.Cancel Then picbox.Image.Save(saveDialog.FileName, System.Drawing.Imaging.ImageFormat.Bmp)
- ElseIf cbformats.SelectedItem = "GIF" Then
- saveDialog.Filter = "GIF|*.gif"
- If saveDialog.ShowDialog <> Windows.Forms.DialogResult.Cancel Then picbox.Image.Save(saveDialog.FileName, System.Drawing.Imaging.ImageFormat.Gif)
- End If
- End If
- Catch ex As Exception
- End Try
- End Sub