How to Dynamically Convert File Size in JavaScript
How to Dynamically Convert File Size in JavaScript
Introduction
In this tutorial we will create a How to Dynamically Convert File Size in JavaScript. This tutorial purpose is to teach you on how to convert any file size. This will tackle all the basic function that will convert the file size you have entered. I will provide a sample program to show the actual coding of this tutorial.
This tutorial is simple and easy to understand just follow the instruction I provided and you can do it without a problem. This program can be use to any system or application if you to know the conversion value of any file size. I will give my best to provide you the easiest way of creating this program Convert File Size. So let's do the coding.
Before we get started:
This is the link for the template that i used for the layout design https://getbootstrap.com/.
Creating The Interface
This is where we will create a simple interface for our application. This code will display html form and buttons. To create this simply copy and write it into your text editor, then save it as index.html.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- </div>
- </nav>
- <div class="col-md-6 well">
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="col-md-5">
- <div class="form-group">
- <input type="number" class="form-control" id="number1"/>
- </div>
- <div class="form-inline">
- <select class="form-control" id="file1" style="width:50%;">
- </select>
- <br />
- <br />
- <select class="form-control" id="file2" style="width:50%;">
- </select>
- </div>
- <br />
- <div class="form-group">
- <input type="text" class="form-control" id="number2" disabled="disabled"/>
- </div>
- </div>
- </div>
- </div>
- </body>
- </html>
Creating JavaScript Function
This is where the main function of the application is. This code will dynamically convert your the file size when the button is clicked. To do this just copy and write these block of codes inside the text editor and save it as script.js.- function convertFileSize(){
- let number=document.getElementById('number1').value;
- if(number==""){
- alert("Please enter something");
- }else{
- let result;
- let file1=document.getElementById('file1').value;
- let file2=document.getElementById('file2').value;
- if(file1=="Byte" && file2=="Byte"){
- result=parseInt(number);
- }else if(file1=="Byte" && file2=="KB"){
- result=Math.round(parseInt(number)/1024)
- }else if(file1=="Byte" && file2=="MB"){
- result=Math.round(parseInt(number)/1024/1024);
- }else if(file1=="Byte" && file2=="GB"){
- result=Math.round(parseInt(number)/1024/1024/1024);
- }else if(file1=="KB" && file2=="Byte"){
- result=parseInt(number)*1024;
- }else if(file1=="KB" && file2=="KB"){
- result=parseInt(number);
- }else if(file1=="KB" && file2=="MB"){
- result=Math.round(parseInt(number)/1024);
- }else if(file1=="KB" && file2=="GB"){
- result=Math.round(parseInt(number)/1024/1024);
- }else if(file1=="MB" && file2=="Byte"){
- result=parseInt(number)*1024*1024;
- }else if(file1=="MB" && file2=="KB"){
- result=parseInt(number)*1024;
- }else if(file1=="MB" && file2=="MB"){
- result=parseInt(number);
- }else if(file1=="MB" && file2=="GB"){
- result=Math.round(parseInt(number)/1024);
- }else if(file1=="GB" && file2=="Byte"){
- result=parseInt(number)*1024*1024*1024;
- }else if(file1=="GB" && file2=="KB"){
- result=parseInt(number)*1024*1024;
- }else if(file1=="GB" && file2=="MB"){
- result=parseInt(number)*1024;
- }else if(file1=="GB" && file2=="GB"){
- result=parseInt(number);
- }
- document.getElementById('number2').value = result;
- }
- }
In the code above we will only create a method called convertFileSize(), this function will convert the file size you have entered to a specific value. This function contains several conditional statement that handle the conversion for the specific file size.
Output:
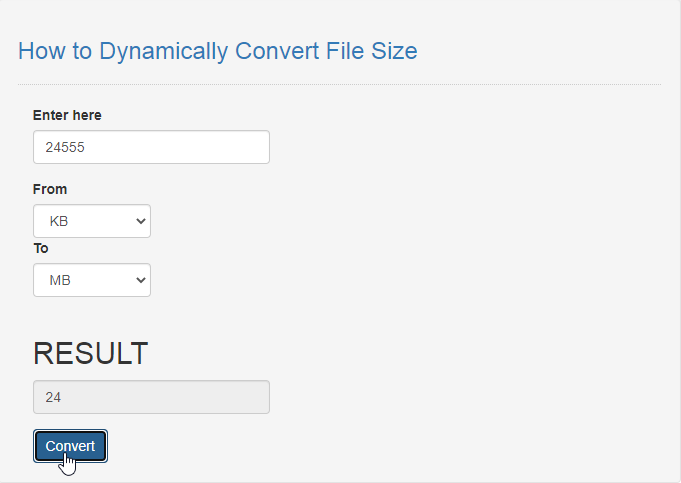
The How to Dynamically Convert File Size in JavaScript source code that I provide can be download below. Please kindly click the download button.
There you have it we successfully created How to Dynamically Convert File Size in JavaScript. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for JavaScript Language
Add new comment
- 244 views