How to Hide Password Dynamically using jQuery
How to Hide Password Dynamically using jQuery
Introduction
In this tutorial we will create a How to Hide Password Dynamically using jQuery. This tutorial purpose is to show how you can hide your password detail. This will cover all the basic function that will hide password. I will provide a sample program to show the actual coding of this tutorial.
This tutorial is simple and easy to understand just follow the instruction I provided and you can do it without a problem. This program can be use to any system if you want to hide your password from being visible. I will give my best to provide you the easiest way of creating this program Hide Password. So let's do the coding.
Before we get started:
First you have to download the jQuery plugin.
Here is the link for the jQuery that i used in this tutorial https://jquery.com/.
Lastly, this is the link for the template that i used for the layout design https://getbootstrap.com/.
Creating The Interface
This is where we will create a simple interface for our application. This code will html forms and a toggle button. To create this simply copy and write it into your text editor, then save it as index.html.- <!DOCTYPE html>
- <html>
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- </div>
- </nav>
- <div class="col-md-6 well">
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="col-md-6" style="background-color:aqua; border-radius:10px; padding:20px;">
- <div class="form-inline">
- <br />
- <input type="text" id="username" class="form-control" value="sourcecodester" readonly="readonly">
- </div>
- <br />
- <div class="form-inline">
- <br />
- </div>
- </div>
- </div>
- </body>
- </html>
Creating JavaScript Function
This is where the main function of the application is. This code will hide your password dynamically when the button is clicked. To do this just copy and write these block of codes inside the text editor and save it as script.js.- $(document).ready(function(){
- $('#toggle').on('click', function(){
- let pass=$('#password').attr('type');
- if(pass == 'password'){
- $('#password').attr('type', 'text');
- $('#btnText').text('Hide');
- $(this).removeClass('btn-primary').addClass('btn-success');
- $('#icon').removeClass('glyphicon-eye-open').addClass('glyphicon-eye-close');
- }
- else{
- $('#password').attr('type', 'password');
- $('#btnText').text('Show');
- $(this).removeClass('btn-success').addClass('btn-primary');
- $('#icon').removeClass('glyphicon-eye-close').addClass('glyphicon-eye-open');
- }
- });
- });
In the given code we first call the basic jQuery ready event to signal that the DOM of the page is now ready to be use. Next we will bind the password textbox a specific variable called pass. In order for us to hide the password we will just use the attr() function. This will toggle the password attribute by changing the textbox type format from text to password or vice versa.
Output:
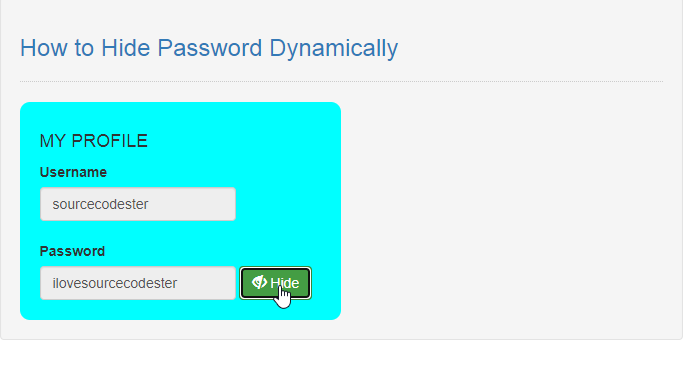
The How to Hide Password Dynamically using jQuery source code that I provide can be download below. Please kindly click the download button.
There you have it we successfully created How to Hide Password Dynamically using jQuery. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for JavaScript Language
Comments
Add new comment
- Add new comment
- 286 views