How to Create Multiplication Table in JavaScript
Introduction
In this tutorial we will create a How to Create Multiplication Table in JavaScript. This tutorial purpose is to give a simple tool that can generate a multiplication table. This will tackle the multiplying of integers. I will provide a sample program to show the actual coding of this tutorial.
This tutorial is very easy to understand just follow the instruction I provided and you will do it without a problem. This program can be use when you want to learn how to multiply numbers. I will try my best to give you the easiest way of creating this program Multiplication Table. So let's do the coding.
Before we get started:
This is the link for the template that i used for the layout design https://getbootstrap.com/.
Creating The Interface
This is where we will create a simple interface for our application. This code will display an html form that we will be needed to submit. To create this simply copy and write it into your text editor, then save it as index.html.- <!DOCTYPE html>
- <html>
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- </div>
- </nav>
- <div class="col-md-6 well">
- <hr style="border-top:1px dotted;"/>
- <div class="col-md-3">
- <div class="form-group">
- <input type="number" class="form-control" id="number"/>
- <br />
- </div>
- </div>
- <div class="col-md-9 table-responsive">
- <table class="table table-bordered">
- <thead class="alert-info">
- <tr>
- </tr>
- </thead>
- </table>
- </div>
- </div>
- </body>
- </html>
Creating JavaScript Function
This is where the main function of the application is. This code will generate a multiplication table when an event is trigger. To do this just copy and write these block of codes inside the text editor and save it as script.js.- document.getElementById("number").onkeyup=function(){
- var input=parseInt(this.value);
- if(input > 20){
- this.value = 20;
- }
- if(input < 0){
- this.value = 1;
- }
- }
- function displayTable(){
- var number = document.getElementById('number').value;
- if(number == ""){
- alert("Enter some number first");
- }else{
- var digit = 0;
- if(number <= 0){
- digit = 1;
- document.getElementById('number').value = 1;
- }else if(number > 20){
- digit = 20;
- document.getElementById('number').value = 20;
- }else{
- digit = number;
- }
- var data = "";
- for(var i = 1; i <= digit; i++) {
- data += "<tr>";
- for(var j = 1; j <= 10; j++){
- data += "<td>"+i*j+"</td>";
- }
- data += "</tr>";
- }
- document.getElementById('data').innerHTML = data;
- }
- }
In this code we first restrict the maximum value that can be submitted in the textbox by 20. We then bind the textbox value into a variable and set its name into number.
To generate the multiplication table, we increment the submitted value using nested loop with a maximum length of 10 in order to get the multiplied numbers and display it into a html content.
Output:
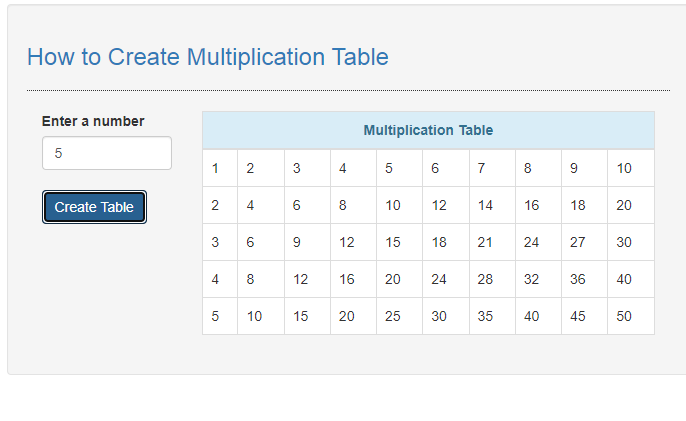
The How to Create Multiplication Table in JavaScript source code that I provide can be download below. Please kindly click the download button.
There you have it we successfully created How to Create Multiplication Table in JavaScript. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for JavaScript Language