JavaScript - Render HTML With Bootstrap Using Node.js
Submitted by razormist on Thursday, January 3, 2019 - 21:02.
Language
In this tutorial we will create a Render HTML With Bootstrap Using Node.js. The Node.js is a run-time environment includes everything you need to execute a program written in JavaScript. It is an open source, cross-platform runtime environment for developing server-side and networking applications. It is widely use by developers because it provide multiple libraries that simplifies the web development.
This will install nodemon, a tool that helps develop node.js based applications by automatically restarting the node application when file changes in the directory are detected.
There you have it we successfully created a Render HTML With Bootstrap Using Node.js. I hope that this very simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!!
Getting started
First you will need to download & install the Node.js, here's the link https://nodejs.org/en/. After Node.js is installed, open the command prompt then type "npm install -g nodemon", and hit enter.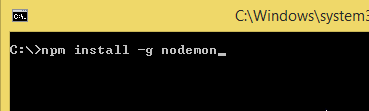
The Main Interface
This code contains the interface of the application. This code will render application and display the form. First cd to your working directory and write these block of code inside the text editor and save this as index.html.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <link type='text/css' href='css/bootstrap.css' rel='stylesheet' />
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- </div>
- </nav>
- <div class="col-md-6 well">
- <hr style="border-top:1px dotted #ccc;"/>
- </div>
- <div class="modal fade" id="form_modal" aria-hidden="true">
- <div class="modal-dialog">
- <div class="modal-content">
- <div class="modal-header">
- </div>
- <div class="modal-body">
- </div>
- <div class="modal-footer">
- </div>
- </div>
- </div>
- </div>
- </body>
- </html>
Creating the Script
This code contains the main function of the application. This code will create a server side script that let you access your website locally. To that just kindly copy and write these block of codes inside the text editor, then save it to your working directory as server.js.- var http = require('http');
- var fs = require('fs');
- var url = require('url');
- var path = require('path');
- var server = http.createServer(function(request, response) {
- var pathname = url.parse(request.url).pathname;
- var ext = path.extname(pathname);
- if(ext){
- if(ext != '.ico'){
- if(ext === '.css'){
- response.writeHead(200, {"Content-Type": "text/css"});
- }else if(ext === '.js'){
- response.writeHead(200, {"Content-Type": "text/js"});
- }
- response.write(fs.readFileSync(__dirname + pathname, 'utf8'));
- }
- }else{
- response.writeHead(200, {"Content-Type": "text/html"});
- response.write(fs.readFileSync("index.html", "utf8"));
- }
- response.end();
- }).listen(8080)
Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.