Undo Text using Ctrl Z in Java
Submitted by donbermoy on Tuesday, December 16, 2014 - 10:22.
Language
This tutorial will teach you how to create a program that can undo a text using a shortcut key of ctrl+z in java.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of undoCtrlZ.java.
2. Import the following package library:
3. We will initialize variables in our Main, variable frame as JFrame, txtArea for JTextArea, undo as UndoManager and declare it as final because we will create an inner class on it, variable doc for the Document class.
4. Create an inner class for the UndoableEditEvent of the doc in our textArea. This will trigger that the textArea can be editable.
5. Create also an inner class that will have an undo event on the textArea. This will use the getActionMap method.
To have a ctrl z shortcut for the undo. Have this code below:
6. Lastly, add the components, set the size and visibility to true, and close its operation.
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import java.awt.event.*; //used to access ActionEvent class
- import javax.swing.*; //used to access AbstractAction,JFrame, JScrollPane, JTextArea, and KeyStroke class
- import javax.swing.event.*; // used to access UndoableEditEvent and UndoableEditListener class
- import javax.swing.text.*;// used to access the Document and JTextComponent class
- import javax.swing.undo.*; // used to access CannotUndoException and UndoManager class
- undo.addEdit(evt.getEdit());
- }
- });
- try {
- if (undo.canUndo()) {
- undo.undo();
- }
- }
- }
- });
- frame.setSize(380, 320);
- frame.setLocationRelativeTo(null);
- frame.setVisible(true);
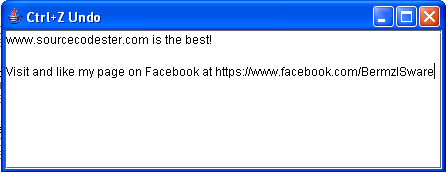
- import java.awt.event.*; //used to access ActionEvent class
- import javax.swing.*; //used to access AbstractAction,JFrame, JScrollPane, JTextArea, and KeyStroke class
- import javax.swing.event.*; // used to access UndoableEditEvent and UndoableEditListener class
- import javax.swing.text.*;// used to access the Document and JTextComponent class
- import javax.swing.undo.*; // used to access CannotUndoException and UndoManager class
- public class undoCtrlZ {
- undo.addEdit(evt.getEdit());
- }
- });
- try {
- if (undo.canUndo()) {
- undo.undo();
- }
- }
- }
- });
- frame.setSize(380, 320);
- frame.setLocationRelativeTo(null);
- frame.setVisible(true);
- }
- }
Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.
Add new comment
- 513 views