Introduction to LINQ With C#
Submitted by thusitcp on Thursday, October 16, 2014 - 16:29.
Language
Introduction to LINQ
Integrating data queries into c# has been a goal for years. Microsoft put lot of effort to such a goal over the years. At lat outcome is LINQ (Language Integrated Queries). Using LINQ we can specify what object we want without knowing the type of data source. There are two type of method usage in LINQ. Static method represents the query operators and anonymous method specifies the predicate.
What are the different types of data?
Development of software is huge challenge for development team since they have to deal with number of data sources. They need to know each of data source API within life time of the project. Using number of data sources within code will make development much harder and delay the development. Following are some of typical data type challengers
Different data type
Different data sources
Relationship with hierarchies
Limited intellisense or language
What is the motivation of LINQ?
LINQ is a new idea and unique API which provide data access easier and enable queering capabilities to more power. Generally data sources determine tools and API to use. As e.g. object data, relation data, XML data. If someone is working with these API he will need better understanding how to dealing with data and API. Greatest achievement of LINQ is, LINQ can be used to query many different types of data, including relational, XML, and even objects. Another way to describe LINQ is that it is programming language syntax that is used to query data.
In this tutorial I will guide you few example of LINQ.
I am here not going to teach you all the LINQ Technologies. Mainly focus on to explain few xml handling with LINQ. Let say we have xml file called customer.xml and it has following structure
Following c# code shows how to use LINQ in C# how to load data to combo box and data grid
How to load document from path
Few data bindings
Following code shows you how to used where in XML document
I will explain object data handling with LINQ in my next LINQ tutorial
- <?xml version="1.0" encoding="utf-8" ?>
- <customers>
- <customer name="steven">
- <id>011475</id>
- <fname>steven</fname>
- <age>36</age>
- <city>newyork</city>
- </customer>
- <customer name="julian">
- <id>52485</id>
- <fname>julian</fname>
- <age>22</age>
- <city>london</city>
- </customer>
- <customer name="dev">
- <id>5485</id>
- <fname>dev</fname>
- <age>26</age>
- <city>sydny</city>
- </customer>
- <customer name="ben">
- <id>485214</id>
- <fname>ben</fname>
- <age>36</age>
- <city>sydny</city>
- </customer>
- <customer name="john">
- <id>24586</id>
- <fname>John</fname>
- <age>42</age>
- <city>london</city>
- </customer>
- </customers>
- XDocument xdoc = XDocument.Load("D:\\customers.xml");
- // list box binding
- var customes = from customer in xdoc.Descendants("customers").Elements("customer").Attributes("name")
- select customer.Value;
- cmbCustomer.DataSource = customes.ToList();
- // Grid binding
- var customesList = from customers in xdoc.Root.Elements()
- name = customers.Element("fname").Value,
- id = customers.Element("id").Value,
- age = customers.Element("age").Value,
- city = customers.Element("city").Value
- };
- grdCustomer.DataSource = customesList.ToList();
- var whereList = from customers in xdoc.Root.Elements()
- where customers.Element("city").Value == "london"
- select new
- {
- name = customers.Element("fname").Value,
- city = customers.Element("city").Value
- };
- grdWhere.DataSource = whereList.ToList();
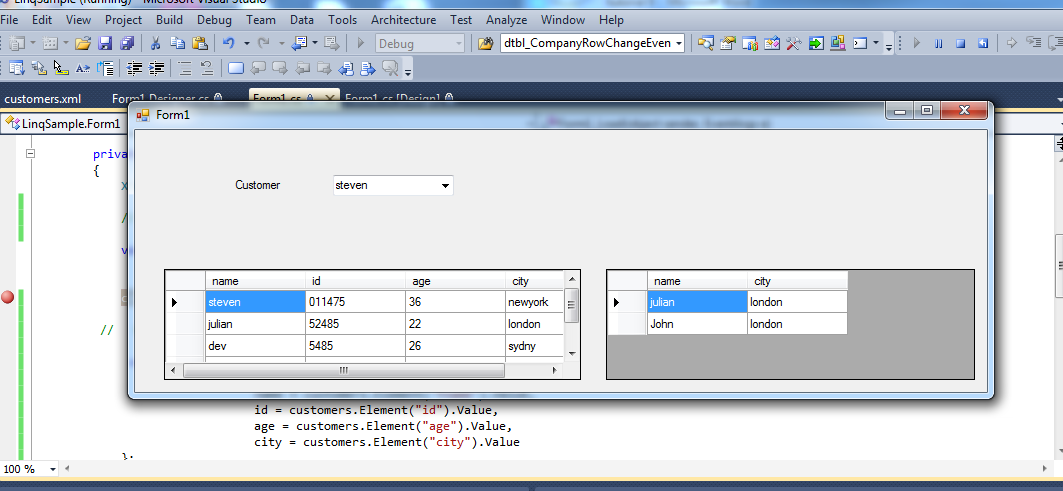
Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.
Add new comment
- 131 views