The Power of Data Structure Libraries in Modern Programming
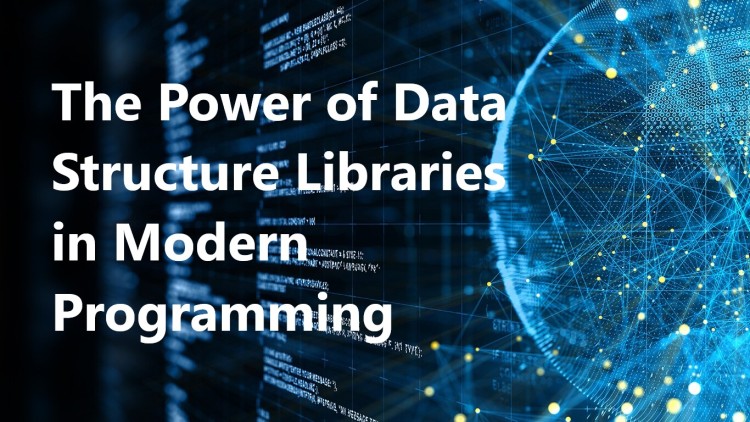
In the world of modern programming, data structure libraries are like the handy tools in a craftsman's toolbox. They may not always steal the spotlight, but they play a crucial role in shaping the final masterpiece. These libraries are the unsung heroes behind the scenes, simplifying complex tasks and making our lives as programmers a whole lot easier.
You see, in programming, data structures are the building blocks that allow us to organize and manipulate information efficiently. Think of them as containers for our data, like shelves in a library where we neatly store and retrieve books. But building and managing these structures from scratch can be a daunting task, consuming both time and brainpower.
This is where data structure libraries come into play. They offer a treasure trove of pre-built data structures and algorithms that can be effortlessly incorporated into your code. Whether you're dealing with lists, arrays, queues, stacks, or more intricate structures like trees and graphs, these libraries have got your back.
In this article, we'll explore how data structure libraries empower modern programmers. We'll delve into the practical benefits they bring to the table and how they streamline the development process. So, whether you're a seasoned coder or just starting your programming journey, fasten your seatbelt, and let's discover the power of data structure libraries in the world of modern programming.
Popular Data Structure Libraries and Implementations:
Let's take a look at some popular data structure libraries in various programming languages:
Python - NumPy and pandas
- NumPy provides efficient arrays for numerical computations. It's like a toolbox for numbers, making math easier for scientists and data analysts. You can do things like adding, multiplying, and dividing numbers in big arrays quickly.
- pandas offers data structures like DataFrames and Series. DataFrames are like spreadsheets that help you organize and analyze data. Series are like lists or columns of data. They make it easy to sort, filter, and manipulate data.
Java - Apache Commons Collections
- Apache Commons Collections makes Java's collections more powerful. It adds new ways to store and organize data, like bags for counting things, multisets for tracking multiple copies of items, and bidirectional maps for looking up values in both directions.
C++ - Boost C++ Libraries:
- Boost is a popular library for C++. It's like a treasure chest full of useful data structures. You can find things like multi-index containers that allow you to access data in multiple ways, circular buffers for managing data efficiently, and heaps for sorting elements.
JavaScript - Lodash:
- Lodash is a helper library for JavaScript. It gives you helpful tools for working with data. It has maps to store key-value pairs, sets for collections of unique values, and queues for managing tasks in a first-in, first-out order.
C# - System.Collections.Generic namespace:
- In C#, you have the System.Collections.Generic namespace, which provides different containers for holding data. There's List for sequences of items, Dictionary for key-value pairs, HashSet for unique items, and Queue for managing things in a first-in, first-out order.
Go - container package:
- Go has a package called "container" that's like a toolkit for managing data. It offers a heap for arranging data efficiently, a ring for circular lists, and a list for organizing items in a simple sequence.
Ruby - ActiveSupport::HashWithIndifferentAccess:
- Ruby's ActiveSupport extension brings extra data structures, like HashWithIndifferentAccess, which is like a special kind of hash. It lets you access data using either symbols or strings as keys, making it flexible and convenient.
Rust - std::collections module:
- In Rust, you can use the standard library's collections module, which provides different containers. There's Vec for dynamic arrays, HashMap for key-value pairs, and BTreeMap for sorted data. These make managing data easy in Rust.
Swift - Foundation framework:
- Swift relies on the Foundation framework, which offers data structures like Arrays for lists of items, Dictionaries for key-value pairs, and Sets for unique values. It also provides types for handling dates and times.
PHP - SPL (Standard PHP Library):
- PHP's SPL is like a library of data tools. It has structures like SplDoublyLinkedList for creating linked lists, SplPriorityQueue for handling priorities, and SplFixedArray for fixed-size arrays. These help PHP developers work with data effectively.These are some of the popular data structure libraries and implementations in common programming languages, which are widely accessible for various software development needs.
Using Data Structure Libraries in Your Code:
Using data structure libraries in your own code involves a few key steps:
-
Import or Include the Library: Import or include the library in your code to make its functions and data structures available.
-
Initialize the Data Structure: Create an instance of the data structure you want to use by calling the library's constructor or factory function.
-
Add or Retrieve Data: Use the library's methods to add data to or retrieve data from the data structure.
-
Perform Operations: Use the library's provided functions to perform various operations on the data structure.
-
Handle Errors: Be prepared to handle any errors or exceptions that may occur when using the library.
-
Dispose of Resources (if needed): Some libraries may require you to release resources or memory associated with the data structure when you're done with it.
-
Compile and Run: Compile or interpret your code, and then run your program to see how the data structure library functions within your application.
Python - NumPy and pandas:
Java - Apache Commons Collections:
C++ - Boost C++ Libraries:
The process is similar for other languages and libraries. You need to understand the library's documentation and APIs to effectively use the data structures they provide in your code.
Pros and Cons of Using Data Structure Libraries:
Pros of using data structure libraries:
- Efficiency: Libraries are often well-optimized for performance.
- Consistency: They provide standardized and reliable data structures.
- Reliability: Libraries are well-tested and reduce the likelihood of bugs.
- Time-saving: They save you time by offering pre-built data structures.
- Wider Community: Popular libraries have active user communities.
Cons of using data structure libraries:
- Learning Curve: You may need to invest time in learning how to use the library.
- Dependency: Your code becomes dependent on the library, which may lead to compatibility issues.
- Overhead: Libraries can add some overhead to your code.
- Limited Customization: You might be constrained by the library's capabilities.
- Code Bloat: Libraries can increase the size of your code.
Using data structure libraries can be a time-saving and efficient choice, but it may introduce a learning curve and potential drawbacks. Your decision to use a library should consider your project's specific needs and trade-offs.
In conclusion, data structure libraries make life easier for programmers. They're like ready-made tools that help organize and manage data. These libraries are widely available in many programming languages, saving time and effort. While they come with some pros and cons, they are valuable assets in the world of modern programming, helping developers build better software efficiently. So, whether you're a newbie or an experienced coder, data structure libraries are there to lend a helping hand in your programming journey.