How to Download File using PHP/MySQLi
Submitted by nurhodelta_17 on Monday, August 28, 2017 - 17:47.
Language
In my previous tutorial, I've created a Simple File Upload using PHP/MySQLi. As a follow-up, I've created another tutorial on how to create a Simple File Download. Most files can be easily download by clicking their link. However, some files cannot be downloaded by doing so. Thus, I've created this tutorial. This tutorial will not give you a good design, but will give you a good idea about the topic.
Creating our Database
First, we're going to create our database. This contains the location of our files. 1. Open phpMyAdmin. 2. Click databases, create a database and name it as "download". 3. After creating a database, click the SQL and paste the below code. See image below for detailed instruction.- CREATE TABLE `file` (
- `fileid` INT(11) NOT NULL AUTO_INCREMENT,
- `file_location` VARCHAR(150) NOT NULL,
- PRIMARY KEY(`fileid`)
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
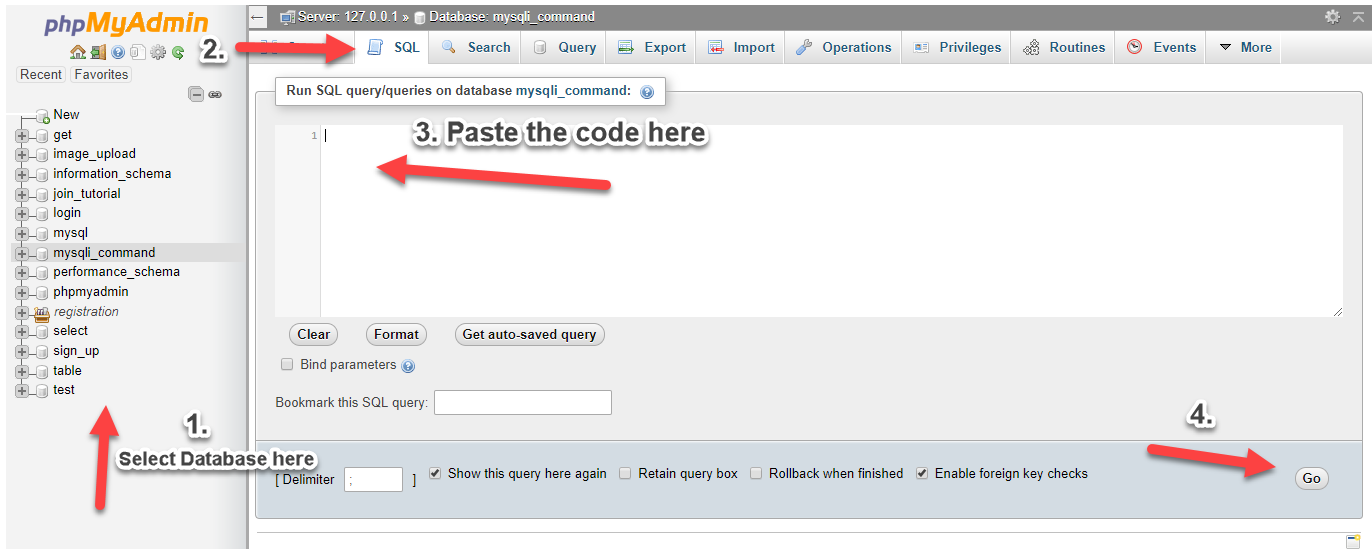
Inserting Data in to our Database
Next step is to insert example data into our database. This will serve as our sample data. 1. Click "dowload" database that we have created earlier. 2. Click SQL and paste the code below.- INSERT INTO `file` (`file_location`) VALUES
- ('upload/butterfly.jpeg'),
- ('upload/cherry.jpeg'),
- ('upload/rose.jpeg');
Creating our Connection
Next step is to create a database connection and save it as "conn.php". This file will serve as our bridge between our form and our database. To create the file, open your HTML code editor and paste the code below after the tag.- <?php
- //MySQLi Procedural
- if (!$conn) {
- }
- ?>
Creating our File Source
I've included in the file of this tutorial, the folder named "upload" which contains our sample files.Creating our Table
Next is to create our sample table. This contains the data that we inserted earlier and our download link. We name this as "index.php".- <!DOCTYPE html>
- <html>
- <head>
- <title>How to Download File using PHP/MySQLi</title>
- </head>
- <body>
- <h2>Sample Images</h2>
- <table border="1">
- <thead>
- <th>File Location</th>
- <th>Action</th>
- </thead>
- <tbody>
- <?php
- include('conn.php');
- ?>
- <tr>
- <td><?php echo $row['file_location']; ?></td>
- </tr>
- <?php
- }
- ?>
- </tbody>
- </table>
- </body>
- </html>
Creating our Download Code
Lastly, We create our download code. This code will download the file when user clicks the download link of that file. We name this as "download.php".- <?php
- }
- else {
- }
- ?>
Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.
Comments
Add new comment
- Add new comment
- 2122 views