How to Convert String Value to JSON Object
Introduction
In this tutorial we will create a How to Convert String Value to JSON Object. This tutorial purpose is to provide you way to convert your html string into JSON object. This will tackle the conversion and appending string to a JSON object. I will provide a sample program to show the actual coding of this tutorial.
This tutorial is simple and easy to understand just follow the instruction I provided and you will do it without a problem. This program is useful if you have some data that your want to be store as a JSON. I will try my best to give you the easiest way of creating this program String value to JSON conversion. So let's do the coding.
Before we get started:
This is the link for the template that i used for the layout design https://getbootstrap.com/.
Creating The Interface
This is where we will create a simple interface for our application. This code will a HTML form that we will be using to conversion. To create this simply copy and write it into your text editor, then save it as index.html.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- </div>
- </nav>
- <div class="col-md-6 well">
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="col-md-6">
- <div class="form-group">
- <input type="text" class="form-control" id="firstname"/>
- </div>
- <div class="form-group">
- <input type="text" class="form-control" id="lastname"/>
- </div>
- <div class="form-group">
- <input type="text" class="form-control" id="address"/>
- </div>
- </div>
- <div class="col-md-6">
- </div>
- </div>
- </body>
- </html>
Creating JavaScript Function
This is where the main function of the application is. This code will convert all the string value into JSON object button is clicked. To do this just copy and write these block of codes inside the text editor and save it as script.js.- const obj = '{"member":[]}';
- const json = JSON.parse(obj);
- function convertToJSON(){
- const firstname = document.getElementById('firstname');
- const lastname = document.getElementById('lastname');
- const address = document.getElementById('address');
- if(firstname.value == "" || lastname.value == "" || address.value == ""){
- alert("Please complete the required field!");
- }else{
- const str = '{"firstname": "'+firstname.value+'", "lastname": "'+lastname.value+'", "address": "'+address.value+'"}';
- json['member'].push(str);
- const decode = JSON.stringify(json, null, ' ');
- const rpl = decode.replace(/\\/g, '');
- document.getElementById('result').innerHTML = "<pre>"+rpl+"</pre>";
- firstname.value = "";
- lastname.value = "";
- address.value = "";
- }
- }
In this code we first create two variables called obj and json, in objwe create json string then parse the string into a json object. After that we created a method that will hold a certain functions. We first bind the form inputs and then contain them into a variable called str. Next we push all the string into the json object, and then use json.stringify().
Output:
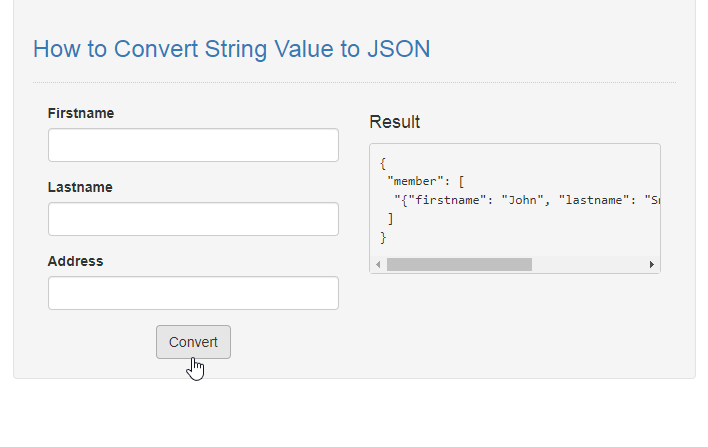
The How to Convert String Value to JSON Object source code that I provide can be download below. Please kindly click the download button.
There you have it we successfully created How to Convert String Value to JSON Object. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for JavaScript Language
Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.
Add new comment
- 450 views