Retrieving Data with CheckBox in VB.Net
Submitted by janobe on Monday, February 18, 2019 - 08:10.
In this tutorial, I will teach you how to retrieve data with checkbox in vb.net. This procedure is very useful if you want something to retrieve in the database that would then be displayed in the datagridview together with the checkboxes. If you want to find out how it’s done, check the instructions below.
For any questions about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
Or feel free to comment below.
Creating Database
Create a database and named it “profile” After that, execute the following query to create a table and insert some data in the table.- --
- -- Dumping data for table `profile`
- --
- (1, 'Jaison Joy', '<a href="mailto:[email protected]" rel="nofollow">[email protected]</a>', '<a href="http://jaisonjoy.com//image/man.png'" rel="nofollow">http://jaisonjoy.com//image/man.png'</a>, '2019-01-01'),
- (2, 'Vini', '<a href="mailto:[email protected]" rel="nofollow">[email protected]</a>', '<a href="http://jaisonjoy.com/image/female.png'" rel="nofollow">http://jaisonjoy.com/image/female.png'</a>, '2019-01-15'),
- (3, 'John', '<a href="mailto:[email protected]" rel="nofollow">[email protected]</a>', '<a href="http://jaisonjoy.com/image/male.png'" rel="nofollow">http://jaisonjoy.com/image/male.png'</a>, '2019-02-03'),
- (4, 'Test', 'Test', NULL, '2019-02-14'),
- (5, 'test2', 'Test2', NULL, '2019-02-14');
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application for visual basic.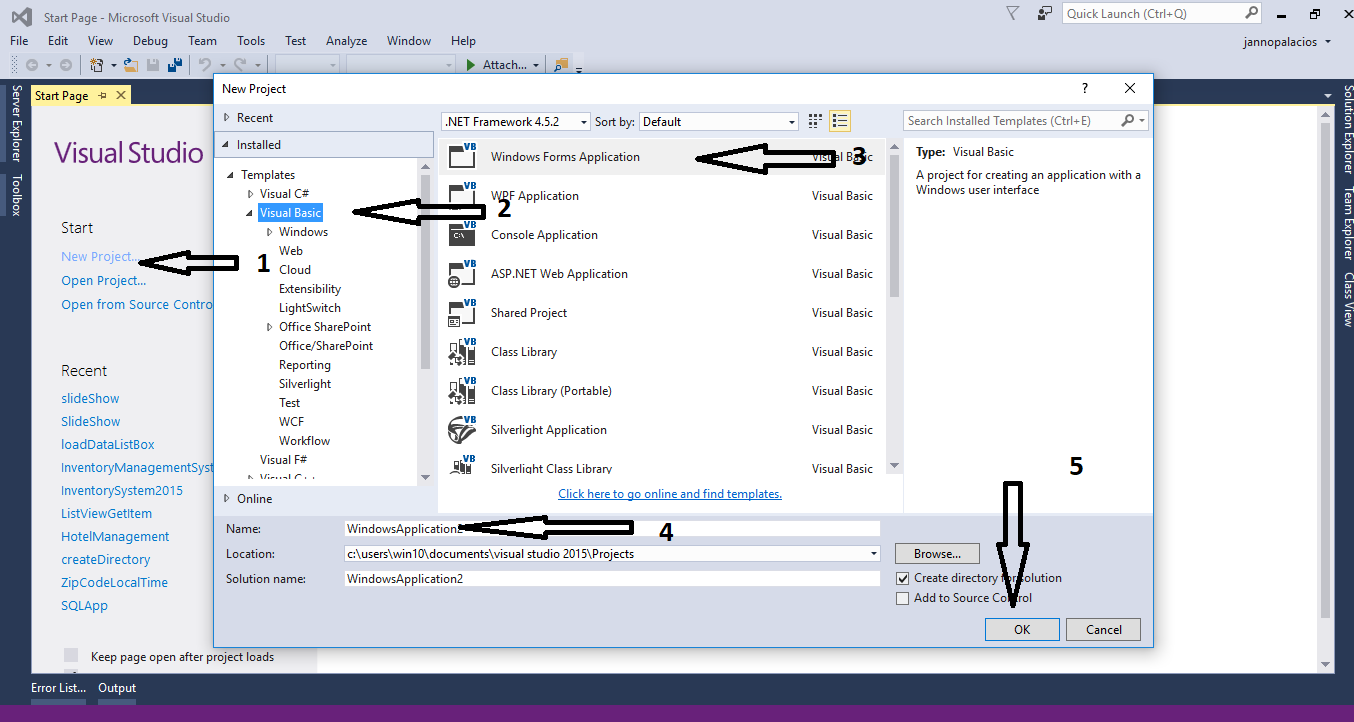
Step 2
Add a DataGridView inside the form. It will look like this.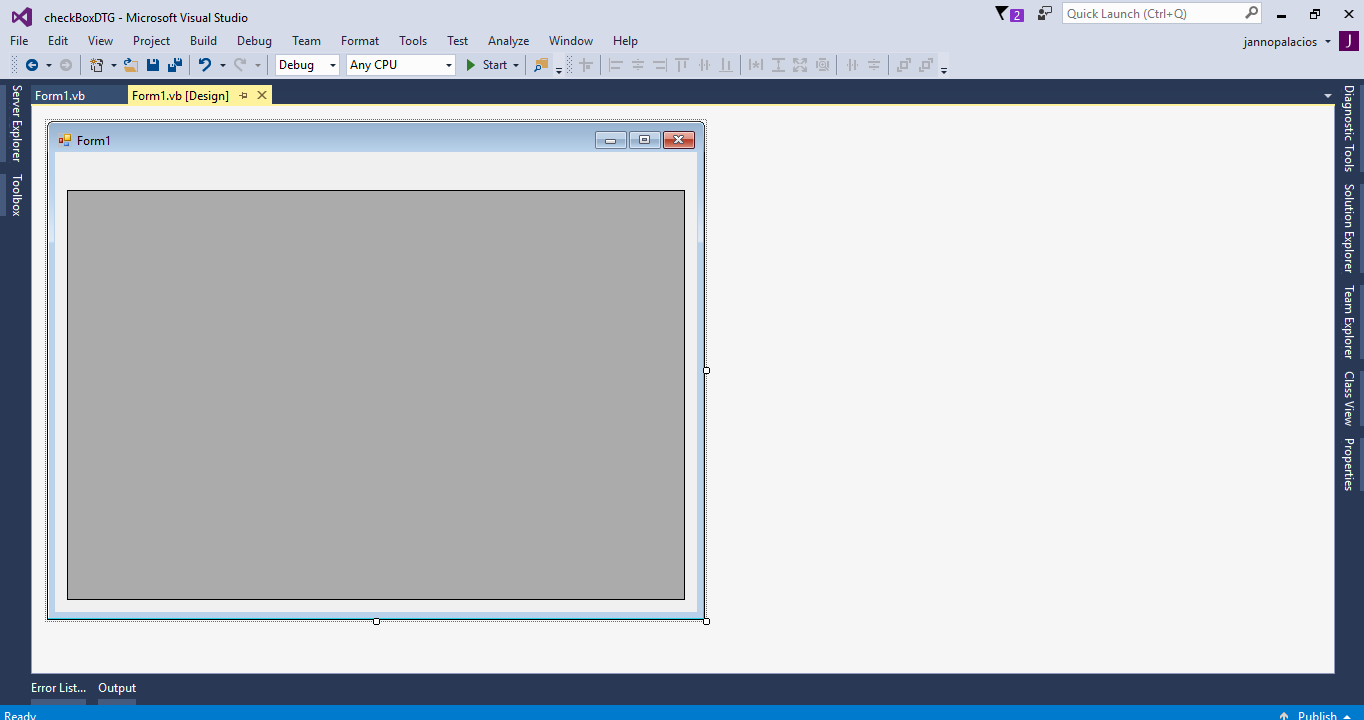
Step 3
Open the code edit by pressingF7
on the keyboard. In the code view, add a namespace above the class to access MySQL Libraries.
- Imports MySql.Data.MySqlClient
Step 4
Create a connection between MySQL and Visual Basic 2015 and declare all the classes that are needed.- Dim con As MySqlConnection = New MySqlConnection("server=localhost;user id=root;password=;database=profile;sslMode=none")
- Dim cmd As MySqlCommand
- Dim da As MySqlDataAdapter
- Dim dt As DataTable
- Dim sql As String
Step 5
Create a Sub Procedure in creating a CheckeBox object.- Private Sub CheckboxColumn()
- 'set a variable as a checkbox column in the DataGridView
- Dim chkbox As New DataGridViewCheckBoxColumn
- 'set the width of the column in the DataGridView
- With chkbox
- .Width = 30
- End With
- With DataGridView1
- 'Adding the checkbox column in the DataGridView
- .Columns.Add(chkbox)
- 'set the rows header to invisible
- .RowHeadersVisible = False
- End With
- End Sub
Step 6
Do the following code for retrieving data in the database to display it inside the DataGridView with CheckBoxes in the first load of the form.- Private Sub Form1_Load(sender As Object, e As EventArgs) Handles MyBase.Load
- Try
- sql = "SELECT * FROM `profile`"
- con.Open()
- cmd = New MySqlCommand()
- With cmd
- .Connection = con
- .CommandText = sql
- End With
- da = New MySqlDataAdapter()
- da.SelectCommand = cmd
- dt = New DataTable()
- da.Fill(dt)
- 'adding a checkbox column in the datagridview
- CheckboxColumn()
- 'display the data from the database to the datagridview
- DataGridView1.DataSource = dt
- Catch ex As Exception
- MsgBox(ex.Message)
- Finally
- con.Close()
- da.Dispose()
- End Try
- End Sub