Add, Edit, Delete with data table using PDO in PHP/MySQL
Submitted by alpha_luna on Saturday, May 14, 2016 - 10:54.
This simple project is created using PDO or it's called PHP Data Objects and it's a database driven using MySQL as a database. This project is intended for beginners in using PDO. It has a basic code so everyone can easily to understand and learn.
Add PDO Query
Result After Adding Data
Edit Form
Edit PDO Query
Result After Edditing Data
Modal Delete Form
Delete PDO Query
And, that's all, you can have Add, Edit, Delete with data table using PDO in PHP/MySQL or kindly click the "Download Code" button to download the full source code.
This is the full source code for Inserting Data, Updating Data, and Deleting Data in MySQL.
Share us your thoughts and comments below. Thank you so much for dropping by and reading this tutorial post. For more updates, don’t hesitate and feel free to visit this website more often and please share this with your friends or email me at [email protected]. Practice Coding. Thank you very much.
Creating our Table
We are going to make our database.- Open the PHPMyAdmin.
- Create a database and name it as "add_pdo".
- After creating a database name, then we are going to create our table. And name it as "student".
- Kindly copy the code below.
- CREATE TABLE IF NOT EXISTS `student` (
- `student_id` INT(100) NOT NULL AUTO_INCREMENT,
- `fname` VARCHAR(100) NOT NULL,
- `mname` VARCHAR(100) NOT NULL,
- `lname` VARCHAR(100) NOT NULL,
- `address` VARCHAR(100) NOT NULL,
- `email` VARCHAR(100) NOT NULL,
- PRIMARY KEY (`student_id`)
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1 AUTO_INCREMENT=4 ;
Database Connection
- <?php $conn = new PDO('mysql:host=localhost; dbname=add_pdo','root', ''); ?>
Add, Update, and Delete in PDO Query
Modal Add Form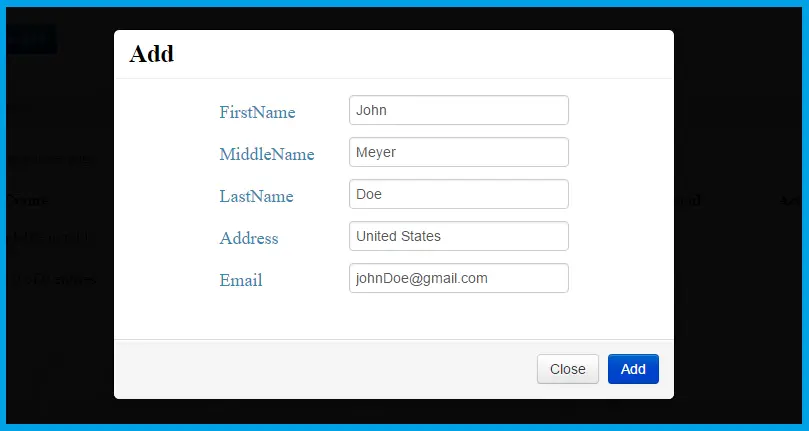
- <?php
- require_once('db.php');
- $fname= $_POST['fname'];
- $mname= $_POST['mname'];
- $lname= $_POST['lname'];
- $address= $_POST['address'];
- $email= $_POST['email'];
- $conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
- $sql = "INSERT INTO student (fname, mname, lname, address, email)
- VALUES ('$fname', '$mname', '$lname', '$address', '$email')";
- echo "<script>alert('Account successfully added!'); window.location='index.php'</script>";
- ?>
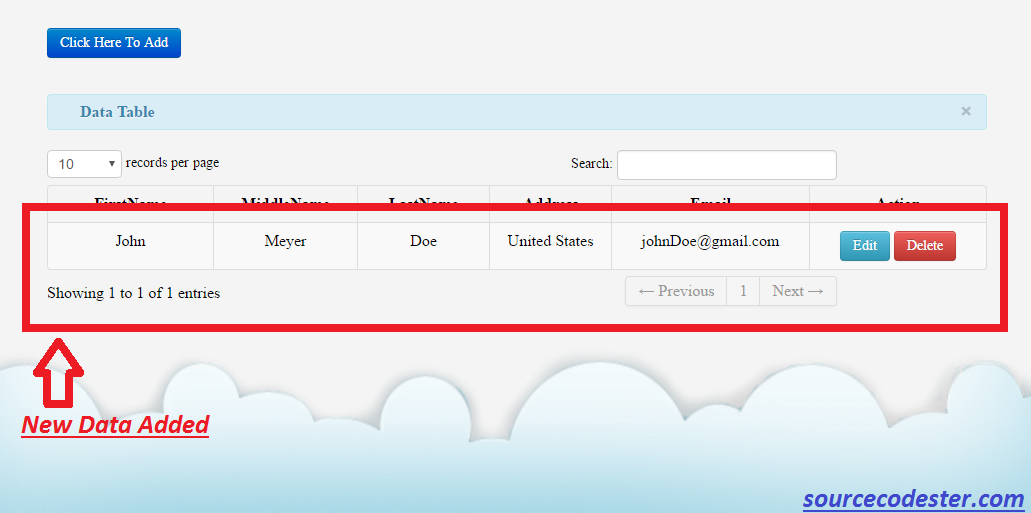
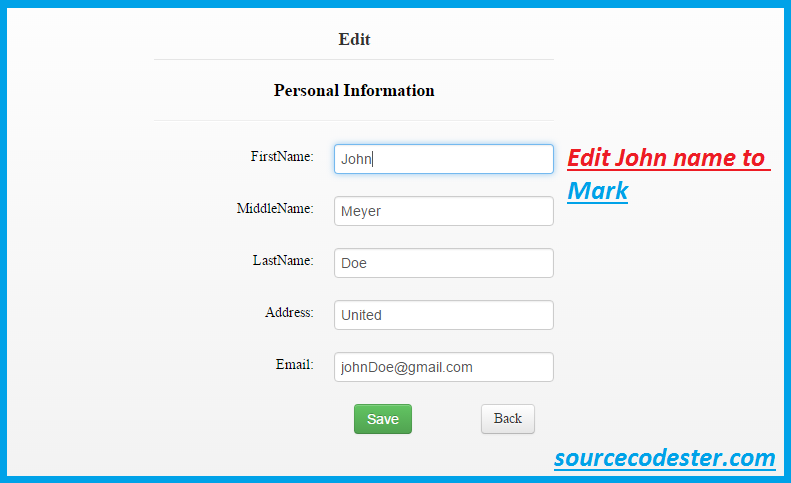
- <?php
- include('db.php');
- $result = $conn->prepare("SELECT * FROM student where student_id='$ID'");
- $result->execute();
- for($i=0; $row = $result->fetch(); $i++){
- $id=$row['student_id'];
- ?>
- <form class="form-horizontal" method="post" action="edit_PDO.php<?php echo '?student_id='.$id; ?>" enctype="multipart/form-data" style="float: right;">
- <hr>
- <div class="control-group">
- <div class="controls">
- <input type="text" name="fname" required value=<?php echo $row['fname']; ?>>
- </div>
- </div>
- <div class="control-group">
- <div class="controls">
- <input type="text" name="mname" required value=<?php echo $row['mname']; ?>>
- </div>
- </div>
- <div class="control-group">
- <div class="controls">
- <input type="text" name="lname" required value=<?php echo $row['lname']; ?>>
- </div>
- </div>
- <div class="control-group">
- <div class="controls">
- <input type="text" name="address" required value=<?php echo $row['address']; ?>>
- </div>
- </div>
- <div class="control-group">
- <div class="controls">
- <input type="email" name="email" required value=<?php echo $row['email']; ?>>
- </div>
- </div>
- <div class="control-group">
- <div class="controls">
- </div>
- </div>
- </form>
- <?php } ?>
- <?php
- include 'db.php';
- $get_id=$_REQUEST['student_id'];
- $fname= $_POST['fname'];
- $mname= $_POST['mname'];
- $lname= $_POST['lname'];
- $address= $_POST['address'];
- $email= $_POST['email'];
- $sql = "UPDATE student SET fname ='$fname', mname ='$mname', lname ='$lname',
- address ='$address', email ='$email' WHERE student_id = '$get_id' ";
- echo "<script>alert('Successfully Edit The Account!'); window.location='index.php'</script>";
- ?>
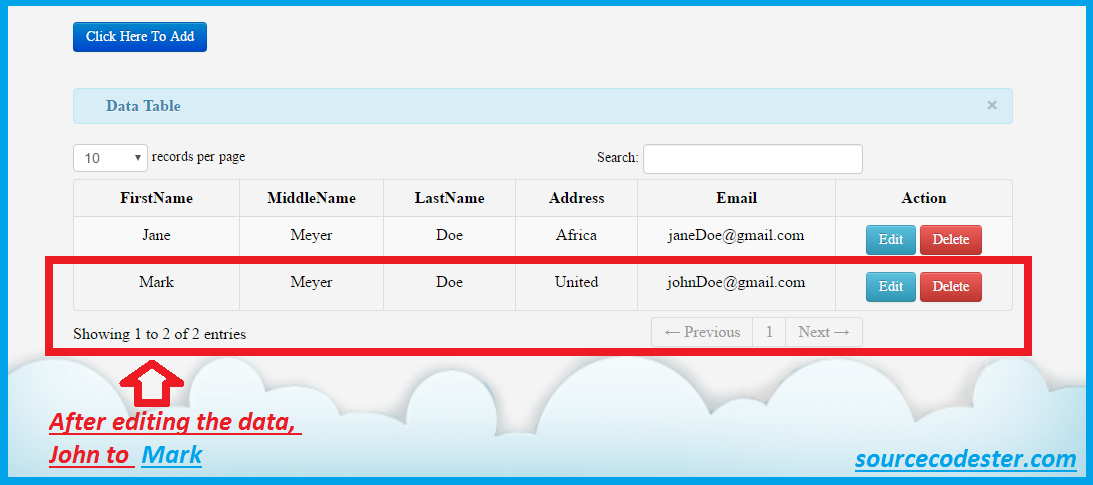
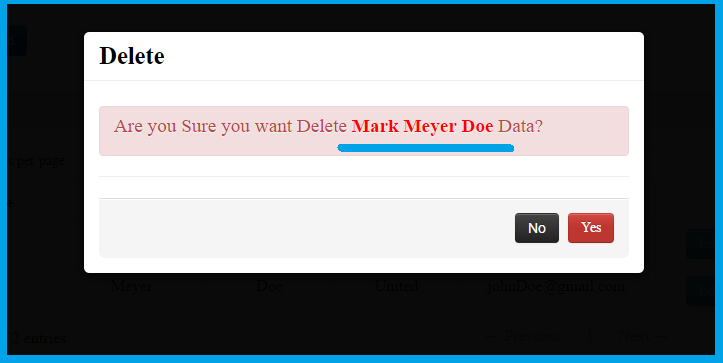
Result After Deleting Data
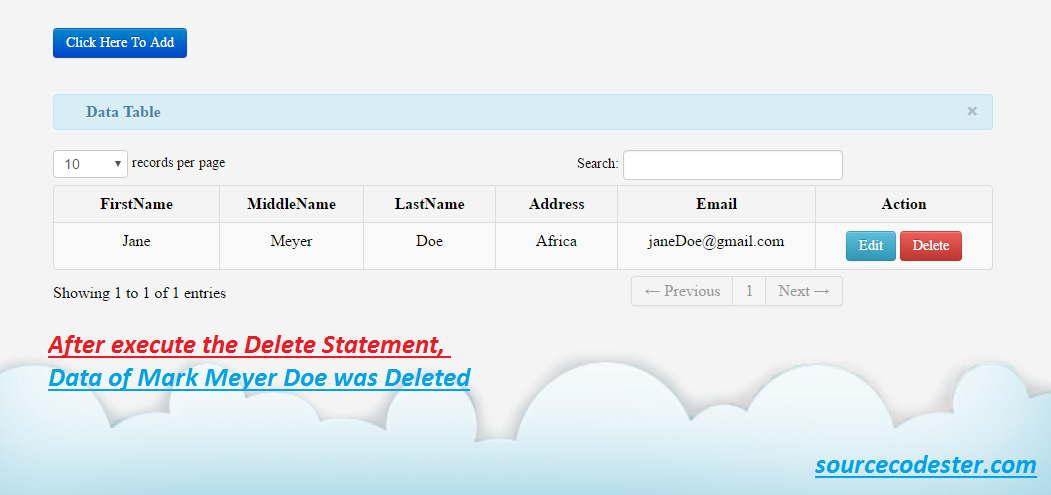
Comments
Add, Edit, Delete Image with data table using PDO in PHP/MySQL
hi, do you know how to add a picture to the data. can you send me the same project with picture added please. thank you
I have tried this so many…
I have tried this so many times I cannot get this to work for me, I keep getting close, I have had to modify the DB.php to another one I found online cause this one didn't work at all for me, I have finally gotten to the point where the main page finds the records I have in the database, but it will not display them, the table says there are 14 entries, and there are but it will not display them. I am not a coder, but I have changed them.. https://bt.inokamanori.com/dataupdate1/index.php.
I am at a loss.
the error I am getting now is.
[02-Feb-2022 18:51:47 UTC] PHP Warning: Trying to access array offset on value of type bool in /home/g88pa0itn9u7/bt.inokamanori.com/dataupdate1/index.php on line 37
[02-Feb-2022 18:51:47 UTC] PHP Warning: Trying to access array offset on value of type bool in /home/g88pa0itn9u7/bt.inokamanori.com/dataupdate1/index.php on line 40
[02-Feb-2022 18:51:47 UTC] PHP Warning: Trying to access array offset on value of type bool in /home/g88pa0itn9u7/bt.inokamanori.com/dataupdate1/index.php on line 41
[02-Feb-2022 18:51:47 UTC] PHP Warning: Trying to access array offset on value of type bool in /home/g88pa0itn9u7/bt.inokamanori.com/dataupdate1/index.php on line 42
[02-Feb-2022 18:51:47 UTC] PHP Warning: Trying to access array offset on value of type bool in /home/g88pa0itn9u7/bt.inokamanori.com/dataupdate1/index.php on line 43
[02-Feb-2022 18:51:47 UTC] PHP Warning: Trying to access array offset on value of type bool in /home/g88pa0itn9u7/bt.inokamanori.com/dataupdate1/index.php on line 44
[02-Feb-2022 18:51:47 UTC] PHP Warning: Trying to access array offset on value of type bool in /home/g88pa0itn9u7/bt.inokamanori.com/dataupdate1/index.php on line 56
[02-Feb-2022 18:51:47 UTC] PHP Warning: Trying to access array offset on value of type bool in /home/g88pa0itn9u7/bt.inokamanori.com/dataupdate1/index.php on line 56
[02-Feb-2022 18:51:47 UTC] PHP Warning: Trying to access array offset on value of type bool in /home/g88pa0itn9u7/bt.inokamanori.com/dataupdate1/index.php on line 56