Are you new to the exciting world of computer science and programming? Curious about data structures and algorithms but unsure where to start? You're in the right place! In this beginner's guide, we'll take you on a journey through the fascinating basics of data structures and algorithms.
These concepts might sound complex, but we promise to keep them simple and straightforward. Think of data structures as the way your computer stores and organizes data, such as lists or collections. Algorithms, on the other hand, are like recipes for solving problems with that data.
Imagine you have a jigsaw puzzle; the pieces are your data, and the process of putting them together is the algorithm. It's all about organizing, searching, and sorting your data efficiently.
We'll help you understand how data structures and algorithms are the building blocks of software and applications. Whether you're dreaming of becoming a programmer, tackling coding challenges, or just want to grasp the basics of computer science, our beginner's guide will give you the confidence to start your journey.
No prior technical knowledge is required – we're here to make it accessible and fun. So, let's begin your adventure into the world of data structures and algorithms. Ready to dive in?
What Are Data Structures and Algorithms?
Data Structures are a way to organize and store data in a computer's memory. Think of them as containers that hold information. For example, arrays are like lists, and they store multiple pieces of data in an ordered way. Linked lists are like chains of connected pieces of data. Trees and graphs organize data in a hierarchical or networked structure. These structures help programmers manage and manipulate data efficiently.
Algorithms, on the other hand, are like step-by-step instructions for solving problems or performing tasks using data structures. They are like recipes for the computer. Algorithms define how to do things with the data in these structures. Sorting a list of numbers from smallest to largest, searching for a specific item in a list, or finding the shortest route between two points on a map are all tasks that can be accomplished with algorithms.
These two concepts work together in the following way:
- Choosing the Right Data Structure: When you have data to work with, you need to pick the most suitable data structure. For instance, if you need to store a list of items in a specific order and easily access or modify them, you might choose an array or a linked list.
- Implementing Algorithms: Once you've selected the appropriate data structure, you need to create algorithms to perform tasks on that data. For example, if you have an array of numbers and you want to find the largest one, you would use an algorithm that goes through the array and keeps track of the largest number it has seen.
- Efficiency: The choice of data structure and the efficiency of your algorithms are closely related. Some data structures are better suited for certain tasks. For instance, a binary search algorithm works well with sorted data but not so well with unsorted data. So, choosing the right data structure and algorithm can greatly impact the speed and efficiency of your program.
- Problem Solving: When faced with a problem, you often need to figure out how to represent the problem's data with the appropriate data structure. Then, you design an algorithm to solve the problem efficiently. This is a common process in programming.
In essence, data structures provide the storage, and algorithms provide the instructions for working with data in an organized and efficient way. Understanding and using them effectively is crucial for writing efficient and powerful computer programs. They're the tools that help computers process and manage information effectively, making them the foundation of computer science and software development.
Why Are Data Structures and Algorithms Important?
Data structures and algorithms are important because they are the foundation of computer programming and problem-solving. They help us organize and process data efficiently, making software faster, more reliable, and easier to maintain. Here's a simple explanation:
- Efficient Data Organization: Data structures allow us to store and organize information in a way that makes it easy to access and manipulate. Imagine a library with well-organized shelves – it's easier to find books, right? Data structures are like those shelves, making data more manageable.
- Speeding Up Tasks: Algorithms are like step-by-step instructions for solving problems. They help us perform tasks faster and with less effort. For example, when searching for a name in a phone book, following a systematic order is much quicker than checking each page randomly. Algorithms provide that systematic order in computer programs.
- Saving Time and Resources: Well-designed algorithms and data structures can significantly reduce the time and resources needed to perform tasks. This is crucial in software development, where efficiency can mean the difference between a fast, responsive application and a slow, frustrating one.
- Scalability: As programs and data grow, efficient data structures and algorithms ensure that the software remains responsive. Think of it as a kitchen – whether you're cooking for one or one hundred, a well-organized kitchen with efficient tools makes the job easier.
- Problem Solving: They help in problem-solving. Like a recipe for baking a cake, algorithms provide a clear path to solve a problem. They're essential for tasks like finding the shortest route on a map, sorting a list of names, or searching for specific data in a massive database.
- Reducing Errors: When data is organized well, and algorithms are implemented correctly, it's less likely that errors will occur in the program. This leads to more reliable software.
- Universal Application: Data structures and algorithms are not limited to a specific programming language or platform. They're universal tools that any programmer can use to create efficient and reliable software, no matter the context. Data structures and algorithms are the tools that programmers use to make software work better, faster, and more reliably. They're like the building blocks of computer programs, helping us solve a wide range of problems in a structured and efficient way.
Example: Finding the Maximum Number in a List
Data Structure: Array
Algorithm: Linear Search
- Data Structure: You have a list of numbers, and you decide to use an array to store them. An array is a data structure that allows you to store a collection of items in a specific order.
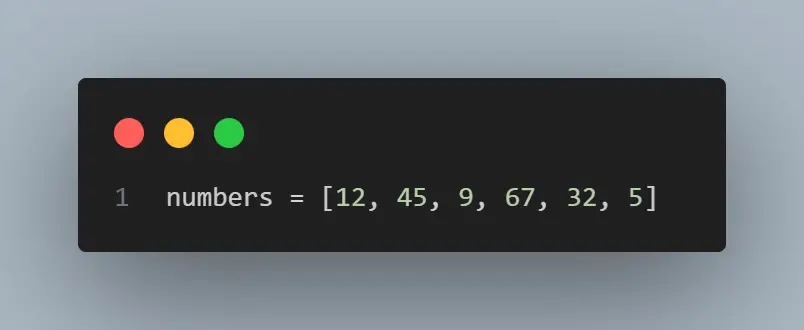
- Algorithm: You want to find the maximum number in the list, so you decide to use a linear search algorithm—a simple method that checks each number in the array one by one.
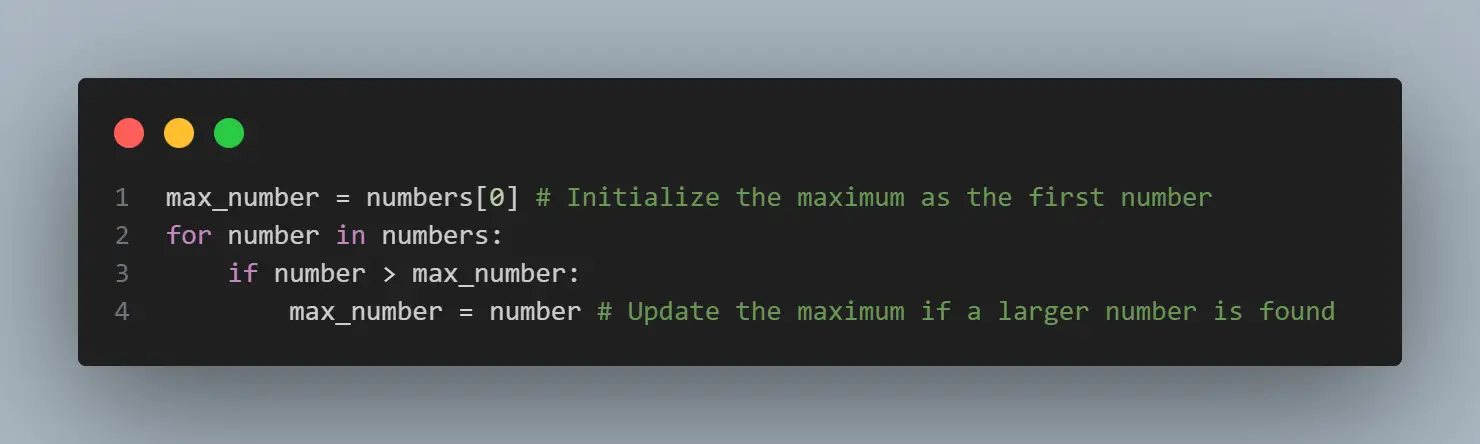
- Working Together: In this example, the data structure (the array) holds the data with which you want to work, and the algorithm (linear search) guides you on how to process that data. The algorithm goes through the array, compares each number with the current maximum, and updates the maximum if a larger number is found.
This is a basic illustration of how data structures and algorithms collaborate to solve a specific problem. Different data structures and algorithms are used for various tasks, and the choice of the right combination is crucial for efficiency and effectiveness in programming.
Conclusion
In summary, data structures and algorithms are essential tools in computer science and programming. They help us organize information efficiently, perform tasks faster, and reduce errors in software. Whether you're a beginner or an experienced programmer, understanding these concepts is the key to building better and more reliable computer programs. So, if you're starting your journey in the world of computer science, remember that data structures and algorithms are your reliable companions on this exciting adventure. Happy coding!