Byte Converter using VB6.0
Submitted by donbermoy on Wednesday, April 30, 2014 - 21:48.
Here in this tutorial, we will create a program that convert bits, bytes, kilobytes, megabytes, gigabytes, and terabytes to their corresponding bytes cases. We all know that 1 byte is equal to 8 bits and 1 kilobyte is equal to 1024 bytes. So that is the formula that we will going to use in this tutorial.
Now, let's start this tutorial!
1.Let's start this tutorial by following the following steps in Microsoft Visual Basic 6.0: Open Microsoft Visual Basic 6.0, click Choose Standard EXE, and click Open.
2. Next, add only one Button named Command1 and labeled it as "Convert". Insert two combobox that will serve as unit of bytes named Combo1 and Combo2. Insert also two textbox named Text1 for inputting a desired value and Text2 for displaying the byte conversion. You must design your interface like this:
3. Put this code in Form_Load to load the unit of bytes in the combobox.
4. In your button1 as the conversion button, put this code below.
Initialize num1 as integer and make that as a valid number in input.
Have a code for select case in your combo1.text as the inputted unit and the output unit will be in Combobox2.
For Bits Conversion:
For Byte Conversion:
For Kilobytes Conversion:
For Megabytes Conversion:
For Gigabytes Conversion:
For Terabytes Conversion:
Output:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
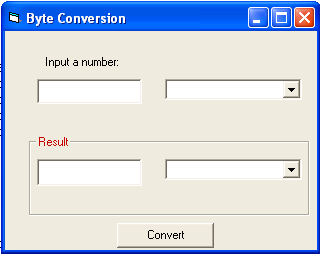
- Private Sub Form_Load()
- Combo1.AddItem ("Bits")
- Combo1.AddItem ("Bytes")
- Combo1.AddItem ("Kilobytes")
- Combo1.AddItem ("Megabytes")
- Combo1.AddItem ("Gigabytes")
- Combo1.AddItem ("Terabytes")
- Combo2.AddItem ("Bits")
- Combo2.AddItem ("Bytes")
- Combo2.AddItem ("Kilobytes")
- Combo2.AddItem ("Megabytes")
- Combo2.AddItem ("Gigabytes")
- Combo2.AddItem ("Terabytes")
- End Sub
- Dim num1 As Integer
- num1 = Val(Text1.Text)
- If Not IsNumeric(num1) Then
- MsgBox ("Make sure your conversion is numeric.")
- Exit Sub
- End If
- Select Case Combo1.Text
- Case "Bits"
- With Combo2
- If .Text = "Bits" Then Text2.Text = num1
- If .Text = "Bytes" Then Text2.Text = num1 / 8
- If .Text = "Kilobytes" Then Text2.Text = num1 / 8 / 1024
- If .Text = "Megabytes" Then Text2.Text = num1 / 8 / 1024 / 1024
- If .Text = "Gigabytes" Then Text2.Text = num1 / 8 / 1024 / 1024 / 1024
- If .Text = "Terabytes" Then Text2.Text = num1 / 8 / 1024 / 1024 / 1024 / 1024
- End With
- Case "Bytes"
- With Combo2
- If .Text = "Bits" Then Text2.Text = num1 * 8
- If .Text = "Bytes" Then Text2.Text = num1
- If .Text = "Kilobytes" Then Text2.Text = num1 / 1024
- If .Text = "Megabytes" Then Text2.Text = num1 / 1024 / 1024
- If .Text = "Gigabytes" Then Text2.Text = num1 / 1024 / 1024 / 1024
- If .Text = "Terabytes" Then Text2.Text = num1 / 1024 / 1024 / 1024 / 1024
- End With
- Case "Kilobytes"
- With Combo2
- If .Text = "Bits" Then Text2.Text = num1 * 1024 * 8
- If .Text = "Bytes" Then Text2.Text = num1 * 1024
- If .Text = "Kilobytes" Then Text2.Text = num1
- If .Text = "Megabytes" Then Text2.Text = num1 / 1024
- If .Text = "Gigabytes" Then Text2.Text = num1 / 1024 / 1024
- If .Text = "Terabytes" Then Text2.Text = num1 / 1024 / 1024 / 1024
- End With
- Case "Megabytes"
- With Combo2
- If .Text = "Bits" Then Text2.Text = num1 * 1024 * 1024 * 8
- If .Text = "Bytes" Then Text2.Text = num1 * 1024 * 1024
- If .Text = "Kilobytes" Then Text2.Text = num1 * 1024
- If .Text = "Megabytes" Then Text2.Text = num1
- If .Text = "Gigabytes" Then Text2.Text = num1 / 1024
- If .Text = "Terabytes" Then Text2.Text = num1 / 1024 / 1024
- End With
- Case "Gigabytes"
- With Combo2
- If .Text = "Bits" Then Text2.Text = num1 * 1024 * 1024 * 1024 * 8
- If .Text = "Bytes" Then Text2.Text = num1 * 1024 * 1024 * 1024
- If .Text = "Kilobytes" Then Text2.Text = num1 * 1024 * 1024
- If .Text = "Megabytes" Then Text2.Text = num1 * 1024
- If .Text = "Gigabytes" Then Text2.Text = num1
- If .Text = "Terabytes" Then Text2.Text = num1 / 1024
- End With
- Case "Terabytes"
- With Combo2
- If .Text = "Bits" Then Text2.Text = num1 * 1024 * 1024 * 1024 * 1024 * 8
- If .Text = "Bytes" Then Text2.Text = num1 * 1024 * 1024 * 1024 * 1024
- If .Text = "Kilobytes" Then Text2.Text = num1 * 1024 * 1024 * 1024
- If .Text = "Megabytes" Then Text2.Text = num1 * 1024 * 1024
- If .Text = "Gigabytes" Then Text2.Text = num1 * 1024
- If .Text = "Terabytes" Then Text2.Text = num1
- End With
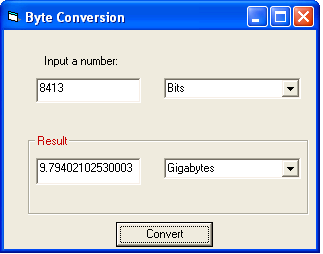