Storing Data and Autocomplete ComboBox
Submitted by janobe on Friday, December 20, 2013 - 17:59.
For today, I will teach you how to create an Autocomplete ComboBox and Store data in MS Access Database. In doing the Autocomplete ComboBox there’s no need for you to dropdown the ComboBox every time you search for your list. All you have to do is type the initial letter in the ComboBox that you’re going to search and the records will automatically dropdown. And that’s how easy it is.
So, let’s begin.
1. Open Visual Basic 2008
2. Create a project.
3. Create a windows Form.
4. Set up your form just like this.
Click the ComboBox , go to the properties, click the AutocompleteMode and select the SuggestAppend. Then click the AutoCompleteSource and select the ListItems.
After setting up the properties of the ComboBox double click the Form and do this code.
Go to
Go back to the Design Views, double click the Save Button and do this code for saving your data.
Complete Source Code is included. Download and run it on your computer.
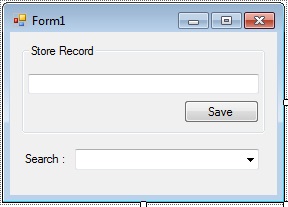
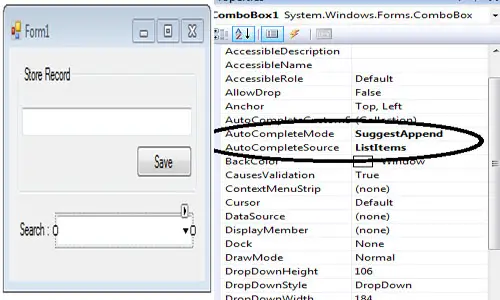
- Public Class Form1
- 'set up connection to MS Access database
- Dim con As OleDb.OleDbConnection = New OleDb.OleDbConnection("Provider=Microsoft.ACE.OLEDB.12.0;Data Source=" & _
- Application.StartupPath & "\datastore.accdb")
- 'represent the set of commands and a database for filling the dataset and update the datatsource.
- Dim da As New OleDb.OleDbDataAdapter
- 'contains basic elements of the database such as table , indexes ,keys and the relation of the tables
- Dim ds As New DataSet
- 'variable string, where i put a query oon it.
- Dim sql As String
- End Class
Form_load
and set up a code for filling the ComboBox on the first load.
- Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
- Try
- 'open the connection
- con.Open()
- 'set your query to a variable(sql)
- sql = "Select * From tbllist"
- 'set new dataset because every time you call the Form_load it execute new dataset in the database
- ds = New DataSet
- da = New OleDb.OleDbDataAdapter(sql, con)
- 'fill and add or refreshes rows in the dataset
- da.Fill(ds, "tbllist")
- 'set your datasource to combobox
- ComboBox1.DataSource = ds.Tables(0)
- 'gets or set the property to display in the listcontrol
- ComboBox1.DisplayMember = "list"
- Catch ex As Exception
- End Try
- 'close the connection
- End Sub
- Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
- Try
- 'open the connection
- con.Open()
- 'set your query to a variable(sql)
- sql = "INSERT INTO tbllist (list) Values ('" & TextBox1.Text & "')"
- da = New OleDb.OleDbDataAdapter(sql, con)
- 'fill and add or refreshes rows in the dataset
- da.Fill(ds)
- Catch ex As Exception
- End Try
- 'close the connection
- 'call the form_load to refresh the records in the combobox.
- Call Form1_Load(sender, e)
- End Sub