Retrieving Data Using DateTimePicker in VB.Net
Submitted by janobe on Friday, February 15, 2019 - 11:19.
In this tutorial, I will teach you how to retrieve data using datetimepicker in vb.net. This method has the ability to filter the data in a specific date by using the datetimepicker. In this way, you will be able to find out how many records will appear in the date that you have selected. See the procedure below.
For any questions about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
Or feel free to comment below.
Creating Database
Create a database named “profile” Do the following query to create a table and add the data inside the table.- --
- -- Dumping data for table `profile`
- --
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application in visual basic.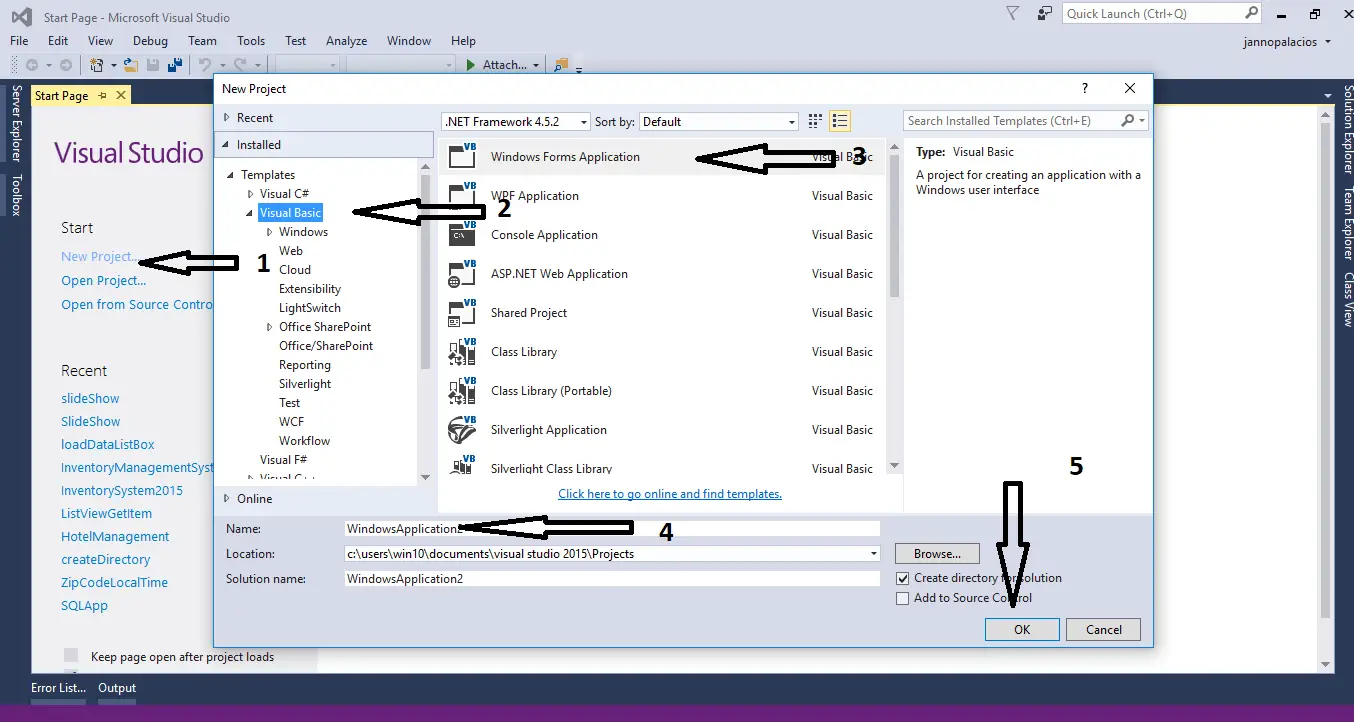
Step 2
Do the form just like shown below.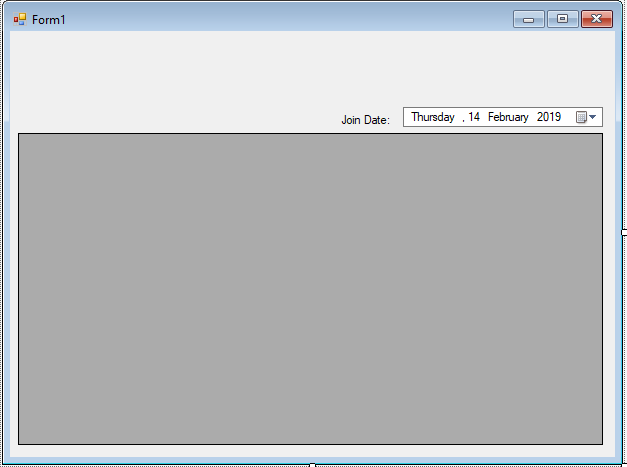
Step 3
Go to the code view and add a namespace for mysql above the class.- Imports MySql.Data.MySqlClient
Step 4
Inside the class, create a connection between MySQL and Visual Basic 2015. After that, declare all the classes that are needed.- Dim con As MySqlConnection = New MySqlConnection("server=localhost;user id=root;password=;database=profile;sslMode=none")
- Dim cmd As MySqlCommand
- Dim da As MySqlDataAdapter
- Dim dt As DataTable
- Dim sql As String
Step 5
Create a function for retrieving data in the database- Dim con As MySqlConnection = New MySqlConnection("server=localhost;user id=root;password=;database=profile;sslMode=none")
- Dim cmd As MySqlCommand
- Dim da As MySqlDataAdapter
- Dim dt As DataTable
- Dim sql As String
Step 6
Do the following codes for retrieving all the data in the database that will be display inside the datagridview in the first load of the form.- Private Sub Form1_Load(sender As Object, e As EventArgs) Handles MyBase.Load
- sql = "SELECT * FROM `profile`"
- DataGridView1.DataSource = findData(sql)
- End Sub
Step 7
Write this code for filtering all the data that will be display inside the datagridview when the date is changed.- Private Sub DateTimePicker1_ValueChanged(sender As Object, e As EventArgs) Handles DateTimePicker1.ValueChanged
- Dim todates As String
- todates = Format(DateTimePicker1.Value, "yyyy-MM-dd")
- sql = "SELECT * FROM `profile` WHERE DATE(joindate) = '" & todates & "'"
- DataGridView1.DataSource = findData(sql)
- End Sub