How to Shake a Form in VB.NET
Submitted by donbermoy on Wednesday, May 6, 2015 - 23:30.
This is a tutorial that will teach you how to create a form shaker in vb.net.
Now, let's start this tutorial!
1. Let's start with creating a Windows Form Application for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application.
2. Next, add two Buttons named Button1 for Left-Right shake and Button2 for Up-Down shake button. You must design your interface like this:
3. Now, we will do the coding.
First, we will create a module named Shaker.
Declare and instantiate the following variables.
We will create a property named timeForPauseBetweenMove to pause between move of the shake.
To get the instantiation of our Form1, we will make a sub procedure named New.
We will create a property named moveDirection to determine the direction of the shake.
Now, here's the code for shaking the form.
To locate the position of the form.
To start shaking the form. Here's the code below:
4. Then, will go back to code for our Form.
Call the Shaker module and initialize it.
In your Button1_Click, have this code below to trigger to shake your form left and right with 50ms to shake.
In your Button2_Click, have this code below to trigger to shake your form up and down with 50ms to shake.
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
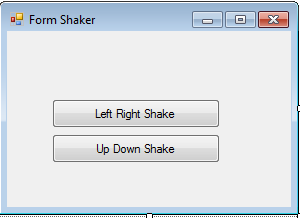
- Private frm As Form
- Private howMuch As Integer
- Private th As Threading.Thread
- Private timeForPause As Integer
- Public Shared MOVE_UP_DOWN As Integer = 0
- Public Shared MOVE_LEFT_RIGHT As Integer = 1
- Private direction As Integer
- Public Property timeForPauseBetweenMove As Integer
- Get
- Return timeForPause
- End Get
- Set(ByVal value As Integer)
- timeForPause = value
- End Set
- End Property
- Sub New(ByVal frm As Form, ByVal howMuchToMove As Integer)
- Me.frm = frm
- howMuch = howMuchToMove
- End Sub
- Public Property timeForPauseBetweenMove As Integer
- Get
- Return timeForPause
- End Get
- Set(ByVal value As Integer)
- timeForPause = value
- End Set
- End Property
- Sub shake()
- Dim tempLoc As Point = frm.Location
- Dim startLoc As Point = New Point(frm.Location.X, frm.Location.Y)
- Select Case moveDirection
- Case MOVE_LEFT_RIGHT
- For a As Integer = howMuch To 0 Step -1
- Dim poss As New ff(AddressOf formPosition)
- frm.Invoke(poss, New Point(startLoc.X - a, startLoc.Y))
- Threading.Thread.Sleep(timeForPauseBetweenMove)
- frm.Invoke(poss, New Point(startLoc.X + a, startLoc.Y))
- Threading.Thread.Sleep(timeForPauseBetweenMove)
- Next
- Case MOVE_UP_DOWN
- For a As Integer = howMuch To 0 Step -1
- Dim poss As New ff(AddressOf formPosition)
- frm.Invoke(poss, New Point(startLoc.X, startLoc.Y - a))
- Threading.Thread.Sleep(timeForPauseBetweenMove)
- frm.Invoke(poss, New Point(startLoc.X, startLoc.Y + a))
- Threading.Thread.Sleep(timeForPauseBetweenMove)
- Next
- End Select
- Dim pos As New ff(AddressOf formPosition)
- frm.Invoke(pos, startLoc)
- End Sub
- Sub formPosition(ByVal p As Point)
- frm.Location = p
- End Sub
- Sub startShake()
- Try
- th = New System.Threading.Thread(AddressOf shake)
- th.Start()
- Catch ex As Exception
- MessageBox.Show(ex.Message)
- End Try
- End Sub
- Dim s As New Shaker(Me, 50)
- Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
- s.moveDirection = Shaker.MOVE_LEFT_RIGHT
- s.timeForPauseBetweenMove = 50
- s.startShake()
- End Sub
- Private Sub Button2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button2.Click
- s.moveDirection = Shaker.MOVE_UP_DOWN
- s.timeForPauseBetweenMove = 50
- s.startShake()
- End Sub