Creating Threads in VB.NET
Submitted by donbermoy on Saturday, June 21, 2014 - 18:02.
Today in VB.NET, I will teach you how to create threads. We all know that a thread is a basic processing unit in our computer to which an operating system allocates processor time, and more than one thread can be executing code inside a process. Thus, threads have priority. It is just like a racing program.
Now, let's start this tutorial!
1. Let's start with creating a Windows Form Application for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Console Application.
Note: We will not design any interface because we will program it using vb console application.
2. In your code module, put this code below.
We used the package System.Threading to access the thread class. We have created three methods named CarA, CarB, and CarC that has an I variable that will write their Car names and has a delay of with the sleep method and its time in millisecond. If variable I will reach 250 then the console application will close after executing the threads. Next, in our Main, we have created the three threads using the addresses of the methods that we have created above. And to start the thread, we use the Start method of the thread class.
Output:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- Imports System.Threading
- Module Module1
- Private Sub CarA()
- Dim I As Integer
- For I = 0 To 250
- System.Console.Write("Car A" & vbCrLf)
- 'delay of 150 ms
- Thread.CurrentThread.Sleep(150)
- Next
- End Sub
- Sub CarB()
- Dim I As Integer
- For I = 0 To 250
- System.Console.Write("Car B" & vbCrLf)
- 'delay of 250 ms
- Thread.CurrentThread.Sleep(250)
- Next
- End Sub
- Sub CarC()
- Dim I As Integer
- For I = 0 To 250
- System.Console.Write("Car C" & vbCrLf)
- 'delay of 100 ms
- Thread.CurrentThread.Sleep(100)
- Next
- End Sub
- Public Sub Main()
- 'Create threads
- Dim A As System.Threading.Thread = New Threading.Thread(AddressOf CarA)
- Dim B As System.Threading.Thread = New Threading.Thread(AddressOf CarB)
- Dim C As System.Threading.Thread = New Threading.Thread(AddressOf CarC)
- 'create threads
- A.Start()
- B.Start()
- C.Start()
- End Sub
- End Module
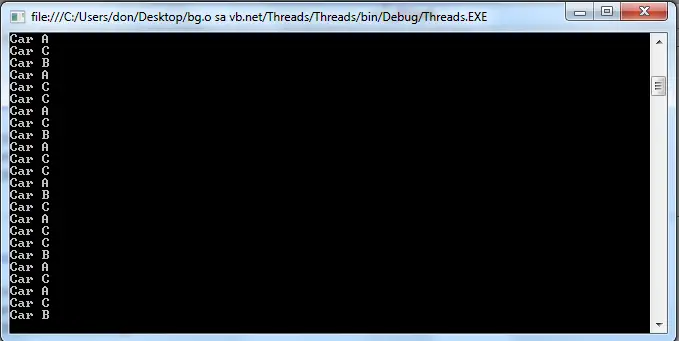