In this tutorial, I will teach you how to
Fill a ComboBox with Data in VB.Net and SQL Server 2019. This method has the ability to
retrieve the data in the sql server database and display it into the combobox. This is simple yet powerful method that you can do it in no time. Follow the step by step guide to know how it works.
I used
Microsoft Visual Studio 2015 and SQL Server 2019 to develop this program.
Let’s Begin:
Creating Database
1. Install the
MSSMS 2019 on your machine.
2. Open the
MSSMS 2019 . After that, right click the database, then select “
New Database” and name it “
dbperson”
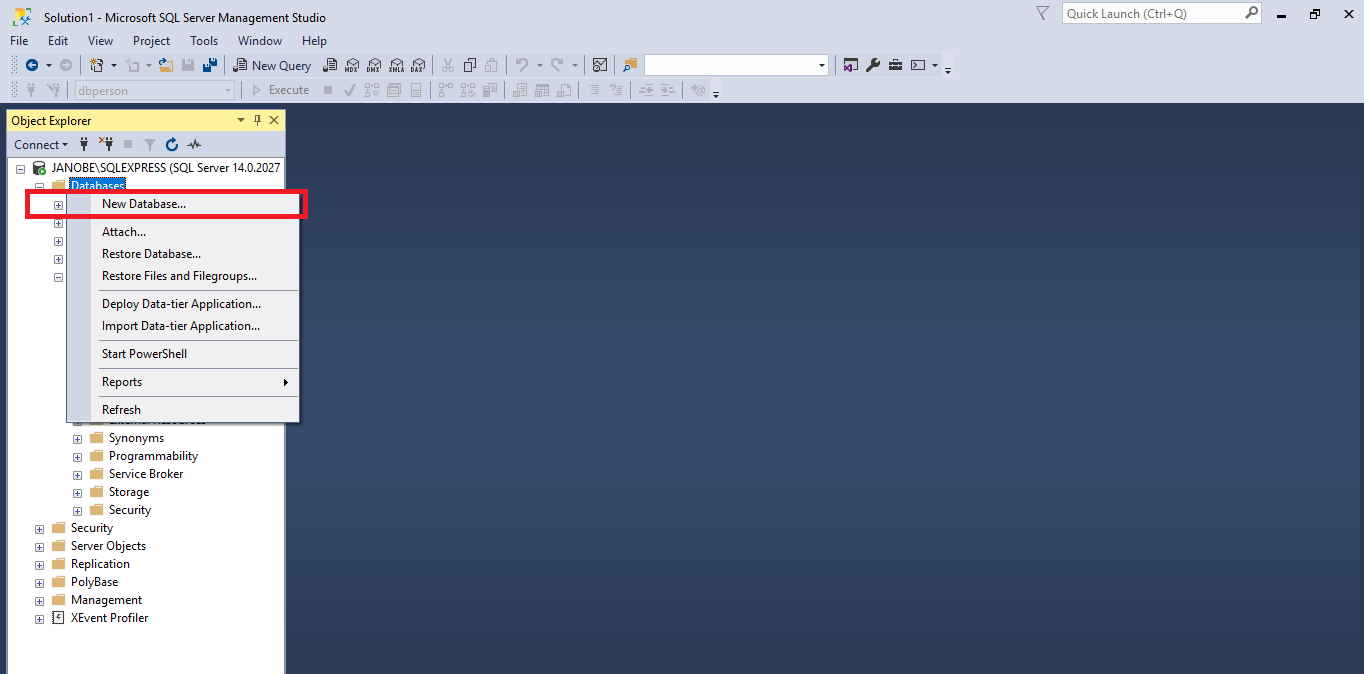
3. Do the following query to create a table in the database that you have created
USE [dbperson]
GO
/****** Object: Table [dbo].[tblperson] Script Date: 11/10/2019 11:15:11 AM ******/
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
CREATE TABLE [dbo].[tblperson](
[PersonID] [INT] IDENTITY(1,1) NOT NULL,
[Fname] [nvarchar](50) NULL,
[Lname] [nvarchar](50) NULL
) ON [PRIMARY]
GO
Creating Application
Step 1
Open
Microsoft Visual Studio 2015 and create a new windows form application for visual basic.
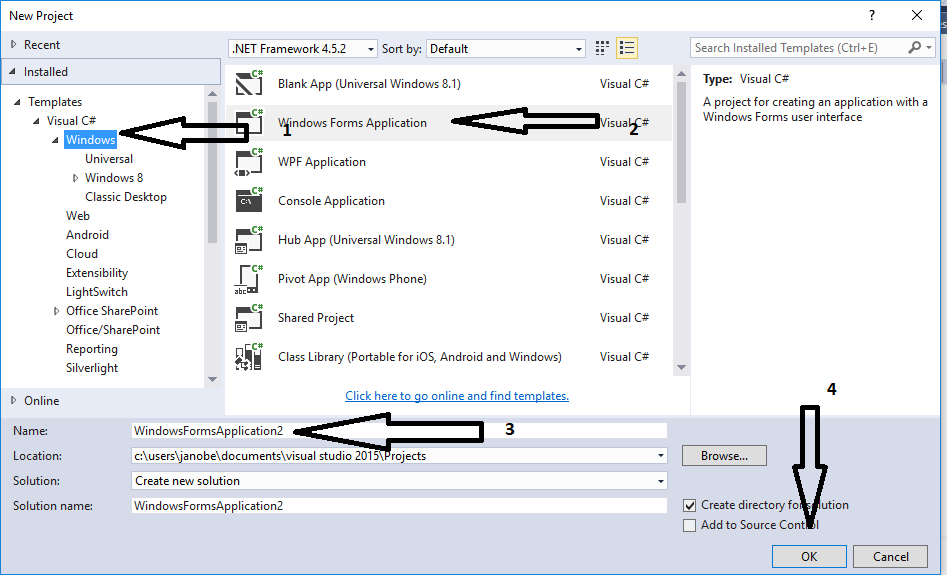
Step 2
Add a
ComboBox inside the Form. Then do the Form just like shown below.
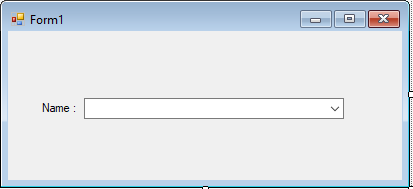
Step 3
Press F7 to open the code editor. In the code editor, add a namespace to access
SQL Server
libraries.
Imports System.Data.SqlClient
Step 4
Create a connection between
Visual Basic 2015 and SQL Server database. After that, declare and initialize all the classes and variables that are needed.
Dim con As SqlConnection = New SqlConnection("Data Source=.\SQLEXPRESS;Database=dbperson;trusted_connection=true;")
Dim cmd As SqlCommand
Dim da As SqlDataAdapter
Dim dt As DataTable
Dim sql As String
Step 5
Create a method for
filling data in the combobox in the database.
Private Sub fillCombo(sql As String, cbo As ComboBox)
Try
con.Open()
cmd = New SqlCommand
da = New SqlDataAdapter
dt = New DataTable
With cmd
.Connection = con
.CommandText = sql
End With
With da
.SelectCommand = cmd
.Fill(dt)
End With
cbo.DataSource = dt
cbo.DisplayMember = dt.Columns(1).ColumnName
cbo.ValueMember = dt.Columns(0).ColumnName
Catch ex As Exception
MsgBox(ex.Message)
Finally
con.Close()
da.Dispose()
End Try
End Sub
Step 6
Write the following codes to fill data in the
combobox in the first load of the form.
Private Sub Form1_Load(sender As Object, e As EventArgs) Handles MyBase.Load
sql = "SELECT * FROM tblperson"
fillCombo(sql, ComboBox1)
End Sub
The complete source code is included. You can download it and run it on your computer.
For any questions about this article. You can contact me @
Email –
[email protected]
Mobile No. – 09305235027 – TNT
Or feel free to comment bel