PHP - Check Email Availability Using jQuery
Submitted by razormist on Friday, July 27, 2018 - 14:52.
In this tutorial we will create a Check Email Availability Using jQuery. By using PHP, you can let your user directly interact with the script and easily to learned its syntax. It is mostly used by a newly coders for its user friendly environment. jQuery is a cross-platform JavaScript library designed to simplify the client-side scripting of HTML. So Let's do the coding...
script.js
Note: To make this script works make sure you already import the jquery plugin to these application.
There you have it we successfully created Check Email Availability Using jQuery. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!!!
Getting Started:
First you have to download & install XAMPP or any local server that run PHP scripts. Here's the link for XAMPP server https://www.apachefriends.org/index.html. And this is the link for the jquery that i used in this tutorial https://jquery.com/. Lastly, this is the link for the bootstrap that i used for the layout design https://getbootstrap.com/.Creating Database
Open your database web server then create a database name in it db_email, after that click Import then locate the database file inside the folder of the application then click ok.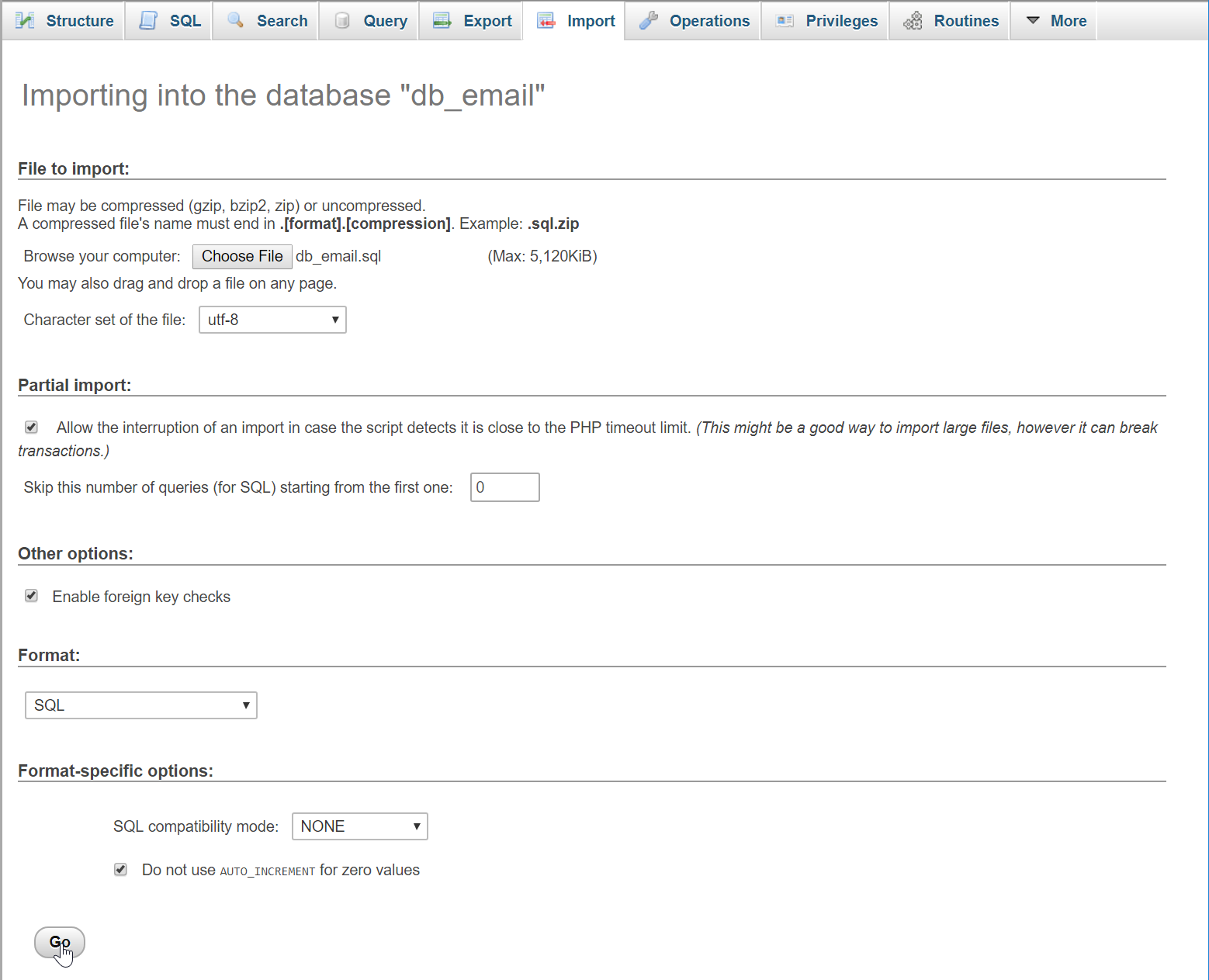
Creating the database connection
Open your any kind of text editor(notepad++, etc..). Then just copy/paste the code below then name it conn.php.- <?php
- $conn = new mysqli('localhost', 'root', '', 'db_email');
- if(!$conn){
- }
- ?>
Creating The Interface
This is where we will create a simple form for our application. To create the forms simply copy and write it into your text editor, then save it as shown below.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - Check Email Availability Using jQuery</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="col-md-2"></div>
- <div class="col-md-6">
- <form>
- <div class="form-group">
- <label>Email</label>
- <input type="text" id="email" class="form-control"/>
- <div id="check_email"></div>
- </div>
- <div class="form-group">
- <label>Password</label>
- <input type="password" id="password" class="form-control"/>
- </div>
- <div class="form-group">
- <label>Firstname</label>
- <input type="text" id="firstname" class="form-control"/>
- </div>
- <div class="form-group">
- <label>Lastname</label>
- <input type="text" id="lastname" class="form-control"/>
- </div>
- <div id="result"></div>
- <div class="form-group">
- <center><button type="button" id="save" class="btn btn-primary"><span class="glyphicon glyphicon-save"></span> Save Data</button></center>
- </div>
- </form>
- </div>
- </div>
- </body>
- <script src="js/jquery-3.2.1.min.js"></script>
- <script src="js/script.js"></script>
- </html>
Creating Save Query
This code contains the saving query for the application. This will store the data inputs to the database server after completing all the required fields. To create this just write this code inside the text editor then save it as save_query.php.- <?php
- require_once 'conn.php';
- $password = $_POST['password'];
- $firstname = $_POST['firstname'];
- $lastname = $_POST['lastname'];
- $conn->query("INSERT INTO `member` VALUES('', '$email', '$password', '$firstname', '$lastname')");
- ?>
Creating Email Validation
This code contains the main function of the application. This will validate every input of the user in the text box to check if is available to use. To make these just kindly follow and write these block of codes inside the text editor then is show below. validation.php- <?php
- require_once 'conn.php';
- $email = $_POST['email'];
- echo "error";
- }else{
- $query = $conn->query("SELECT * FROM `member` WHERE `email` = '$email'");
- $rows = $query->num_rows;
- if($rows > 0){
- echo "invalid";
- }else{
- echo "success";
- }
- }
- ?>
- $(document).ready(function(){
- var email_status;
- $('#email').on('keyup', function(){
- $.post(
- 'validation.php',
- {email: $(this).val()},
- function(data){
- if(data == "success"){
- $('#check_email').html("<label class='text-success'>Email is valid</label>")
- email_status = true;
- }else if(data == "error"){
- $('#check_email').html("<label class='text-danger'>This is not a valid email</label>")
- email_status = false;
- }else if(data == "invalid"){
- $('#check_email').html("<label class='text-danger'>Email is already taken!</label>")
- email_status = false;
- }
- });
- });
- $('#save').on('click', function(){
- var email = $('#email').val();
- var password = $('#password').val();
- var firstname = $('#firstname').val();
- var lastname = $('#lastname').val();
- if(email_status === false || password == "" || firstname == "" || lastname == ""){
- $('#result').html("<center><label class='text-warning'>Please fill up the required field!</label></center>")
- }else{
- $.post(
- 'save_query.php',
- {email: email, password: password, firstname: firstname, lastname: lastname},
- function(){
- $('#email').val('');
- $('#password').val('');
- $('#firstname').val('');
- $('#latname').val('')
- $('#result').html("<center><label class='text-success'>Data Inserted</label></center>")
- });
- }
- });
- });