How to Pretty Print PHP Array
Submitted by nurhodelta_17 on Thursday, April 19, 2018 - 23:18.
Creating our PHP Array
First, we are going to create a sample PHP array.Printing the Array
Then, we use print_r() function to view item in our array.- <?php
- ?>
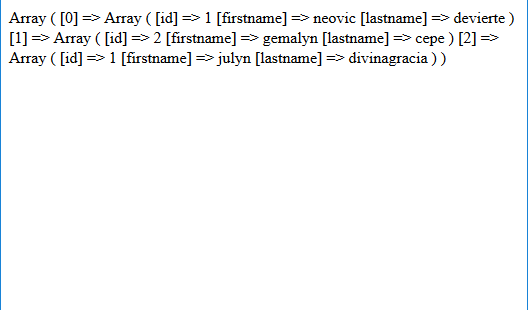
Prettifying our Array
Finally, we prettify the display of our array by creating a function.- <?php
- function prettyPrint($array) {
- }
- echo prettyPrint($array);
- ?>
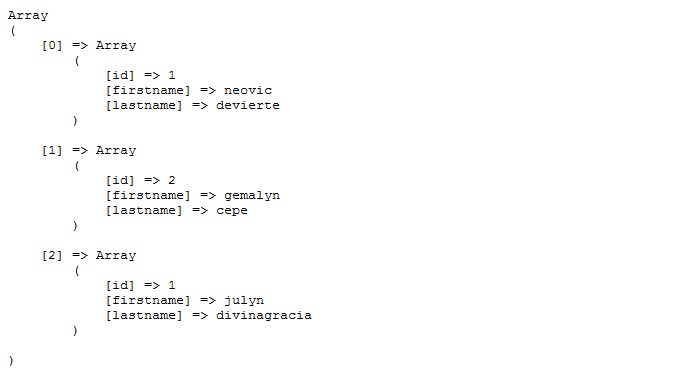
Full Code
Here's the full code.- <?php
- //create example array
- 'id' => '1',
- 'firstname' => 'neovic',
- 'lastname' => 'devierte'
- ),
- 'id' => '2',
- 'firstname' => 'gemalyn',
- 'lastname' => 'cepe'
- ),
- 'id' => '1',
- 'firstname' => 'julyn',
- 'lastname' => 'divinagracia'
- )
- );
- //creating prettify function
- function prettyPrint($array) {
- }
- //displaying pretty array
- echo prettyPrint($array);
- ?>