Total Sum Of Products In PHP/MySQL
Submitted by rinvizle on Thursday, November 24, 2016 - 14:10.
Total Sum Of Products System is a simple project created in PHP, MySQL Javascript, and it has a extension of Bootstrap and Modal for the better and responsive design for all the users. This simple system is very useful for the projects and also for the other company that manages products. This system creates a total sum or cost of all products where automatically gives you the amount under the product table.
Table_member - And this script is for the table of products where it displays the details.
Table_user.php - This script also is for creating a new product.
Hope that you learn in this tutorial. And for more updates and programming tutorials don't hesitate to ask and we will answer your questions and suggestions. Don't forget to LIKE & SHARE this website.
Sample Code
Home.php - this is where the two forms combined and synchronized to a one form of code.- <?php
- include('header.php');
- ?>
- <!DOCTYPE html>
- <html>
- <head>
- <title>Total Sum Of Products</title>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <div class="navbar-header">
- <a class="navbar-brand" href="https://www.sourcecodester.com">Sourcecodester</a>
- </div>
- <ul class="nav navbar-nav">
- <li class="active"><a href="home.php">Home</a></li>
- <li><a href="#">About</a></li>
- <li><a href="#">Contact Us</a></li>
- </ul>
- </div>
- </nav>
- <h1 align="center">Products</h1>
- <div class="container">
- <div class="row-fluid">
- <div class="span12">
- <ul id="myTab" class="nav nav-tabs">
- <li class="active"><a href="#member" data-toggle="tab"><i class="icon-group icon-large"></i> Total Product List</a></li>
- <li><a href="#user" data-toggle="tab"><i class="icon-user icon-large"></i> Add Product</a></li>
- <li>
- <a data-toggle="modal" data-target="#myModal"><i class="icon-signout icon-large"></i> Logout</a>
- </li>
- </ul>
- </div>
- </div>
- <div class="tab-content">
- <?php
- include('tab_member.php');
- ?>
- <?php
- include('tab_user.php');
- ?>
- </div>
- <?php
- include('modal.php');
- ?>
- </div>
- </body>
- </html>
- <div class="tab-pane fade in active" id="member">
- <table cellpadding="0" cellspacing="0" border="0" class="table table-striped table-bordered" id="example">
- <thead>
- <tr>
- <th>Product Name</th>
- <th>Quantity</th>
- <th>Price</th>
- <th>Amount</th>
- <th>Delete</th>
- </tr>
- </thead>
- <tbody>
- <?php
- $id=$row['product_id'];
- ?>
- <tr>
- <td><?php echo $row['name'] ?></td>
- <td><?php echo $row['qty'] ?></td>
- <td><?php echo $row['price'] ?></td>
- <td><?php echo $row['amount']; ?></td>
- <td><a id="<?php echo $id;?>" class="delete_product"> Delete</a></td>
- </tr>
- <?php } ?>
- </tbody>
- </table>
- <?php
- ?>
- <div class="pull-right">
- <div class="span"><div class="alert alert-success"><i class="icon-credit-card icon-large"></i> Total: <?php echo $rows['sum(amount)']; ?></div></div>
- </div>
- <?php }
- ?>
- <?php
- ?>
- <div class="pull-right">
- <div class="span"><div class="alert alert-info"><i class="icon-credit-card icon-large"></i> Total number of Items: <?php echo $rows1['sum(qty)']; ?></div></div>
- </div>
- <?php }
- ?>
- <script type="text/javascript">
- $(document).ready( function() {
- $('.delete_product').click( function() {
- var id = $(this).attr("id");
- if(confirm("Are you sure you want to delete this Product?")){
- $.ajax({
- type: "POST",
- url: "delete.php",
- data: ({id: id}),
- cache: false,
- success: function(html){
- $(".del"+id).fadeOut('slow');
- }
- });
- }else{
- return false;}
- });
- });
- </script>
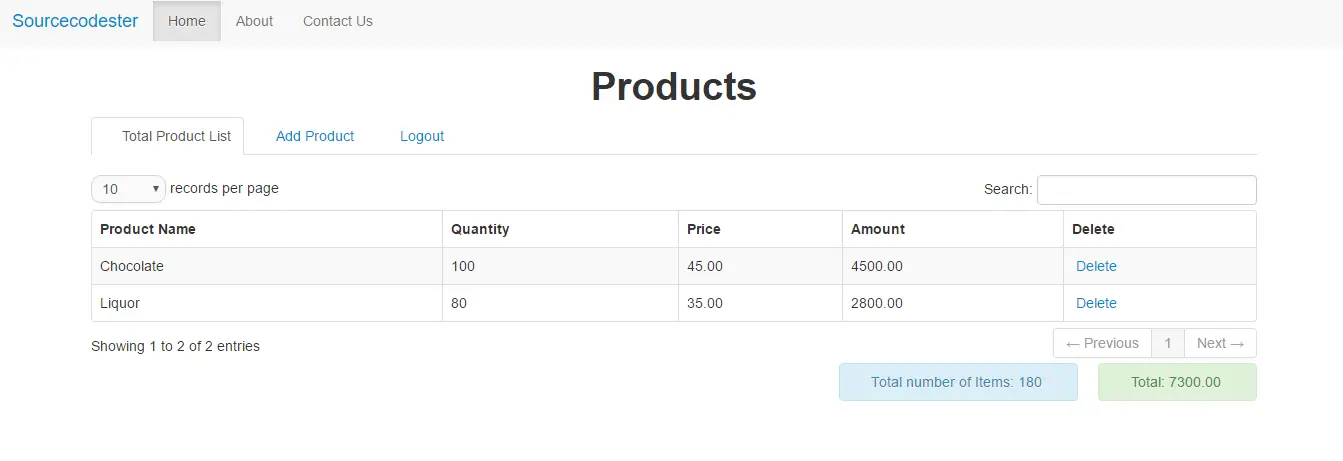
- <div class="tab-pane fade"id="user">
- <div class="containers">
- <p align="center" style="color:#000">Fill Up All The Details Below!</p>
- <form class="form-horizontal" method="POST">
- <div class="form-group">
- <label class="control-label col-sm-2" for="name">Product Name:</label>
- <div class="span4">
- <input type="text" name="name" class="form-control" id="name" placeholder="Product Name" required>
- </div>
- </div>
- <div class="form-group">
- <label class="control-label col-sm-2" for="qty">Quantity:</label>
- <div class="span4">
- <input type="text" name="qty" class="form-control" id="qty" placeholder="Quantity" required>
- </div>
- </div>
- <div class="form-group">
- <label class="control-label col-sm-2" for="price">Price:</label>
- <div class="span4">
- <input type="text" name="price" class="form-control" id="price" placeholder="Price" required>
- </div>
- </div>
- <div class="form-group">
- <div class="col-sm-offset-2 col-sm-10">
- <button type="submit" name="submit" class="btn btn-primary">Submit</button>
- </div>
- </div>
- </form>
- <?php
- $name=$_POST['name'];
- $qty=$_POST['qty'];
- $price=$_POST['price'];
- $amount=$qty * $price;
- ?>
- <script type="text/javascript">
- alert('Successfully Added a New Product');
- window.location="../admin/home.php";
- </script>
- <?php
- }
- ?>
- </div>
- </div>