Sign Up Form Using Bootstrap with PHP Function Using PDO
Submitted by alpha_luna on Friday, May 27, 2016 - 14:36.
Sign Up Form Using Bootstrap with PHP Function Using PDO
In this article, we are going to learn on how to create sign up form using bootstrap with PHP function using PDO. We are going to use bootstrap for the input field where the user enters their information to sign up and PHP using PDO for the function.Creating our Table
We are going to make our database.- Open the PHPMyAdmin.
- Create a database and name it as "bootstrap_signup".
- After creating a database name, then we are going to create our table. And name it as "user_account".
- Kindly copy the code below.
- CREATE TABLE `user_account` (
- `user_account_id` INT(11) NOT NULL,
- `user_name` VARCHAR(100) NOT NULL,
- `email` VARCHAR(100) NOT NULL,
- `password` VARCHAR(100) NOT NULL,
- `confirm_password` VARCHAR(100) NOT NULL
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
Sign Up Form
- <!-- form start -->
- <form method="post" action="add.php">
- <div class="body_style">
- <div class="form-group">
- <div class="input-group">
- <input name="user_name" type="text" class="form-control" autofocus="autofocus" placeholder="User Name ....." style="border:1px solid blue;">
- </div>
- </div>
- <div class="form-group">
- <div class="input-group">
- <input name="email" type="text" class="form-control" placeholder="Email ....." style="border:1px solid blue;">
- </div>
- </div>
- <div class="row">
- <div class="form-group col-lg-6">
- <div class="input-group">
- <input name="password" id="password" type="password" class="form-control" placeholder="Password ....." style="border:1px solid blue;">
- </div>
- </div>
- <div class="form-group col-lg-6">
- <div class="input-group">
- <input name="confirm_password" type="password" class="form-control" placeholder="Re-type Password ....." style="border:1px solid blue;">
- </div>
- </div>
- </div>
- </div>
- <div class="footer_style">
- <button type="submit" name="submit" class="btn btn-success" style="border:1px solid blue;">
- </button>
- </div>
- </form>
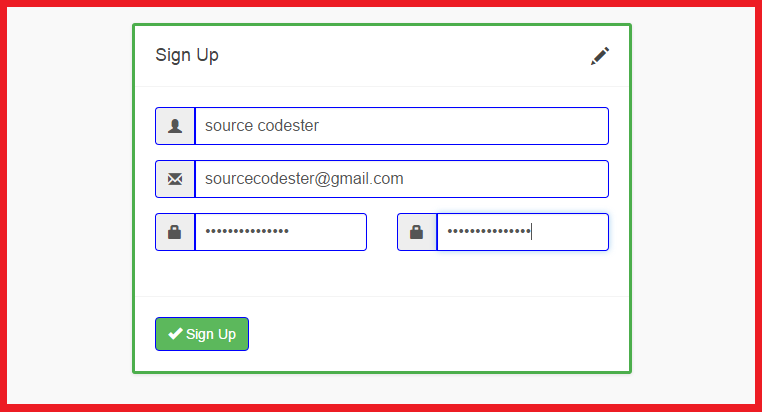
PHP Function Using PDO
- <?php
- include ('database.php');
- $user_name=$_POST['user_name'];
- $email=$_POST['email'];
- $password=$_POST['password'];
- $confirm_password=$_POST['confirm_password'];
- $conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
- $sql = "INSERT INTO user_account (user_name, email, password, confirm_password)
- VALUES ('$user_name', '$email', '$password', '$confirm_password')";
- echo "<script>alert('Successfully Added!'); window.location='view.php'</script>";
- ?>
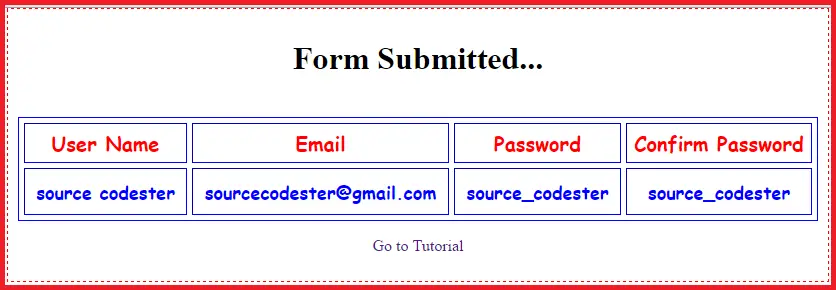