Bootstrap Navigation Bar With Multi-Level Dropdowns
Submitted by rinvizle on Monday, August 8, 2016 - 10:11.
This tutorial we will teach you how to construct or create a responsive Bootstrap Navigation Bar With Multi-Level Dropdowns. With Bootstrap, a navigation bar can extend or collapse, depending on the screen size. A standard navigation bar is created with a class called "navbar navbar-default". Navigation bars can also hold multiple dropdown menus. And it has a simple HTML page or Web Template by using bootstrap.
And for the content of the web page or the html form. This content has a glyphicon effects using a simple script of javascript to make it more responsive.
Sample Code
Index.php - This is the code for a fix navigation bar and the for the multi-level dropdown.- <div class="container">
- <nav class="navbar navbar-default navbar-fixed-top">
- <div class="container-fluid">
- <div class="navbar-header">
- <a class="navbar-brand" href="#">Sourcecodester</a>
- </div>
- <ul class="nav navbar-nav">
- <li class="active"><a href="#"><span class="glyphicon glyphicon-home"></span></a></li>
- <li><a href="#">HTML</a></li>
- <li><a href="#">CSS</a></li>
- <li><a href="#">JAVASCRIPT</a></li>
- <li><a href="#">PHP</a></li>
- <li><a href="#">SQL</a></li>
- <li class="dropdown">
- <a class="dropdown-toggle" data-toggle="dropdown" href="#">TUTORIAL<span class="caret"></span></a>
- <ul class="dropdown-menu">
- <li><a href="#">HTML/CSS</a></li>
- <li><a href="#">JAVASCRIPT</a></li>
- <li><a href="#">PHP</a></li>
- <li>
- <a class="test" tabindex="-1" href="#">SERVER SIDE <span class="caret"></span></a>
- <ul class="dropdown-menu">
- <li><a tabindex="-1" href="#">SQL</a></li>
- <li><a tabindex="-1" href="#">ASP.NET</a></li>
- <li>
- <a class="test" href="#">WEB BUILDING <span class="caret"></span></a>
- <ul class="dropdown-menu">
- <li><a href="#">WEB TEMPLATES</a></li>
- <li><a href="#">WEB STATISTICS</a></li>
- </ul>
- </li>
- </ul>
- </li>
- </ul>
- </li>
- </ul>
- <ul class="nav navbar-nav navbar-right">
- <li><a href="#"><span class="glyphicon glyphicon-user"></span> Sign Up</a></li>
- <li><a href="#"><span class="glyphicon glyphicon-search"></span></a></li>
- </ul>
- </div>
- </nav>
- </div>
For the dropdown menu script to create a multi-level dropdown list.
- <script>
- $(document).ready(function(){
- $('.dropdown a.test').on("click", function(e){
- $(this).next('ul').toggle();
- e.stopPropagation();
- e.preventDefault();
- });
- });
- </script>
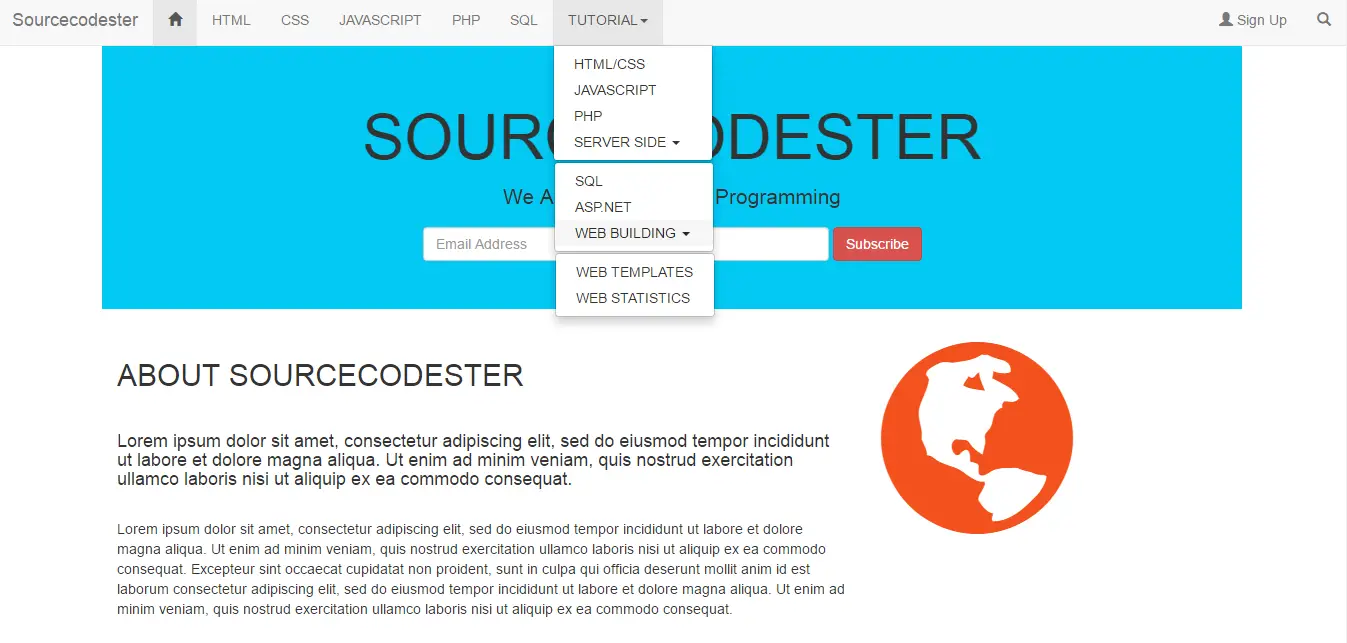
And for the content of the web page or the html form. This content has a glyphicon effects using a simple script of javascript to make it more responsive.
- <div class="content">
- <div class="jumbotron text-center">
- <h1>SOURCECODESTER</h1>
- <p>We Are Specialized In Programming</p>
- <form class="form-inline">
- <input type="email" class="form-control" size="50" placeholder="Email Address" required>
- <button type="button" class="btn btn-danger">Subscribe</button>
- </form>
- </div>
- <div id="about" class="container-fluid">
- <div class="row">
- <div class="col-sm-8">
- <h2>About Sourcecodester</h2><br>
- <h4>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.</h4><br>
- <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.</p>
- </div>
- <div class="col-sm-4">
- <span class="glyphicon glyphicon-globe logo slideanim"></span>
- </div>
- </div>
- </div>
-
-
- <div id="services" class="container-fluid text-center">
- <h2>SERVICES</h2>
- <h4>What we offer</h4>
- <br>
- <div class="row slideanim">
- <div class="col-sm-4">
- <span class="glyphicon glyphicon-off logo-small"></span>
- <h4>POWER</h4>
- </div>
- <div class="col-sm-4">
- <span class="glyphicon glyphicon-heart logo-small"></span>
- <h4>LOVE</h4>
- </div>
- <div class="col-sm-4">
- <span class="glyphicon glyphicon-lock logo-small"></span>
- <h4>JOB DONE</h4>
- </div>
- </div>
- <div class="col-sm-4">
- <span class="glyphicon glyphicon-leaf logo-small"></span>
- <h4>GREEN</h4>
- </div>
- <div class="col-sm-4">
- <span class="glyphicon glyphicon-certificate logo-small"></span>
- <h4>CERTIFIED</h4>
- </div>
- <div class="col-sm-4">
- <span class="glyphicon glyphicon-wrench logo-small"></span>
- <h4 style="color:#303030;">HARD WORK</h4>
- </div>
- </div>
- </div>
-
- <footer class="container-fluid text-center"><hr/>
- <p><a href="http://www.sourcecodester.com" title="">www.sourcecodester.com</a></p>
- </footer>
-
- <script>
- $(document).ready(function(){
- $(".navbar a, footer a[href='#myPage']").on('click', function(event) {
- event.preventDefault();
- $('html, body').animate({
- }, 900, function(){
- });
- }
- });
-
- $(window).scroll(function() {
-
- var winTop = $(window).scrollTop();
- $(this).addClass("slide");
- }
- });
- });
- })
- </script>
- </div>
- </body>
- </html>
Hope that you like my tutorial. And don't forget to Like and Share this to your friends. Enjoy Coding.
- <div class="content">
- <div class="jumbotron text-center">
- <h1>SOURCECODESTER</h1>
- <p>We Are Specialized In Programming</p>
- <form class="form-inline">
- <input type="email" class="form-control" size="50" placeholder="Email Address" required>
- <button type="button" class="btn btn-danger">Subscribe</button>
- </form>
- </div>
- <div id="about" class="container-fluid">
- <div class="row">
- <div class="col-sm-8">
- <h2>About Sourcecodester</h2><br>
- <h4>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.</h4><br>
- <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.</p>
- </div>
- <div class="col-sm-4">
- <span class="glyphicon glyphicon-globe logo slideanim"></span>
- </div>
- </div>
- </div>
- <div id="services" class="container-fluid text-center">
- <h2>SERVICES</h2>
- <h4>What we offer</h4>
- <br>
- <div class="row slideanim">
- <div class="col-sm-4">
- <span class="glyphicon glyphicon-off logo-small"></span>
- <h4>POWER</h4>
- </div>
- <div class="col-sm-4">
- <span class="glyphicon glyphicon-heart logo-small"></span>
- <h4>LOVE</h4>
- </div>
- <div class="col-sm-4">
- <span class="glyphicon glyphicon-lock logo-small"></span>
- <h4>JOB DONE</h4>
- </div>
- </div>
- <div class="col-sm-4">
- <span class="glyphicon glyphicon-leaf logo-small"></span>
- <h4>GREEN</h4>
- </div>
- <div class="col-sm-4">
- <span class="glyphicon glyphicon-certificate logo-small"></span>
- <h4>CERTIFIED</h4>
- </div>
- <div class="col-sm-4">
- <span class="glyphicon glyphicon-wrench logo-small"></span>
- <h4 style="color:#303030;">HARD WORK</h4>
- </div>
- </div>
- </div>
- <footer class="container-fluid text-center"><hr/>
- <p><a href="http://www.sourcecodester.com" title="">www.sourcecodester.com</a></p>
- </footer>
- <script>
- $(document).ready(function(){
- $(".navbar a, footer a[href='#myPage']").on('click', function(event) {
- event.preventDefault();
- $('html, body').animate({
- }, 900, function(){
- });
- }
- });
- $(window).scroll(function() {
- var winTop = $(window).scrollTop();
- $(this).addClass("slide");
- }
- });
- });
- })
- </script>
- </div>
- </body>
- </html>