Secure Login and Registration
Submitted by rinvizle on Friday, August 5, 2016 - 14:12.
This tutorial we will teach you on how to build a Secure Login and Registration system whereby users of your websites will be able to create their accounts, log in with their valid information so as to access their various accounts. Users information during sign up process are validated and stored in the database. The system validates new users for duplicate email addresses and performs valid authentication during registered users log in. And this is compose of PHP and MySql, this programs are written in a way that any one can understand and customize.
signup.php - Signup.php is for creating a new account and storing the data in the database. Every account that the user created their passwords will automaticall encrypted using the MD5.
Hope that you learn in this tutorial and enjoy coding. Don't forget to LIKE & SHARE this website.
Sample Code
login.php - This is for the login activity of every users, and it has a script that gets a data in the database so the can user can login to their account.- <?php
- include "database_connection.php";
- {
- $validate_user_information = mysql_query("select * from `signup_and_login_table` where `email` = '".mysql_real_escape_string($user_email)."' and `password` = '".mysql_real_escape_string($encrypted_md5_password)."'");
- if($user_email == "" || $user_password == "")
- {
- $error = '<br><div class="info">Sorry, all fields are required to log into your account. Thanks.</div><br>';
- }
- {
- $_SESSION["VALID_USER_ID"] = $user_email;
- $_SESSION["USER_FULLNAME"] = strip_tags($get_user_information["firstname"].' '.$get_user_information["lastname"]);
- }
- else
- {
- $error = '<br><div class="info">Sorry, you have provided incorrect information. Please enter correct user information to proceed. Thanks.</div><br>';
- }
- }
- ?>
- <!DOCTYPE html>
- <html>
- <head>
- <title>Secure Login and Registration</title>
- <link href="css/style.css" rel="stylesheet" type="text/css">
- </head>
- <body>
- <center>
- <br /><br /><br /><br /><div style="font-family:Verdana, Geneva, sans-serif; font-size:24px;"><h3>Secure Login and Registration</h3></div>
- <center>
- <div class="main_wrapper">
- <br clear="all">
- <form method="post" action="<?php echo $_SERVER['PHP_SELF']; ?>">
- <h2 align="center" style="margin-top:0px;">User Login</h2><hr/><br/>
- <div style="width:115px;float:left;" align="left"><h3>Email Address:</h3></div>
- <div style="width:300px;float:left;" align="left"><input type="text" name="email" id="email" value="" class="textAreaBoxInputs" placeholder="Email Address"></div><br clear="all"><br clear="all">
- <div style="width:115px;float:left;" align="left"><h3>Password:</h3></div>
- <div style="width:300px;float:left;" align="left"><input type="password" name="passwd" id="passwd" value="" class="textAreaBoxInputs" placeholder="Password"></div><br clear="all"><br clear="all">
- <div style="width:115px; padding-top:10px;float:left;" align="left"> </div>
- <div style="width:300px;float:left;" align="left">
- <input type="hidden" name="submitted" id="submitted" value="yes">
- <input type="submit" name="submit" id="" value="Login" style="margin-right:50px;" class="general_button">
- <a href="signup.php" style="text-decoration:none;" class="general_button">Register</a>
- </div>
- </form>
- <br clear="all">
- <br clear="all"><br clear="all">
- <div style="width:250px; font-family:Verdana, Geneva, sans-serif; font-size:11px;" align="left">
- <b>Account To Login For A Demonstration</b><br>
- Email Address: <i>[email protected]</i><br>
- Demo Password: <i>source123</i>
- </div>
- </div>
- </center>
- </center>
- </body>
- </html>
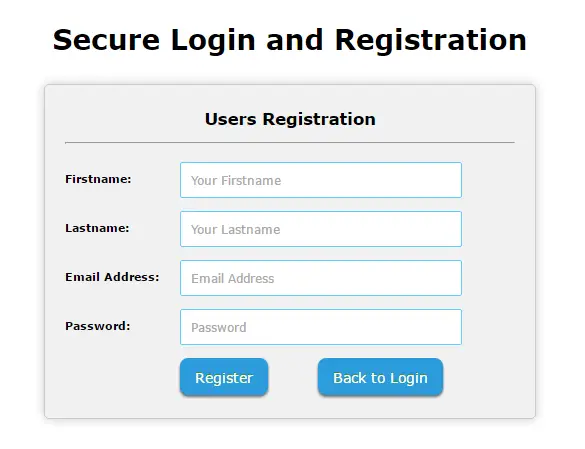
- <?php
- include "database_connection.php";
- {
- $check_for_duplicates = mysql_query("select * from `signup_and_login_table` where `email` = '".mysql_real_escape_string($user_email)."'");
- if($firstname == "" || $lastname == "" || $user_email == "" || $user_password == "")
- {
- $error = '<br><div class="info">Sorry, all fields are required to create a new account. Thanks.</div><br>';
- }
- elseif(!eregi("^[_a-z0-9-]+(\.[_a-z0-9-]+)*@[a-z0-9-]+(\.[a-z0-9-]+)*(\.[a-z]{2,3})$", $user_email))
- {
- $error = '<br><div class="info">Sorry, Your email address is invalid, please enter a valid email address to proceed. Thanks.</div><br>';
- }
- {
- $error = '<br><div class="info">Sorry, your email address already exist in our database and duplicate email addresses are not allowed for security reasons.<br>Please enter a different email address to proceed or login with your existing account. Thanks.</div><br>';
- }
- else
- {
- if(mysql_query("insert into `signup_and_login_table` values('', '".mysql_real_escape_string($firstname)."', '".mysql_real_escape_string($lastname)."', '".mysql_real_escape_string($user_email)."', '".mysql_real_escape_string($encrypted_md5_password)."', '".mysql_real_escape_string(date('d-m-Y'))."')"))
- {
- $_SESSION["VALID_USER_ID"] = $user_email;
- }
- else
- {
- $error = '<br><div class="info">Sorry, your account could not be created at the moment. Please try again or contact the site admin to report this error if the problem persist. Thanks.</div><br>';
- }
- }
- }
- ?>
- <!DOCTYPE html>
- <html>
- <head>
- <title>Secure Login and Registration</title>
- <link href="css/style.css" rel="stylesheet" type="text/css">
- </head>
- <body>
- <center>
- <br /><br /><br /><div style="font-family:Verdana, Geneva, sans-serif; font-size:24px;"><h3>Secure Login and Registration</h3></div>
- <center>
- <div class="main_wrapper">
- <br clear="all">
- <form method="post" action="<?php echo $_SERVER['PHP_SELF']; ?>">
- <h2 align="center" style="margin-top:0px;">Users Registration</h2><hr/><br />
- <div style="width:115px; padding-top:10px;float:left;" align="left"><b>Firstname:</b></div>
- <div style="width:300px;float:left;" align="left"><input type="text" name="firstname" id="firstname" value="" class="textAreaBoxInputs" placeholder="Your Firstname"></div><br clear="all"><br clear="all">
- <div style="width:115px; padding-top:10px;float:left;" align="left"><b>Lastname:</b></div>
- <div style="width:300px;float:left;" align="left"><input type="text" name="lastname" id="lastname" value="" class="textAreaBoxInputs" placeholder="Your Lastname"></div><br clear="all"><br clear="all">
- <div style="width:115px; padding-top:10px;float:left;" align="left"><b>Email Address:</b></div>
- <div style="width:300px;float:left;" align="left"><input type="text" name="email" id="email" value="" class="textAreaBoxInputs" placeholder="Email Address"></div><br clear="all"><br clear="all">
- <div style="width:115px; padding-top:10px;float:left;" align="left"><b>Password:</b></div>
- <div style="width:300px;float:left;" align="left"><input type="password" name="passwd" id="passwd" value="" class="textAreaBoxInputs" placeholder="Password"></div><br clear="all"><br clear="all">
- <div style="width:115px; padding-top:10px;float:left;" align="left"> </div>
- <div style="width:300px;float:left;" align="left">
- <input type="hidden" name="submitted" id="submitted" value="yes">
- <input type="submit" name="submit" id="" value="Register" style="margin-right:50px;" class="general_button">
- <a href="login.php" style="text-decoration:none;" class="general_button">Back to Login</a>
- </div>
- </form>
- <br clear="all"><br clear="all">
- </div>
- </center>
- </center>
- </body>
- </html>