Digital Clock using jQuery
Submitted by alpha_luna on Thursday, July 28, 2016 - 15:46.
In this article, we are going to create Digital Clock using jQuery. You can use this simple program to your thesis projects or any kind of systems that you've created. Kindly download the full source code below.
Kindly click the "Download Code" button below for full source code. Thank you very much. If you are interested in programming, we have an example of programs that may help you even just in small ways. Share us your thoughts and comments below. Thank you so much for dropping by and reading this tutorial post. For more updates, don’t hesitate and feel free to visit this website more often and please share this with your friends or email me at [email protected]. Practice Coding. Thank you very much.
You will Learn:
- You can learn to construct simple markup using HTML.
- You can learn to create stylish Digital Clock.
- You can learn to build Digital Clock using jQuery.
Creating Markup
Creating simple HTML source code to display our Digital Clock. Kindly copy and paste this short source code to your BODY tag of your page.Constructing CSS Style
This style where you can edit your Digital Clock design. After downloading the source code below, try to edit the design more attractive to the user. Enjoy coding.- body{
- background:#f5f5f5;
- font:bold 12px Arial, Helvetica, sans-serif;
- margin:0;
- padding:0;
- min-width:960px;
- color:#000;
- }
- a {
- text-decoration:none;
- color:darkblue;
- }
- h1 {
- font: 4em normal Arial, Helvetica, sans-serif;
- padding: 20px; margin: 0;
- text-align:center;
- }
- h1 small{
- font: 0.2em normal Arial, Helvetica, sans-serif;
- text-transform:uppercase; letter-spacing: 0.2em; line-height: 5em;
- display: block;
- }
- h2 {
- font-weight:700;
- color:#bbb;
- font-size:20px;
- }
- h2, p {
- margin-bottom:10px;
- }
jQuery Script
This script that we are going to construct helps us to display a Digital Clock in our page. We have the list of months and name of the day. Copy and paste this script to your HEAD tag of your page.- <script type="text/javascript">
- $(document).ready(function() {
- // Create two variable with the names of the months and days in an array
- var monthNames = [ "January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December" ];
- var dayNames= ["Sunday","Monday","Tuesday","Wednesday","Thursday","Friday","Saturday"]
- // Create a newDate() object
- var newDate = new Date();
- // Extract the current date from Date object
- newDate.setDate(newDate.getDate());
- // Output the day, date, month and year
- $('#Date').html(dayNames[newDate.getDay()] + " " + newDate.getDate() + ' ' + monthNames[newDate.getMonth()] + ' ' + newDate.getFullYear());
- setInterval( function() {
- // Create a newDate() object and extract the seconds of the current time on the visitor's
- var seconds = new Date().getSeconds();
- // Add a leading zero to seconds value
- $("#sec").html(( seconds < 10 ? "0" : "" ) + seconds);
- },1000);
- setInterval( function() {
- // Create a newDate() object and extract the minutes of the current time on the visitor's
- var minutes = new Date().getMinutes();
- // Add a leading zero to the minutes value
- $("#min").html(( minutes < 10 ? "0" : "" ) + minutes);
- },1000);
- setInterval( function() {
- // Create a newDate() object and extract the hours of the current time on the visitor's
- var hours = new Date().getHours();
- // Add a leading zero to the hours value
- $("#hours").html(( hours < 10 ? "0" : "" ) + hours);
- }, 1000);
- });
- </script>
Output
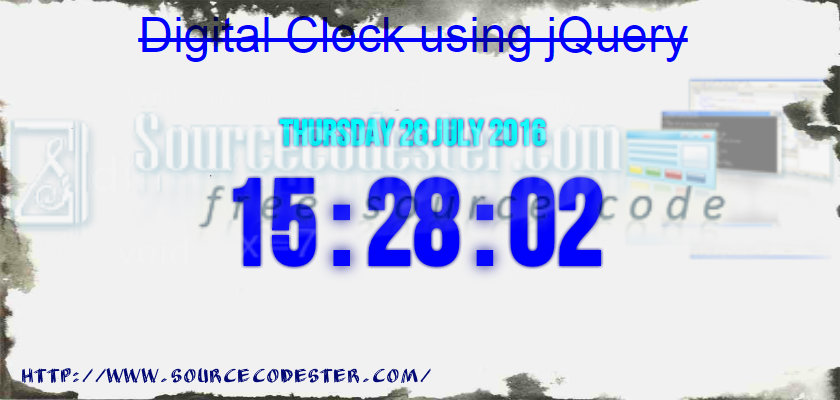
Kindly click the "Download Code" button below for full source code. Thank you very much. If you are interested in programming, we have an example of programs that may help you even just in small ways. Share us your thoughts and comments below. Thank you so much for dropping by and reading this tutorial post. For more updates, don’t hesitate and feel free to visit this website more often and please share this with your friends or email me at [email protected]. Practice Coding. Thank you very much.