How To Make Counter For Numbers, Vowels, And Consonants Using JavaScript
Submitted by alpha_luna on Monday, April 25, 2016 - 12:16.
This program that I make using JavaScript Programming Language is How To Make Counter For Numbers, Vowels, And Consonants Using JavaScript. This program will ask the user to type their sentence on the Textarea of Form Field then the program will calculate the number of vowels, consonants, and numbers.
This program is very simple and you can create after downloading the source code below as your own. Hope you find this useful.
HTML Source Code
This code contains Textarea for the user to type their sentence.
JavaScript Source Code
This script will help us to calculate the number of vowels, consonants, and numbers.
And, the style is.
This is the Output:
Share us your thoughts and comments below. Thank you so much for dropping by and reading this tutorial post. For more updates, don’t hesitate and feel free to visit this website more often and please share this with your friends or email me at [email protected]. Practice Coding. Thank you very much.
- <div class="wrapper">
- <table cellspacing="5" cellpadding="5" width="100%" style="border:red 1px solid;">
- <tr>
- </tr>
- <tr>
- </tr>
- <tr>
- </tr>
- <tr>
- </tr>
- <tr>
- </tr>
- <tr>
- </tr>
- <tr>
- </tr>
- <tr>
- </tr>
- <tr>
- <td colspan="2">
- <input type='button' value='Enter' class="btn_enter" title="Click here to process." onclick="javascript:all_count();" />
- <input type='button' value='Clear' class="btn_cancel" title="Click here to clear all the text fields." onclick="javascript:reset_all();" />
- </td>
- </tr>
- </table>
- </div>
- <script type="text/javascript">
- function all_count() {
- var str = document.getElementById('sentence_counter').value;
- var count = 0, total_vowels="";
- var count2=0, consonants_lists="";
- var count3=0, total_digits="";
- for (var i = 0; i < str.length; i++) {
- if (str.charAt(i).match(/[a-zA-Z]/) != null) {
- if (str.charAt(i).match(/[aeiouAEIOU]/))
- {
- total_vowels = total_vowels + str.charAt(i);
- count++;
- }
- if (str.charAt(i).match(/[bcdfghjklmnpqrstvwxyzBCDFGHJKLMNPQRSTVWXYZ]/))
- {
- consonants_lists = consonants_lists + str.charAt(i);
- count2++;
- }
- }
- function retnum(str1) {
- var num = str1.replace(/[^0-9]/g, '');
- return num;
- }
- function count_digits(str2) {
- var num2 = str2.replace(/[^0-9]/g,"").length;
- return num2;
- }
- }
- document.getElementById('all_consonants').value = count2;
- document.getElementById('consonants_lists').value = consonants_lists;
- document.getElementById('vowels').value = total_vowels;
- document.getElementById('all_vowels').value = count;
- document.getElementById('no_digits').value = count_digits(str);
- document.getElementById('digits_list').value = retnum(str);
- }
- function reset_all()
- {
- document.getElementById('all_consonants').value ="";
- document.getElementById('consonants_lists').value ="";
- document.getElementById('vowels').value = "";
- document.getElementById('all_vowels').value = "";
- document.getElementById('no_digits').value ="";
- document.getElementById('digits_list').value ="";
- document.getElementById('sentence_counter').value ="";
- document.getElementById('sentence_counter').focus();
- }
- </script>
- <style type="text/css">
- body {
- width:700px;
- margin:auto;
- }
- .wrapper{
- border: blue 1px solid;
- padding: 10px;
- margin: 25px;
- }
- td {
- font-size: 18px;
- color: blue;
- text-align: center;
- font-weight: bold;
- font-family: cursive;
- }
- .btn_enter {
- width: 100px;
- font-size: 18px;
- color: blue;
- background: azure;
- border: blue 1px solid;
- border-radius: 4px;
- padding: 5px;
- cursor:pointer;
- margin-right:20px;
- }
- .btn_cancel {
- width: 100px;
- font-size: 18px;
- color: red;
- background: azure;
- border: red 1px solid;
- border-radius: 4px;
- padding: 5px;
- cursor:pointer;
- }
- .txtBox_info {
- border: blue 1px solid;
- font-size: 18px;
- text-indent: 10px;
- background: azure;
- cursor: pointer;
- }
- .txtBox_readOnly {
- border: blue 1px solid;
- font-size: 18px;
- text-indent: 10px;
- text-align:center;
- color:red;
- background: #d0d0d0;
- cursor: no-drop;
- }
- </style>
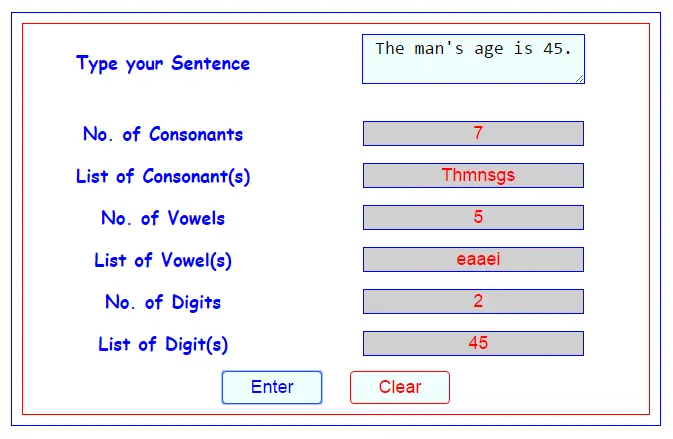