How to Calculate and Display Output Using Dialog Boxes
Submitted by GeePee on Thursday, May 14, 2015 - 23:27.
The following program prompts the user to enter the radius of a circle. The program then outputs the circle’s radius, area and circumference. . I will be using the JCreator IDE in developing the program.
To start in this tutorial, first open the JCreator IDE, click new and paste the following code.
Sample Run:
The class
- import javax.swing.JOptionPane;
- public class AreaCircumference
- {
- public static final double PI = 3.14;
- {
- double radius;
- double area;
- String outputStr;
- String radiusString;
- double circumference;
- area = PI * radius * radius;
- circumference = 2 * PI * radius;
- outputStr = "Radius: " + radius + "\n"+
- "Area: " + area + " square units\n"+
- "Circumference: " + circumference + " square units\n";
- }
- }
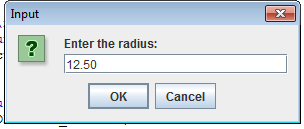
JOptionPane
is contained in the package javax.swing
. Therefore to use this class in a program, the program must import this class from the package javax.swing
. The following statement illustrates how to import class JOptionPane
.
import javax.swing.JOptionPane;
The statement public static final double PI = 3.14;
declares and initializes the named constant PI =3.14. The statement double radius, double radius, double area, String outputStr; String radiusString, double circumference
declare the appropriate variables to manipulate the data.
The statement radiusString = JOptionPane.showInputDialog("Enter the radius: ")
displays the input dialog box with the message Enter the radius:
The string containing the input data is assign to the String variable radiusString
. The statement radius = Double.parseDouble(radiusString);
converts the string containing the radius into a value of the type double and stores it in the variable radius.
The statement area = PI * radius * radius;
and circumference = 2 * PI * radius;
calculate the area and circumference of the circle and store them in the variables area and circumference. The statement outputStr = "Radius: " + radius + "\n" + "Area: " + area + " square units\n" + "Circumference: " + circumference + " square units\n";
constructs the string containing the radius, area and circumference of the circle.
The string is assigned to the variable outputStr. The statement JOptionPane.showMessageDialog(null, outputStr, "Circle", JOptionPane.INFORMATION_MESSAGE);
uses the message dialog box to display the circle’s radius, area and circumference . The statement System.exit(0);
terminates the program after the user presses the OK button in the dialog box.