Compute Exponent in Java
Submitted by donbermoy on Friday, December 26, 2014 - 21:06.
This tutorial will teach you how to create a program that will compute exponent in java.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of powExponent.java.
2. Import the io package library:
3. We will initialize variables in our Main, variable in for BufferedReader as our input, num and power as Integer.
4. Now, we will have inputting for the base number and the exponent. Have the code below:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import java.io.*;
- int num;
- int power;
5. Lastly, we will display compute the exponential number using the Math.pow method.
The Math.pow method has the following parameters of a base number and the exponent (basenumber,exponent). Then it will compute the exponential number.
Output:
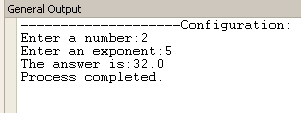
- import java.io.*;
- public class powExponent {
- int num;
- int power;
- }
- }