Convert String to Time Object in Java
Submitted by donbermoy on Friday, December 26, 2014 - 21:05.
This tutorial will teach you how to create a program that will convert a string into a time object using java.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of timeParse.java.
2. Import the following package library:
3. We will initialize variables in our Main, time as String, sdf as DateFormat, and date as Date.
As what you have seen the code above, i have initialized my time as String that holds the value of 23:30:11. Then, we used the DateFormat class to have a formatting of our time. And we used the Date to access the current date and time. To have a conversion of string to time, we will use the parse method of the SimpleDateFormat that has the time variable as String.
4. To display the converted string into time, have the code below:
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import java.text.*; //use to access DateFormat and SimpleDateFormat class
- import java.util.*; // use to access Date class
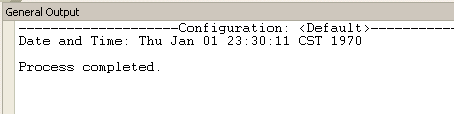
- import java.text.*; //use to access DateFormat and SimpleDateFormat class
- import java.util.*; // use to access Date class
- public class timeParse {
- }
- }