How to Create a Compound Border in Java
Submitted by donbermoy on Monday, December 8, 2014 - 22:24.
This tutorial will teach you how to create a compound border in Java. A compound border is a border nested with other borders. Thus, it is composed of two border objects.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of compoundBorders.java.
2. Import the following package library:
3. We will initialize variables in our Main, variable frame as JFrame and button as JButton.
4. Now, we will create first the line borders. Remember that the first parameter of the createLineBorder method from the BorderFactory class is the color of the line and next is the thickness of the line as a pixel.
Then, to declare the Compound Border we will use the Border class to instantiate the CompoundBorder class. As such, this is a parameter for creating a CompoundBorder:
Now, we will nest all the line borders that we have created above using the CompoundBorder. Have this code below:
Add the last border that you have created in your CompoundBorder in the button.
5. Now, we will have its layout into Grid Layout using the setLayout method of the frame.
Then add the buttons into the frame using the add method. Lastly, set the size, visibility, and the close operation of the frame. Have this code below:
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import java.awt.*; //used to access Color and GridLayout class
- import javax.swing.*; //used to access BorderFactory, JFrame, and JButton class
- import javax.swing.border.*; //used to access Border, LineBorder, and CompoundBorder class
- button.setBorder(border5);
- frame.getContentPane().add(button1);
- frame.getContentPane().add(button2);
- frame.setSize(300, 100);
- frame.setVisible(true);
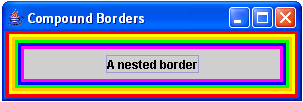
- import java.awt.*; //used to access Color and GridLayout class
- import javax.swing.*; //used to access BorderFactory, JFrame, and JButton class
- import javax.swing.border.*; //used to access Border, LineBorder, and CompoundBorder class
- public class compoundBorders {
- button.setBorder(border5);
- frame.getContentPane().add(button);
- frame.setSize(300, 100);
- frame.setVisible(true);
- }
- }