How to Put an HTML code in a Button in Java
Submitted by donbermoy on Wednesday, December 3, 2014 - 10:17.
This tutorial is about how to put an HTML code in a button in Java. The HTML tags will be put inside the JButton to display text.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of htmlInButton.java.
2. Import the javax.swing.* package library that is used to access the JButton, and JFrame class.
3. Initialize your variable in your Main, variable frame for JFrame, and variable button as JButton.
4. Now, to create an HTML code inside the button we will use the setText method of our button. The setText method can recognize HTML code. It has an advantage of calling a text with a font, color, alignment, font style without using the classes of awt library like font class, color class, alignment, and the styles of the font. Have this code below:
html - an html tag
font face='Tahoma'" + " color=blue - a font tag with a font style of tahoma and font color of blue
b- start of the bold tag
This text has an HTML Code - the text inside the html with a specific font of tahoma and blue color of font
font face='courier new'" + " color=red - a font tag with a font style of courier new and font color of red
center - a center alignment tag
Sourcecodester - the text inside the html with a specific font of courier new and red color of font
Add the button to the frame using the add method.
5. Lastly, set the size, set visibility to true, and the close operation of the frame. Have this code below:
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import javax.swing.*; //used to access the JButton, and JFrame class.
- button.setText ("<html><font face='Tahoma'" + " color=blue><b>This text has an HTML Code<b></font><br><font face='courier new'" + " color=red><center>Sourcecodester<center></font></html>");
- frame.getContentPane().add(button);
- frame.setSize(300, 200);
- frame.setVisible(true);
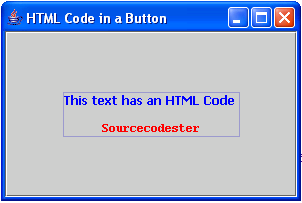
- import javax.swing.*; //used to access the JButton, and JFrame class.
- public class htmlInButton{
- button.setText ("<html><font face='Tahoma'" + " color=blue><b>This text has an HTML Code<b></font><br><font face='courier new'" + " color=red><center>Sourcecodester<center></font></html>");
- frame.getContentPane().add(button);
- frame.setSize(300, 200);
- frame.setVisible(true);
- }
- }