FlowLayout as Layout Manager in Java
Submitted by donbermoy on Saturday, November 29, 2014 - 08:34.
This is a tutorial in which we will going to create a program that will have a FlowLayout as Layout Manager in Java. A FlowLayout as a layout manager provides a layout that is simple and used as default by the JPanel. It makes it every component seen according to its preferred size and arranges them in horizontal wrapping lines so that they will have spacing.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of flowLayout.java.
2. Import the following packages:
3. In your class, extends it to JFrame and implement it with an ActionListener because we will use such event in the radio buttons. Initialize the following variables below:
As you what you have seen the code above, we have 3 radio buttons labeled Left, Right, and Center button. We also initialize variable bg as ButtonGroup so that we can group the radio buttons. We also make variable con as a Container that will provide the pane as a container of the components. And to use the flow layout, we have variable layout declared as a FlowLayout class.
4. Now, we will make a constructor named flowLayout.
Inside of this, we add the radio button components by using the add method of our container variable.
To group the radio buttons, we will use the add method of the ButtonGroup variable.
We will add then the action listener of the radio buttons by using the addActionListener method and the parameter this inside the radio button. The this keyword indicates that it is in the current file.
Then use the setLayout method of the container and put the variable layout as a FlowLayout inside of it.
5. Now, we will create a listener and the event for the radio buttons. Have this code below:
In every radio buttons, their is a corresponding alignment for the FlowLayoutusing the setAlignment method. The layoutContainer method indicates that the layout used in the container is the FlowLayour.
6. Now, in your main, instantiate the class name or the constructor inside and make it named as frame as a variable. Lastly set the size, visibility, and title of the frame.
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import javax.swing.*; // used to access the JFrame, JRadioButton, ButtonGroup, and Container class
- import java.awt.*; // used to access the FlowLayout class
- import java.awt.event.*; //used to access the ActionEvent and ActionListener class
- con.add(rbLeft);
- con.add(rbCenter);
- con.add(rbRight);
- bg.add(rbLeft);
- bg.add(rbRight);
- bg.add(rbCenter);
- rbLeft.addActionListener(this);
- rbRight.addActionListener(this);
- rbCenter.addActionListener(this);
- con.setLayout(layout);
- setDefaultCloseOperation(EXIT_ON_CLOSE);
- if(source == rbLeft)
- {
- }
- else if (source == rbRight){
- }
- else{
- }
- layout.layoutContainer(con);
- }
- flowLayout frame = new flowLayout();
- frame.setSize(300, 100);
- frame.setVisible(true);
- frame.setTitle("FlowLayout as Layout Manager");
- }
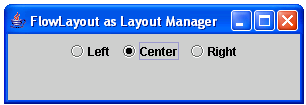
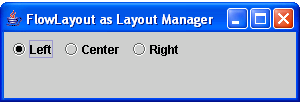
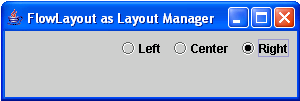
- import javax.swing.*; // used to access the JFrame, JRadioButton, ButtonGroup, and Container class
- import java.awt.*; // used to access the FlowLayout class
- import java.awt.event.*; //used to access the ActionEvent and ActionListener class
- public flowLayout() {
- con.add(rbLeft);
- con.add(rbCenter);
- con.add(rbRight);
- bg.add(rbLeft);
- bg.add(rbRight);
- bg.add(rbCenter);
- rbLeft.addActionListener(this);
- rbRight.addActionListener(this);
- rbCenter.addActionListener(this);
- con.setLayout(layout);
- setDefaultCloseOperation(EXIT_ON_CLOSE);
- }
- if(source == rbLeft)
- {
- }
- else if (source == rbRight){
- }
- else{
- }
- layout.layoutContainer(con);
- }
- flowLayout frame = new flowLayout();
- frame.setSize(300, 100);
- frame.setVisible(true);
- frame.setTitle("FlowLayout as Layout Manager");
- }
- }