JScrollPane Component in Java
Submitted by donbermoy on Monday, November 24, 2014 - 13:34.
This is a tutorial in which we will going to create a program that will have a JScrollPaneComponent in Java. A JScrollPane implements scrollable view of a component that lets the user display a component that is large or one whose size can change using scrollbars.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of jScrollPaneComponent.java.
2. Import the following packages:
3. Initialize your variable in your Main, variable frame for JFrame, variable pane for JPanel, variable scroll for JScrollPane, label for JLabel, and variable txtFont for Font.
As you have seen the code above, we have put the panel inside the scrollpane. The ScrollPaneConstants.VERTICAL_SCROLLBAR_ALWAYS code will trigger to always show the vertical scrollbar of the panel. The ScrollPaneConstants.HORIZONTAL_SCROLLBAR_ALWAYS code will trigger to always show the horizontal scrollbar of the panel. We have also created the Font class that has the Tahoma as font, Bold as font style, and 38 as font size.
Now, we will add the label to the panel using the add method and the font of the label using the setFont method. Have this code below:
4. Now, add the JScrollPane variable to the frame using the setContentPane().add method. Lastly, set the size, visibility, and the close operation of the frame. Have this code below:
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import javax.swing.*; // used to access the JFrame, JPanel, JLabel, and JScrollPane class
- import java.awt.*; // used to access the Font class
- JScrollPane scroll = new JScrollPane(panel, ScrollPaneConstants.VERTICAL_SCROLLBAR_ALWAYS, ScrollPaneConstants.HORIZONTAL_SCROLLBAR_ALWAYS);
- panel.add(label);
- label.setFont(txtFont);
- frame.setContentPane(scroll);
- frame.setSize(300, 100);
- frame.setVisible(true);
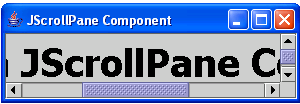
- import javax.swing.*; // used to access the JFrame, JPanel, JLabel, and JScrollPane class
- import java.awt.*; // used to access the Font class
- public class jScrollPaneComponent {
- JScrollPane scroll = new JScrollPane(panel, ScrollPaneConstants.VERTICAL_SCROLLBAR_ALWAYS, ScrollPaneConstants.HORIZONTAL_SCROLLBAR_ALWAYS);
- panel.add(label);
- label.setFont(txtFont);EXIT_ON_CLOSE
- frame.setContentPane(scroll);
- frame.setSize(300, 100);
- frame.setVisible(true);
- }
- }