JMenuBar, JMenu, and JMenuItem Component in Java
Submitted by donbermoy on Wednesday, November 19, 2014 - 00:08.
This is a tutorial in which we will going to create a program that has the JMenuBar, JMenu, and JMenuItem Component using Java. A menu is a component that provides a way to let the user choose one of several options that can hold other components as choices such as combobox, radio buttons, spinners, and tool bars, and lists.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of jTabbedPaneComponent.java.
2. Import the javax.swing package to access the JFrame,JButton, JLabel,JTextField, and the JTabbedPane class.
3. Initialize your variable in your Main, variable frame for creating JFrame, variable menuBar for JMenuBar, variable fileMenu and transMenu for JMenu class.
4. Now, we will create a JMenuItem class to create items in the menu bar. We will have two menus for the File and Transaction Menu. For the File Menu, we will create New and Save items. In the Transaction Menu, we will create Copy and Paste items. Have his code below:
5. Now, to add the JMenuBar to the frame we will not use the getContentPane.add method but instead we will use the setJMenuBar of the frame.
6. Lastly set the size, visibility, and the close operation of the frame. Have this code below:
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import javax.swing.*; // used to access JFrame, JMenu, JMenuBar, and JMenuItem class
The JMenuBar class here provides the component of the JMenu bar and the JMenu is the menu container of an item to be placed on the menu.
To simply add a menu for transactions to the menu bar, we will use the add method of the JMenuBar. We will have two menus on the menu named File and Transaction. Have this code below:
- menuBar.add(fileMenu);
- menuBar.add(transMenu);
To add items on the File and Transaction menu, we will use add method of the JMenu class and put inside the variables for JMenuItem. We will also use addSeparator method of the JMenuItem class to have a separator line to the items in the menu bar.
- transMenu.add(copyItem);
- fileMenu.addSeparator();
- transMenu.add(pasteItem);
- frame.setJMenuBar(menuBar);
- frame.setSize(350, 250);
- frame.setVisible(true);
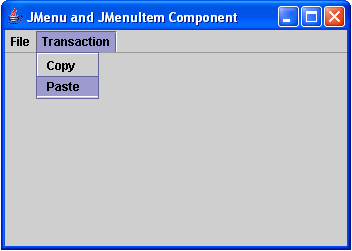
- import javax.swing.*; // used to access JFrame, JMenu, JMenuBar, and JMenuItem class
- public class jMenuComponent {
- menuBar.add(fileMenu);
- menuBar.add(transMenu);
- fileMenu.add(newMenuItem);
- fileMenu.addSeparator();
- fileMenu.add(saveMenuItem);
- transMenu.add(copyItem);
- fileMenu.addSeparator();
- transMenu.add(pasteItem);
- frame.setJMenuBar(menuBar);
- frame.setSize(350, 250);
- frame.setVisible(true);
- }
- }