How to Insert a Text in a Specified Position of JTextArea Component in Java GU
Submitted by donbermoy on Thursday, May 22, 2014 - 10:50.
This is a continuation of my other tutorials entitled JTextArea Component in Java GUI and Set Font and Color to Text of JTextArea Component in Java GUI. But in this tutorial, i will teach you how to insert a text in a specified position of JTextArea component using Java GUI.
1. First and foremost, Open JCreator or NetBeans and make a java program with a file name of jTextArea.java. When you click the link above, copy first all the code there.
2. Now, insert this code after the JScrollPane scrollingArea = new JScrollPane(txtArea);. This code will insert a text in your desired position of JTextArea component.
The txtArea is our variable for JTextArea component that we used the insert method here. This insert method here allows to insert a text at the specified position. Meaning insert(String text,int position)
is the syntax. Here, i used the word "Sourcecodester" at the position 0. This 0 means will be placed before the start of "Encode". Take note that you can also change the word i have inserted. I also used again the insert method with the word "best" in the 27th position which means the word will be place after the word "more".
Output:
Hope this helps :)
Best Regards,
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import java.awt.*;
- import javax.swing.*;
- public jTextArea() {
- txtArea.setText("Encode more text to see scrollbars");
- this.setContentPane(content);
- this.pack();
- }
- txtArea.setTitle("JTextArea Component");
- txtArea.setVisible(true);
- txtArea.setSize(250,140);
- txtArea.setLocation(300,300);
- }
- }
- txtArea.insert("Sourcecodester ", 0);
- txtArea.insert(" best ", 27);
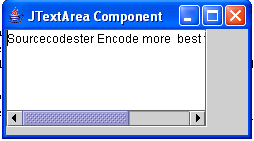