Textbox Validation using Error Provider in C#
Submitted by donbermoy on Tuesday, July 22, 2014 - 13:52.
Today in C#, I will teach you how to create a program that will validate an inputted textbox using ErrorProvider. In this tutorial, you will know what are the inputs you are going to put in the textbox and it will notify you whatever errors that you might encounter.
Now, let's start this tutorial!
1. Let's start with creating a Windows Form Application in C# for this tutorial by following the following steps in Microsoft Visual Studio 2010: Go to File, click New Project, and choose Windows Application.
2. Add 2 textbox named txtstring by inputting string/text and txtnumber to input numbers only. Then insert one button named Button1. Design your interface like this:
3. Now put this code in your Button1_Click to validate the textbox.
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
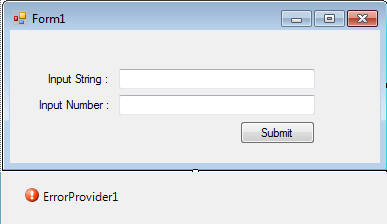
- private void Button1_Click(System.Object sender, System.EventArgs e)
- {
- //CHECKING IF THE TWO TEXTBOXES ARE CLEARED
- if (txtstring.Text == "" && txtnumber.Text == "")
- {
- ErrorProvider1.SetError(txtstring, "Field must be filled up.");
- ErrorProvider1.SetError(txtnumber, "Field must be filled up.");
- }
- else
- {
- //THE ERROR PROVIDER WILL BE CLEARED.
- ErrorProvider1.SetError(txtstring, "");
- ErrorProvider1.SetError(txtnumber, "");
- //CONDITION FOR THE STRING VALUE
- //CHECK IF THE INPUT IS A NUMERIC VALUE THEN PERFORM THE ERROR PROVIDER
- //IF NOT, CLEAR THE ERROR PROVIDER.
- if (IsNumeric(txtstring.Text))
- {
- //THE ERROR PROVIDER WILL APPEAR AND WILL NOTIFY THE PROBLEM TO THE USER.
- ErrorProvider1.SetError(txtstring, "Input string value is not valid.");
- }
- else
- {
- //THE ERROR PROVIDER WILL BE CLEARED.
- ErrorProvider1.SetError(txtstring, "");
- }
- //CONDITION FOR THE NUMERIC VALUE
- //CHECK IF THE INPUT IS NOT A NUMERIC VALUE THEN PERFORM THE ERROR PROVIDER
- //IF NUMERIC, CLEAR THE ERROR PROVIDER.
- if (!IsNumeric(txtnumber.Text))
- {
- //THE ERROR PROVIDER WILL APPEAR AND WILL NOTIFY THE PROBLEM TO THE USER.
- ErrorProvider1.SetError(txtnumber, "Input numeric value is not valid.");
- }
- else
- {
- //THE ERROR PROVIDER WILL BE CLEARED.
- ErrorProvider1.SetError(txtnumber, "");
- }
- }
- }
Output:
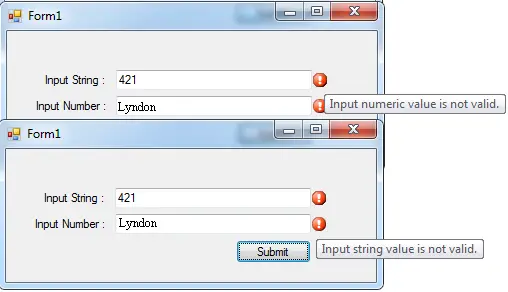