Get the Number of Occurences of a Text in a ListBox using C#
Submitted by donbermoy on Thursday, July 17, 2014 - 19:33.
In this C# tutorial, we will create a program that can determine the most repeated string or text inputted that is displayed in the ListBox.
Now, let's start this tutorial!
1. Let's start with creating a Windows Form Application in C# for this tutorial by following the following steps in Microsoft Visual Studio 2010: Go to File, click New Project, and choose Windows Application.
2. Next, add one TextBox named TextBox1 that will serve as your input, two buttons named Button1 for adding the inputted text in the ListBox and Button2 for getting and displaying the most repeated text in a ListBox, and one listbox named ListBox1 for displaying the list of inputted text/items in the textbox. You must design your interface like this:
3.Now put this code for your code module.
For Button1, we used this button to add the inputted string in the textbox to be displayed in the listbox. Then after displaying, it will clear the inputted string inside the textbox.
For Button2, this button is used to determine the most repeated text inside the listview. We have initialized variable ob1, ob2, ob3 As String and variable num1, num2 As Integer. Then, we have created a nested For Each loop. We have first initialized variable ob1 in the for each loop to hold the items of the listbox, then the variable ob2. If variable ob1 and ob2 are equal num1 will increment by 1. if variable num1 is greater than variable num2 then the two variables will be equal and variable ob3 will now hold the value of variable ob3 then the num1 will be equal to be 0. The ob3 variable here holds the string value that is the most repeated text in the listbox and variable num2 holds the number of times it occurs in the listbox.
Output:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
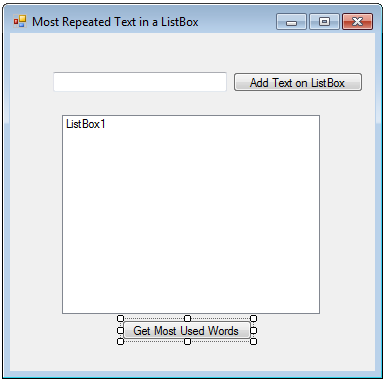
- using System.Windows.Forms;
- public class Form1
- {
- private void Button1_Click(System.Object sender, System.EventArgs e)
- {
- ListBox1.Items.Add(TextBox1.Text);
- TextBox1.Clear();
- }
- private void Button2_Click(System.Object sender, System.EventArgs e)
- {
- string ob1 = default(string);
- string ob2 = default(string);
- string ob3 = default(string);
- int num1 = default(int);
- int num2 = default(int);
- foreach (string tempLoopVar_ob1 in ListBox1.Items)
- {
- ob1 = tempLoopVar_ob1;
- foreach (string tempLoopVar_ob2 in ListBox1.Items)
- {
- ob2 = tempLoopVar_ob2;
- if (ob1 == ob2)
- {
- num1++;
- }
- }
- if (num1 > num2)
- {
- num2 = num1;
- ob3 = ob1;
- }
- num1 = 0;
- }
- MessageBox.Show(ob3 + " is repeated " + num2.ToString() + " times");
- }
- }
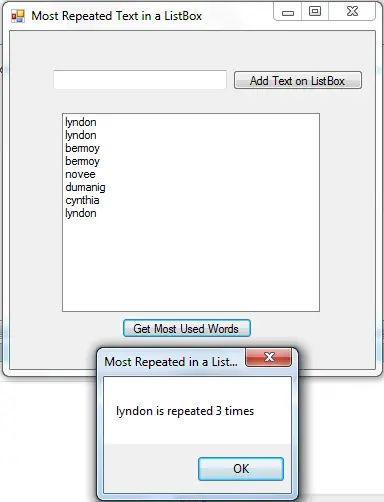