How to hava a Complex Password in C#
Submitted by donbermoy on Thursday, July 17, 2014 - 19:08.
Today in C#, we will create a program that can determine if the inputted password is complex or not. Strong passwords meet a number of requirements for complexity - including length and character categories - that make passwords more difficult for attackers to determine. Establishing strong password policies for your organization can help prevent attackers from impersonating users and can thereby help prevent the loss, exposure, or corruption of sensitive information.
Now, let's start this tutorial!
1. Let's start with creating a Windows Form Application in C# for this tutorial by following the following steps in Microsoft Visual Studio 2010: Go to File, click New Project, and choose Windows Application.
2. Next, add only one Button named Button1 and labeled it as "Submit" for determining the complexity of the password. Add one textbox named TextBox1 for inputting your desired password. Add two labels named Label1 labeled it as "Password is Complex:" and Label2 which is an empty string that has a boolean value for displaying the complexity. You must design your interface like this:
3. Import System.text.RegularExpressions.
We imported this namespace because it enables us to determine whether a particular string conforms to a regular expression pattern.
4. Create a function named ValidatePassword. Put this code in your code module:
We have created ValidatePassword Function that has the following parameters of a password as string, minimum length of the password is 8, Capital Letter and Lower Letter must have a minimum of two letters, two digits of numbers, and must have a minimum of 2 special characters to make it complex. Then we have initialized variable upper to have capitalized letter, variable lower to have non-capitalized letter, variable number to have numerical inputs, and we used RegEx because it will hold the regular expression of not having a number or letter but to have a special character which it holds by variable special. Then it checks the length, if it will not have a minimum of 8 characters then it will return False as well as the other parameters. If it meets all the requirements, then it will display True.
5. Then, put this code to button1_click. This will display the output in boolean in Label2.
A complex password
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
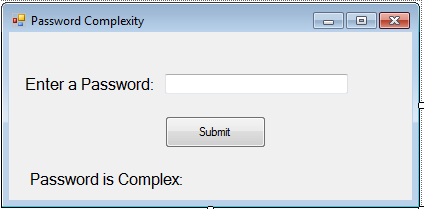
- using System.Text.RegularExpressions;
- public bool ValidatePassword(string pwd, int minLength = 8, int numUpper = 2, int numLower = 2, int numNumbers = 2, int numSpecial = 2)
- {
- // Replace [A-Z] with \p{Lu}, to allow for Unicode uppercase letters.
- // Special is "none of the above".
- System.Text.RegularExpressions.Regex special = new System.Text.RegularExpressions.Regex("[^a-zA-Z0-9]");
- // Check the length.
- if (pwd.Length < minLength)
- {
- return false;
- }
- // Check for minimum number of occurrences.
- if (upper.Matches(pwd).Count() < numUpper)
- {
- return false;
- }
- if (lower.Matches(pwd).Count() < numLower)
- {
- return false;
- }
- if (number.Matches(pwd).Count() < numNumbers)
- {
- return false;
- }
- if (special.Matches(pwd).Count() < numSpecial)
- {
- return false;
- }
- // Passed all checks.
- return true;
- }
- private void Button1_Click(object sender, EventArgs e)
- {
- Label2.Text = ValidatePassword(TextBox1.Text);
- }
Output:
Not a complex password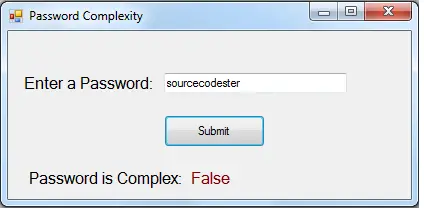
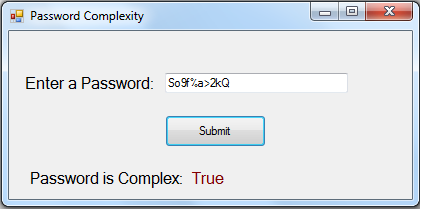