Math Functions in C#
Submitted by donbermoy on Friday, July 11, 2014 - 13:41.
Today, I will teach you how to create a program that has math functions in C#. Here you will learn about Abs, Log, Round, Sin, Cos, and Tan Function.
Now, let's start this tutorial!
1. Let's start with creating a Windows Form Application in C# for this tutorial by following the following steps in Microsoft Visual Studio 2010: Go to File, click New Project, and choose Windows Application.
2. Next, add two textboxes named txtNum for your inputted number and txtOut for the output. Insert 6 Buttons named btnAbs for Absolute Value, btnLog for Logarithm, btnRound for Rounding off, btnSin for finding the sine of an angle, btnCos for the Cosine of an angle, and btnTan for the Tangent of the angle. You must design your interface like this:
3. In your btnAbs for Absolute Value, copy this code below. This Abs function will return absolute value of our inputted number, means even if we input negative number, the output will be positive.
4. In your btnLog for finding the Logarithm of a number, copy this code below. This Log function will return the logarithmic value of our inputted number.
5. In your btnRound for rounding off a number, copy this code below. This Round function will return the inputted number to nearest the specified value. It means that if the nearest value after the decimal point is 5, then it will increase by 1 the whole number. Otherwise, the whole number will be the same.
6. In your btnSin for finding the sine value of the inputted number (angle), copy this code below. This Sin function will return the specifying the sine of an angle as our inputted number.
7. In your btnCos for finding the cosine value of the inputted number (angle), copy this code below. This Cosine function will return the specifying the cosine of an angle as our inputted number.
8. In your btnTan for finding the tangent value of the inputted number (angle), copy this code below. This Tan function will return the specifying the tangent of an angle as our inputted number.
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
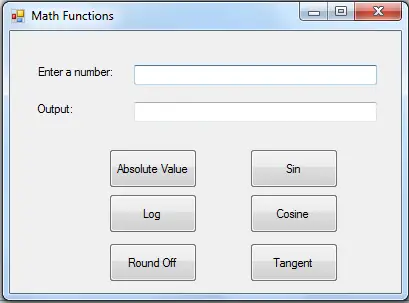
- public void btnAbs_Click(System.Object sender, System.EventArgs e)
- {
- txtOut.Text = System.Convert.ToString(Math.Abs(Conversion.Val(txtNum.Text)));
- }
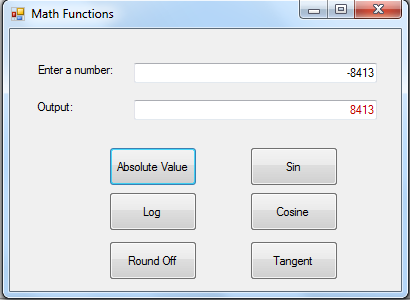
- public void btnLog_Click(System.Object sender, System.EventArgs e)
- {
- txtOut.Text = System.Convert.ToString(Math.Log(Conversion.Val(txtNum.Text)));
- }
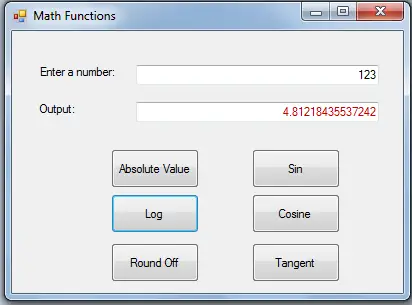
- public void btnRound_Click(System.Object sender, System.EventArgs e)
- {
- txtOut.Text = System.Convert.ToString(Math.Round(Conversion.Val(txtNum.Text)));
- }
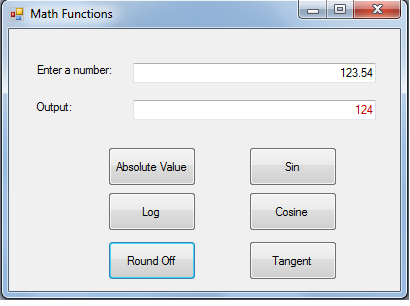
- public void btnSin_Click(System.Object sender, System.EventArgs e)
- {
- txtOut.Text = System.Convert.ToString(Math.Sin(Conversion.Val(txtNum.Text)));
- }
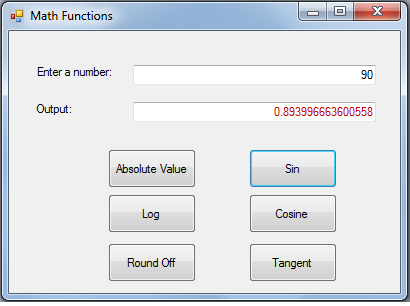
- public void btnCos_Click(System.Object sender, System.EventArgs e)
- {
- txtOut.Text = System.Convert.ToString(Math.Cos(Conversion.Val(txtNum.Text)));
- }
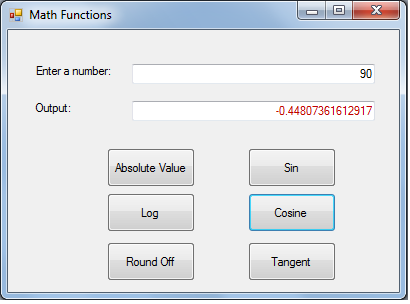
- public void btnTan_Click(System.Object sender, System.EventArgs e)
- {
- txtOut.Text = System.Convert.ToString(Math.Tan(Conversion.Val(txtNum.Text)));
- }
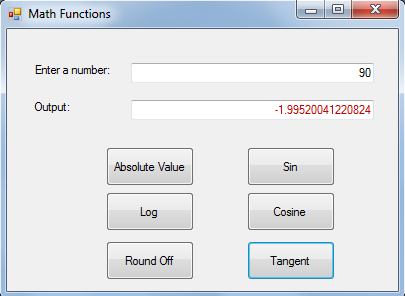