Declaring a Simple Structure in C#
Submitted by donbermoy on Saturday, June 28, 2014 - 13:26.
This is a C# tutorial that will be based on how to declare a structure function. We all know that a structure is similar to a class, but is a value type, not a reference type.
To declare a struct in C#, have this syntax:
A structure or a struct cannot be used as a base for other structures or classes, cannot inherit other structures or classes, can also define constructors, but not destructors, and structure members can be methods, fields, indexers, properties, operator methods, and events but cannot be specified as abstract, virtual, or protected.
Now, let's start this tutorial!
1. Let's start with creating a Console Application in C# for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Console Application and name your project as structure.
2. Now, to declare a structure put this code outside of your Main.
We declare a structure here with the name Fraction and has the struct keyword. Inside of it are the declaration of the variables numerator and denominator as an integer and has the public modifier to be accessible inside or outside of the program.
3. Now, put this code for your main and call the structure you have coded.
To call the structure, we called the structure named Fraction that we have created a while ago. We also created and f variable on it to pass the statements inside the structure function. Next, the f variable has a dot and called the numerator and denominator inside the structure. The dot indicates to call all the variables declared inside the structure and we put a value 15 in the numerator and 3 in the denominator. Then we have created a quotient variable as double and has the operation of division. Then we display the output using the Console.Writeline syntax.
Press Ctrl+F5 to run the program.
Output:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- struct name : interfaces {
- // member declarations
- }
- struct Fraction
- {
- public int numerator;
- public int denominator;
- }
- static void Main(string[] args)
- {
- double quotient;
- Fraction f;
- f.numerator = 15;
- f.denominator = 3;
- quotient = f.numerator / f.denominator;
- Console.WriteLine("The quotient is:" + quotient);
- }
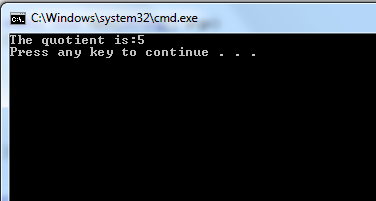