How to Compute Date Difference in C#
Submitted by donbermoy on Sunday, June 8, 2014 - 22:02.
Today in C#, i will teach you how to create a program that finds and calculates the difference between two dates using C#.
So, now let's start this Date Difference tutorial in C#!
1. Let's start with creating a Windows Form Application in C# for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application and name your project as Date Difference.
2. Next, add two DateTimePicker named DateTimePicker1 and DateTimePicker2 in the ToolBox. Then add a button named Button1 labeled as "Compute Date Difference". Take note that you have to place DateTimePicker2 above DatePicker1. You must design your interface like this:
3. To have the current value of the two DateTimePicker component, put the following code to Form_Load.
4. Now, put this code in Button1_Click. This will display the result of the DateDifference from the two DateTimePicker.
Initialize firstDate variable as DateTime that holds the value of DateTimePicker1 and secondDate variable as DateTime that holds the value of DateTimePicker2.
Now, compute the date difference between the two DateTimePicker with a diff2 variable as string using the minus opearation. The variable diff2 holds the Date Difference between the secondDate and the FirstDate with their total days to minus with a ToString function to convert it to string.
Then after being subtracted it is now converted into string. Then it will display the Date Difference of the two DateTimePicker.
Full source code:
Press F5 to run the program.
Output:
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
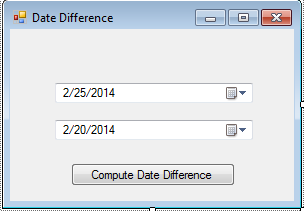
- private void Form1_Load(object sender, EventArgs e)
- {
- DateTimePicker1.Value = DateTime.Today;
- DateTimePicker2.Value = DateTime.Today;
- }
- DateTime firstTime = default(DateTime);
- DateTime secondTime = default(DateTime);
- firstTime = DateTimePicker1.Value;
- secondTime = DateTimePicker2.Value;
- string diff2 = (string) ((secondTime - firstTime).TotalDays.ToString());
- MessageBox.Show("The date difference is:" + " " + diff2.ToString());
- using System.Diagnostics;
- using System;
- using System.Windows.Forms;
- using System.Collections;
- using System.Drawing;
- using System.Data;
- using System.Collections.Generic;
- namespace DateDifference
- {
- public partial class Form1
- {
- public Form1()
- {
- InitializeComponent();
- }
- public void Button1_Click(System.Object sender, System.EventArgs e)
- {
- DateTime firstTime = default(DateTime);
- DateTime secondTime = default(DateTime);
- firstTime = DateTimePicker1.Value;
- secondTime = DateTimePicker2.Value;
- string diff2 = (string) ((secondTime - firstTime).TotalDays.ToString());
- MessageBox.Show("The date difference is:" + " " + diff2.ToString());
- }
- private void Form1_Load(object sender, EventArgs e)
- {
- DateTimePicker1.Value = DateTime.Today;
- DateTimePicker2.Value = DateTime.Today;
- }
- }
- }
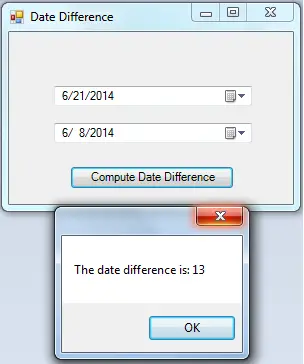