Create Folder using C#
Submitted by donbermoy on Saturday, June 7, 2014 - 19:49.
This is a continuation of my other tutorial entitled Delete Folder and Its Content using C# but now we will create here a program that will create a folder using C# also.
Now, let's start this tutorial!
1. Let's start with creating a Windows Form Application in C# for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application and name your project as Create Folder.
2. Next, add only one Button named Button1 and labeled it as "Create Folder". Add also TextBox named TextBox1 for our inputting the path of the folder that you want to delete. You must design your interface like this:
3. To achieve that, first of all we need to include the following statement in the program code:
using System.IO - This namespace has to precede the whole program code as it is higher in hierarchy for DirectoryInfo. In Fact, this is the concept of object oriented programming where DirectoryInfo is part of the namespace System.IO.
4. Now to create a folder, we will now code for Button1.
The
Full source code:
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
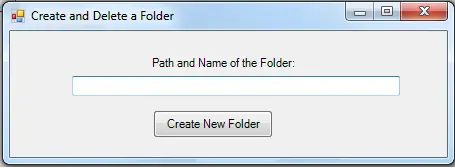
- using System;
- using System.Collections.Generic;
- using System.ComponentModel;
- using System.Data;
- using System.Drawing;
- using System.Text;
- using System.Windows.Forms;
- using System.IO;
- public void Button1_Click(System.Object sender, System.EventArgs e)
- {
- if (di.Exists)
- {
- MessageBox.Show("That path exists already.");
- TextBox1.Clear();
- }
- else
- {
- di.Create();
- Interaction.MsgBox("Folder Is Created.......!", MsgBoxStyle.Information, "Create Folder");
- TextBox1.Clear();
- }
- }
DirectoryInfo class
above is used to locate and have a directory of a file or folder. It passes the value of the directory as the input of Textbox1.
If directory is already existed (di.Exists) then it will create the TextBox1 and display "That path exists already.". Otherwise, it will create a New Folder saved in the Directory and Folder name that corresponds to TextBox1. It will also clear the value of the TextBox1 and display "Folder Is Created.......!".
Now, try to type this on your TextBox1: C:/sample. Then Click Button1. This will display the result that the folder is created. Then if you type again C:/sample, the program will prompt the user that the path is already existed.
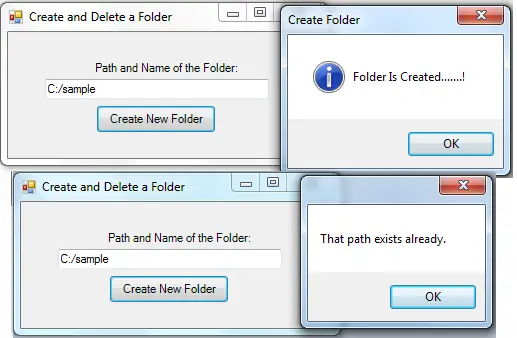
- using System.Diagnostics;
- using System;
- using System.Windows.Forms;
- using System.Collections;
- using System.Drawing;
- using System.Data;
- using System.Collections.Generic;
- using System.IO;
- namespace Create_and_Delete_a_Folder
- {
- public partial class Form1
- {
- public Form1()
- {
- InitializeComponent();
- }
- public void Button1_Click(System.Object sender, System.EventArgs e)
- {
- if (di.Exists)
- {
- MessageBox.Show("That path exists already.");
- TextBox1.Clear();
- }
- else
- {
- di.Create();
- Interaction.MsgBox("Folder Is Created.......!", MsgBoxStyle.Information, "Create Folder");
- TextBox1.Clear();
- }
- }
- }
- }