URL Validation using C#
Submitted by donbermoy on Wednesday, June 4, 2014 - 19:00.
Today, i will teach you how to create a program that will validate a URL inputted using C#. We know that a URL is the address of a specific Web site or file on the Internet. It cannot have spaces or certain other characters and uses forward slashes to denote different directories. Some examples of URLs are http://www.sourcecodester.com/, http://google.com/, etc.
Now, let's start this validating a URL tutorial!
1. Let's start with creating a Windows Form Application in C# for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application and name your project validate url.
2. Next, add only one TextBox named Textbox1 for inputting a desired URL. Add also one button named Button1 to know if the inputted URL is valid or not. You must design your layout like this:
3. Import a namespace named System.Text.RegularExpressions because we will use regular expression function to commnunicate with our input. We have imported System.Text.RegularExpressions because we will use a regular expression to match the pattern of the URL and the inputted URL in the textbox using IsMatch method.
4. Now put add this code for your code module. The Button1_click here will trigger to decide if the inputted URL in the textbox is valid or not.
We will have to initialize pattern as String to hold a string value of http(s)?://([\w+?\.\w+])+([a-zA-Z0-9\~\!\@\#\$\%\^\&\*\(\)_\-\=\+\\\/\?\.\:\;\'\,]*)?. because this regular expression is the pattern for validating URL.
If the pattern will match the inputted URL in the textbox then it will display Valid URL!. Otherwise, it will prompt an Invalid URL!. Note: It will be a valid URL if it has htpp at the start not www.
Full source code:
Output:
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
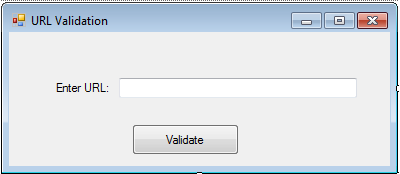
- using System.Diagnostics;
- using System;
- using System.Windows.Forms;
- using System.Collections;
- using System.Drawing;
- using System.Data;
- using System.Collections.Generic;
- using System.Text.RegularExpressions;
- string pattern = default(string);
- pattern = "http(s)?://([\\w+?\\.\\w+])+([a-zA-Z0-9\\~\\!\\@\\#\\$\\%\\^\\&\\*\\(\\)_\\-\\=\\+\\\\\\/\\?\\.\\:\\;\\\'\\,]*)?";
- if (Regex.IsMatch(TextBox1.Text, pattern))
- {
- MessageBox.Show("Valid URL!");
- }
- else
- {
- MessageBox.Show("Invalid URL!");
- }
- using System.Diagnostics;
- using System;
- using System.Windows.Forms;
- using System.Collections;
- using System.Drawing;
- using Microsoft.VisualBasic;
- using System.Data;
- using System.Collections.Generic;
- using System.Text.RegularExpressions;
- namespace validate_url
- {
- public partial class Form1
- {
- public Form1()
- {
- InitializeComponent();
- }
- public void Button1_Click(System.Object sender, System.EventArgs e)
- {
- string pattern = default(string);
- pattern = "http(s)?://([\\w+?\\.\\w+])+([a-zA-Z0-9\\~\\!\\@\\#\\$\\%\\^\\&\\*\\(\\)_\\-\\=\\+\\\\\\/\\?\\.\\:\\;\\\'\\,]*)?";
- if (Regex.IsMatch(TextBox1.Text, pattern))
- {
- MessageBox.Show("Valid URL!");
- }
- else
- {
- MessageBox.Show("Invalid URL!");
- }
- }
- }
- }
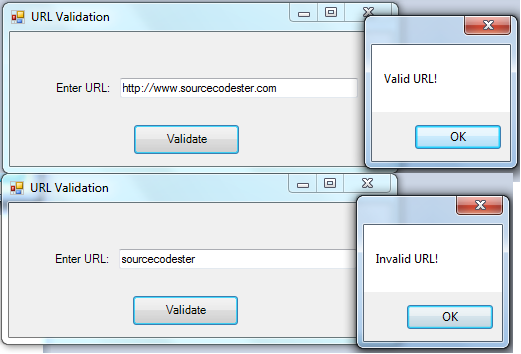