How to Read Data from a Text File using C#
Submitted by donbermoy on Monday, May 26, 2014 - 09:15.
Today, I will teach you to create a program that can read the data from a text file using C#.
Now, let's start this tutorial!
1. Open Notepad. Put any data in there, for example, i have write "Sourcecodester is the best!". Save it to the C directory with folder named file and named it as data.txt.
2. Create a Windows Form Application in C# for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application.
3. Next, add only one Button named btnRead and labeled it as "Read". Insert two textboxes named txtInput for inputting the source file and path of the text file that we wanted to read, and txtOutput to display the content of our text file. You must design your interface like this:
4. In your btnRead, put this code below.
Create a string variable named pathfile that will hold the text inputted in txtInput.
Initialize the System.IO.StreamReader having a file variable. This will locate the file and path of our inputted source.
Code for reading the file.
Close your file.
Display the content of our file and will be viewed in txtOutput.
Full source code:
Press "F5" to run the program.
Output:
Hope this helps! :)
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
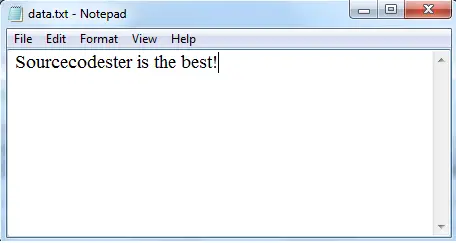
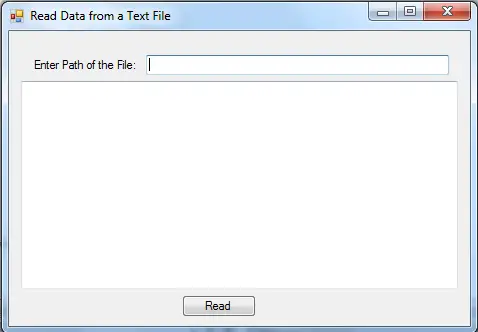
- string pathfile = txtInput.Text;
- string data = File.ReadToEnd();
- File.Close();
- txtOutput.Text = data;
- using System;
- using System.Collections.Generic;
- using System.ComponentModel;
- using System.Data;
- using System.Drawing;
- using System.Text;
- using System.Windows.Forms;
- namespace Read_Data_from_TextFile_CSharp
- {
- public partial class Form1 : Form
- {
- public Form1()
- {
- InitializeComponent();
- }
- private void btnRead_Click(object sender, EventArgs e)
- {
- // change the path of your file
- // string pathfile = @"C:/files/data.txt";
- string pathfile = txtInput.Text;
- string data = File.ReadToEnd();
- File.Close();
- txtOutput.Text = data;
- }
- }
- }
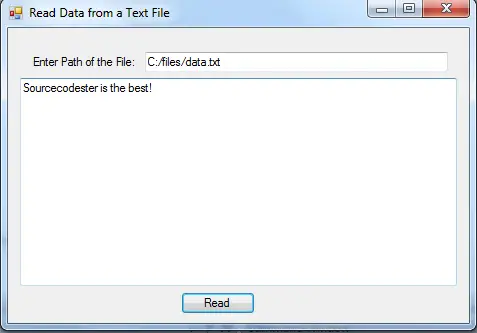